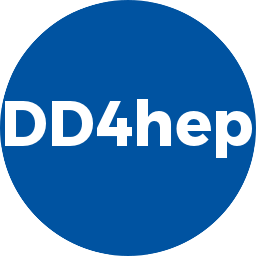 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DD4HEP_SHAPES_H
15 #define DD4HEP_SHAPES_H
27 #pragma GCC diagnostic push
28 #pragma GCC diagnostic ignored "-Wdeprecated" // Code that causes warning goes here
42 #include <TGeoTorus.h>
43 #include <TGeoSphere.h>
44 #include <TGeoHalfSpace.h>
45 #include <TGeoParaboloid.h>
46 #include <TGeoScaledShape.h>
47 #include <TGeoCompositeShape.h>
48 #include <TGeoShapeAssembly.h>
50 #include <TGeoTessellated.h>
53 #pragma GCC diagnostic pop
62 std::string
toStringSolid(
const TGeoShape* shape,
int precision=2);
66 std::string
toStringMesh(
const TGeoShape* shape,
int precision=2);
78 template <
typename SOLID>
bool isInstance(
const Handle<TGeoShape>& solid);
80 template <
typename SOLID>
bool isA(
const Handle<TGeoShape>& solid);
82 template <
typename SOLID> std::vector<double>
dimensions(
const Handle<TGeoShape>& solid);
84 template <
typename SOLID>
void set_dimensions(SOLID solid,
const std::vector<double>& params);
87 inline std::vector<double>
_make_vector(
const double* values,
size_t length) {
88 return {values, values+length};
91 double (SOLID::*extract)(Int_t)
const,
92 Int_t (SOLID::*len)()
const) {
93 std::vector<double> result;
94 Int_t count = (solid->*len)();
95 for(Int_t i=0; i<count; ++i) result.emplace_back((solid->*extract)(i));
98 template <
typename SOLID> std::vector<double>
zPlaneZ(
const SOLID* solid) {
99 const auto* shape = solid->access();
102 template <
typename SOLID> std::vector<double>
zPlaneRmin(
const SOLID* solid) {
103 const auto* shape = solid->access();
106 template <
typename SOLID> std::vector<double>
zPlaneRmax(
const SOLID* solid) {
107 const auto* shape = solid->access();
143 void _assign(T* n,
const std::string& nam,
const std::string& tit,
bool cbbox);
167 const char*
name()
const;
174 const char*
title()
const;
177 const char*
type()
const;
179 operator T*()
const {
195 TGeoVolume*
divide(
const Volume& voldiv,
const std::string& divname,
196 int iaxis,
int ndiv,
double start,
double step)
const;
243 void make(
const std::string&
name,
Solid base_solid,
double x_scale,
double y_scale,
double z_scale);
258 Scale(
Solid base_solid,
double x_scale,
double y_scale,
double z_scale)
259 {
make(
"", base_solid, x_scale, y_scale, z_scale); }
261 Scale(
const std::string& nam,
Solid base_solid,
double x_scale,
double y_scale,
double z_scale)
262 {
make(nam.c_str(), base_solid, x_scale, y_scale, z_scale); }
265 template <
typename X,
typename Y,
typename Z>
266 Scale(
Solid base_solid,
const X& x_scale,
const Y& y_scale,
const Z& z_scale)
269 template <
typename X,
typename Y,
typename Z>
270 Scale(
const std::string& nam,
Solid base_solid,
const X& x_scale,
const Y& y_scale,
const Z& z_scale)
298 void make(
const std::string&
name,
double x_val,
double y_val,
double z_val);
313 Box(
double x_val,
double y_val,
double z_val)
314 {
make(
"", x_val, y_val, z_val); }
316 Box(
const std::string& nam,
double x_val,
double y_val,
double z_val)
317 {
make(nam.c_str(), x_val, y_val, z_val); }
320 template <
typename X,
typename Y,
typename Z>
321 Box(
const X& x_val,
const Y& y_val,
const Z& z_val)
324 template <
typename X,
typename Y,
typename Z>
325 Box(
const std::string& nam,
const X& x_val,
const Y& y_val,
const Z& z_val)
355 void make(
const std::string&
name,
const double*
const point,
const double*
const normal);
374 HalfSpace(
const std::string& nam,
const double*
const point,
const double*
const normal)
384 const double* pos =
access()->GetPoint();
385 return {pos[0], pos[1], pos[2]};
389 const double* n =
access()->GetNorm();
390 return {n[0], n[1], n[2]};
406 void make(
const std::string&
name,
double z,
double rmin1,
double rmax1,
double rmin2,
double rmax2);
420 Cone(
double z,
double rmin1,
double rmax1,
double rmin2,
double rmax2)
421 { this->
make(
"", z, rmin1, rmax1, rmin2, rmax2); }
423 template <
typename Z,
typename RMIN1,
typename RMAX1,
typename RMIN2,
typename RMAX2>
424 Cone(
const Z& z,
const RMIN1& rmin1,
const RMAX1& rmax1,
const RMIN2& rmin2,
const RMAX2& rmax2)
428 Cone(
const std::string& nam,
double z,
double rmin1,
double rmax1,
double rmin2,
double rmax2)
429 { this->
make(nam, z, rmin1, rmax1, rmin2, rmax2); }
431 template <
typename Z,
typename RMIN1,
typename RMAX1,
typename RMIN2,
typename RMAX2>
432 Cone(
const std::string& nam,
const Z& z,
const RMIN1& rmin1,
const RMAX1& rmax1,
const RMIN2& rmin2,
const RMAX2& rmax2)
440 Cone&
setDimensions(
double z,
double rmin1,
double rmax1,
double rmin2,
double rmax2);
489 const std::vector<double>& r,
const std::vector<double>&
z);
492 const std::vector<double>& rmin,
const std::vector<double>& rmax,
const std::vector<double>&
z);
498 const std::vector<double>& r,
const std::vector<double>&
z);
501 const std::vector<double>& rmin,
const std::vector<double>& rmax,
const std::vector<double>&
z);
509 void addZPlanes(
const std::vector<double>& rmin,
const std::vector<double>& rmax,
const std::vector<double>&
z);
517 double z(
int which)
const {
return access()->GetZ(which); }
519 double rMin(
int which)
const {
return access()->GetRmin(which); }
521 double rMax(
int which)
const {
return access()->GetRmax(which); }
546 double rmin1,
double rmax1,
547 double rmin2,
double rmax2,
566 template <
typename DZ,
567 typename RMIN1,
typename RMAX1,
568 typename RMIN2,
typename RMAX2,
569 typename STARTPHI,
typename ENDPHI>
570 ConeSegment(DZ dz, RMIN1 rmin1, RMAX1 rmax1, RMIN2 rmin2, RMAX2 rmax2,
577 ConeSegment(
const std::string& nam,
double dz,
double rmin1,
double rmax1,
581 template <
typename DZ,
582 typename RMIN1,
typename RMAX1,
583 typename RMIN2,
typename RMAX2,
584 typename STARTPHI,
typename ENDPHI>
585 ConeSegment(
const std::string& nam, DZ dz, RMIN1 rmin1, RMAX1 rmax1, RMIN2 rmin2, RMAX2 rmax2,
598 double rmin2,
double rmax2,
632 void make(
const std::string& nam,
double rmin,
double rmax,
double z,
double startPhi,
double endPhi);
647 Tube(
double rmin,
double rmax,
double dz)
648 { this->
make(
"", rmin, rmax, dz, 0, 2*
M_PI); }
650 template <
typename RMIN,
typename RMAX,
typename DZ>
Tube(RMIN rmin, RMAX rmax, DZ dz)
654 { this->
make(
"", rmin, rmax, dz, 0,
endPhi); }
656 template <
typename RMIN,
typename RMAX,
typename DZ,
typename ENDPHI>
663 template <
typename RMIN,
typename RMAX,
typename DZ,
typename STARTPHI,
typename ENDPHI>
668 Tube(
const std::string& nam,
double rmin,
double rmax,
double dz)
669 { this->
make(nam, rmin, rmax, dz, 0, 2*
M_PI); }
671 template <
typename RMIN,
typename RMAX,
typename DZ>
672 Tube(
const std::string& nam, RMIN rmin, RMAX rmax, DZ dz)
675 Tube(
const std::string& nam,
double rmin,
double rmax,
double dz,
double endPhi)
676 { this->
make(nam, rmin, rmax, dz, 0,
endPhi); }
678 template <
typename RMIN,
typename RMAX,
typename DZ,
typename ENDPHI>
679 Tube(
const std::string& nam, RMIN rmin, RMAX rmax, DZ dz, ENDPHI
endPhi)
685 template <
typename RMIN,
typename RMAX,
typename DZ,
typename STARTPHI,
typename ENDPHI>
721 double rmin,
double rmax,
double dz,
double startPhi,
double endPhi,
722 double lx,
double ly,
double lz,
double tx,
double ty,
double tz);
738 double lx,
double ly,
double lz,
double tx,
double ty,
double tz);
742 double rmin,
double rmax,
double dz,
double startPhi,
double endPhi,
743 double lx,
double ly,
double lz,
double tx,
double ty,
double tz);
845 void make(
const std::string& nam,
double a,
double b,
double dz);
862 template <
typename A,
typename B,
typename DZ>
868 { this->
make(nam,
a,
b, dz); }
870 template <
typename A,
typename B,
typename DZ>
904 void make(
const std::string& nam,
double twist_angle,
double rmin,
double rmax,
905 double zneg,
double zpos,
int nsegments,
double totphi);
921 double dz,
double dphi)
922 { this->
make(
"", twist_angle, rmin, rmax, -dz, dz, 1, dphi); }
925 double dz,
int nsegments,
double totphi)
926 { this->
make(
"", twist_angle, rmin, rmax, -dz, dz, nsegments, totphi); }
929 double zneg,
double zpos,
double totphi)
930 { this->
make(
"", twist_angle, rmin, rmax, zneg, zpos, 1, totphi); }
933 double zneg,
double zpos,
int nsegments,
double totphi)
934 { this->
make(
"", twist_angle, rmin, rmax, zneg, zpos, nsegments, totphi); }
937 TwistedTube(
const std::string& nam,
double twist_angle,
double rmin,
double rmax,
938 double dz,
double dphi)
939 { this->
make(nam, twist_angle, rmin, rmax, -dz, dz, 1, dphi); }
941 TwistedTube(
const std::string& nam,
double twist_angle,
double rmin,
double rmax,
942 double dz,
int nsegments,
double totphi)
943 { this->
make(nam, twist_angle, rmin, rmax, -dz, dz, nsegments, totphi); }
945 TwistedTube(
const std::string& nam,
double twist_angle,
double rmin,
double rmax,
946 double zneg,
double zpos,
double totphi)
947 { this->
make(nam, twist_angle, rmin, rmax, zneg, zpos, 1, totphi); }
949 TwistedTube(
const std::string& nam,
double twist_angle,
double rmin,
double rmax,
950 double zneg,
double zpos,
int nsegments,
double totphi)
951 { this->
make(nam, twist_angle, rmin, rmax, zneg, zpos, nsegments, totphi); }
954 template <
typename A,
typename B,
typename DZ>
955 TwistedTube(
const std::string& nam,
const A& a,
const B& b,
const DZ& dz)
978 void make(
const std::string&
name,
double pz,
double py,
double px,
double pLTX);
993 double h1,
double bl1,
double tl1,
double alpha1,
994 double h2,
double bl2,
double tl2,
double alpha2);
996 Trap(
double pz,
double py,
double px,
double pLTX)
997 { this->
make(
"", pz,py,px,pLTX); }
999 template <
typename PZ,
typename PY,
typename PX,
typename PLTX>
Trap(PZ pz, PY py, PX px, PLTX pLTX)
1004 double z,
double theta,
double phi,
1005 double h1,
double bl1,
double tl1,
double alpha1,
1006 double h2,
double bl2,
double tl2,
double alpha2);
1008 Trap(
const std::string& nam,
double pz,
double py,
double px,
double pLTX)
1009 { this->
make(nam, pz,py,px,pLTX); }
1011 template <
typename PZ,
typename PY,
typename PX,
typename PLTX>
1012 Trap(
const std::string& nam, PZ pz, PY py, PX px, PLTX pLTX)
1021 double h1,
double bl1,
double tl1,
double alpha1,
1022 double h2,
double bl2,
double tl2,
double alpha2);
1025 double phi()
const {
return access()->GetPhi()*dd4hep::deg; }
1064 void make(
const std::string& nam,
double x1,
double x2,
double y1,
double y2,
double z,
double radius,
bool minusZ);
1078 PseudoTrap(
double x1,
double x2,
double y1,
double y2,
double z,
double radius,
bool minusZ)
1079 { this->
make(
"", x1, x2, y1, y2, z, radius, minusZ); }
1083 double x1,
double x2,
1084 double y1,
double y2,
1085 double z,
double radius,
bool minusZ)
1086 { this->
make(nam, x1, x2, y1, y2, z, radius, minusZ); }
1107 void make(
const std::string& nam,
double x1,
double x2,
double y,
double z);
1122 Trd1(
double x1,
double x2,
double y,
double z)
1123 { this->
make(
"", x1, x2, y, z); }
1125 template <
typename X1,
typename X2,
typename Y,
typename Z>
1130 Trd1(
const std::string& nam,
double x1,
double x2,
double y,
double z)
1131 { this->
make(nam, x1, x2, y, z); }
1133 template <
typename X1,
typename X2,
typename Y,
typename Z>
1134 Trd1(
const std::string& nam, X1 x1, X2 x2, Y y, Z z)
1167 void make(
const std::string& nam,
double x1,
double x2,
double y1,
double y2,
double z);
1182 Trd2(
double x1,
double x2,
double y1,
double y2,
double z)
1183 { this->
make(
"", x1, x2, y1, y2, z); }
1185 template <
typename X1,
typename X2,
typename Y1,
typename Y2,
typename Z>
1186 Trd2(X1 x1, X2 x2, Y1 y1, Y2 y2, Z z)
1190 Trd2(
const std::string& nam,
double x1,
double x2,
double y1,
double y2,
double z)
1191 { this->
make(nam, x1, x2, y1, y2, z); }
1193 template <
typename X1,
typename X2,
typename Y1,
typename Y2,
typename Z>
1194 Trd2(
const std::string& nam, X1 x1, X2 x2, Y1 y1, Y2 y2, Z z)
1230 void make(
const std::string& nam,
double r,
double rmin,
double rmax,
double startPhi,
double deltaPhi);
1244 template<
typename R,
typename RMIN,
typename RMAX,
typename STARTPHI,
typename DELTAPHI>
1252 template<
typename R,
typename RMIN,
typename RMAX,
typename STARTPHI,
typename DELTAPHI>
1292 void make(
const std::string& nam,
1293 double rmin,
double rmax,
1314 template<
typename RMIN,
typename RMAX,
1315 typename STARTTHETA=double,
typename ENDTHETA=double,
1316 typename STARTPHI=double,
typename ENDPHI=
double>
1328 double rmin,
double rmax,
1333 template<
typename RMIN,
typename RMAX,
1334 typename STARTTHETA=double,
typename ENDTHETA=double,
1335 typename STARTPHI=double,
typename ENDPHI=
double>
1337 RMIN rmin, RMAX rmax,
1381 void make(
const std::string& nam,
double r_low,
double r_high,
double delta_z);
1396 { this->
make(
"", r_low, r_high, delta_z); }
1398 template<
typename R_LOW,
typename R_HIGH,
typename DELTA_Z>
1403 Paraboloid(
const std::string& nam,
double r_low,
double r_high,
double delta_z)
1404 { this->
make(nam, r_low, r_high, delta_z); }
1406 template<
typename R_LOW,
typename R_HIGH,
typename DELTA_Z>
1407 Paraboloid(
const std::string& nam, R_LOW r_low, R_HIGH r_high, DELTA_Z delta_z)
1437 void make(
const std::string& nam,
double rin,
double stin,
double rout,
double stout,
double dz);
1451 Hyperboloid(
double rin,
double stin,
double rout,
double stout,
double dz)
1452 {
make(
"", rin, stin, rout, stout, dz); }
1454 template <
typename RIN,
typename STIN,
typename ROUT,
typename STOUT,
typename DZ>
1459 Hyperboloid(
const std::string& nam,
double rin,
double stin,
double rout,
double stout,
double dz)
1460 {
make(nam, rin, stin, rout, stout, dz); }
1462 template <
typename RIN,
typename STIN,
typename ROUT,
typename STOUT,
typename DZ>
1463 Hyperboloid(
const std::string& nam, RIN rin, STIN stin, ROUT rout, STOUT stout, DZ dz)
1497 void make(
const std::string& nam,
int nsides,
double rmin,
double rmax,
double zpos,
double zneg,
double start,
double delta);
1512 { this->
make(
"", nsides, rmin, rmax, zlen / 2, -zlen / 2, 0, 2.0*
M_PI); }
1514 template <
typename NSIDES,
typename RMIN,
typename RMAX,
typename ZLEN>
1524 { this->
make(
"", nsides, rmin, rmax, zlen / 2, -zlen / 2, phi_start, 2.0*
M_PI); }
1526 template <
typename NSIDES,
typename PHI_START,
typename RMIN,
typename RMAX,
typename ZLEN>
1536 { this->
make(
"", nsides, rmin, rmax, zplanes[0], zplanes[1], 0, 2.0*
M_PI); }
1540 { this->
make(nam, nsides, rmin, rmax, zlen / 2, -zlen / 2, 0, 2.0*
M_PI); }
1542 PolyhedraRegular(
const std::string& nam,
int nsides,
double phi_start,
double rmin,
double rmax,
double zlen)
1543 { this->
make(nam, nsides, rmin, rmax, zlen / 2, -zlen / 2, phi_start, 2.0*
M_PI); }
1545 PolyhedraRegular(
const std::string& nam,
int nsides,
double rmin,
double rmax,
double zplanes[2])
1546 { this->
make(nam, nsides, rmin, rmax, zplanes[0], zplanes[1], 0, 2.0*
M_PI); }
1560 double z(
int which)
const {
return access()->GetZ(which); }
1562 double rMin(
int which)
const {
return access()->GetRmin(which); }
1564 double rMax(
int which)
const {
return access()->GetRmax(which); }
1586 void make(
const std::string& nam,
int nsides,
double start,
double delta,
1587 const std::vector<double>&
z,
1588 const std::vector<double>& rmin,
1589 const std::vector<double>& rmax);
1604 const std::vector<double>&
z,
const std::vector<double>& r) {
1605 std::vector<double> rmin(r.size(), 0e0);
1606 this->
make(
"", nsides, start,
delta,
z, rmin, r);
1610 const std::vector<double>&
z,
const std::vector<double>& rmin,
const std::vector<double>& rmax)
1611 { this->
make(
"", nsides, start,
delta,
z, rmin, rmax); }
1615 const std::vector<double>&
z,
const std::vector<double>& r) {
1616 std::vector<double> rmin(r.size(), 0e0);
1617 this->
make(nam, nsides, start,
delta,
z, rmin, r);
1621 const std::vector<double>&
z,
const std::vector<double>& rmin,
const std::vector<double>& rmax)
1622 { this->
make(nam, nsides, start,
delta,
z, rmin, rmax); }
1636 double z(
int which)
const {
return access()->GetZ(which); }
1638 double rMin(
int which)
const {
return access()->GetRmin(which); }
1640 double rMax(
int which)
const {
return access()->GetRmax(which); }
1662 void make(
const std::string& nam,
1663 const std::vector<double> & pt_x,
1664 const std::vector<double> & pt_y,
1665 const std::vector<double> & sec_z,
1666 const std::vector<double> & sec_x,
1667 const std::vector<double> & sec_y,
1668 const std::vector<double> &
zscale);
1683 const std::vector<double> & pt_y,
1684 const std::vector<double> & sec_z,
1685 const std::vector<double> & sec_x,
1686 const std::vector<double> & sec_y,
1687 const std::vector<double> &
zscale)
1688 { this->
make(
"", pt_x, pt_y, sec_z, sec_x, sec_y,
zscale); }
1692 const std::vector<double> & pt_x,
1693 const std::vector<double> & pt_y,
1694 const std::vector<double> & sec_z,
1695 const std::vector<double> & sec_x,
1696 const std::vector<double> & sec_y,
1697 const std::vector<double> &
zscale)
1698 { this->
make(nam, pt_x, pt_y, sec_z, sec_x, sec_y,
zscale); }
1724 void make(
const std::string& nam,
double dz,
const double* vtx);
1754 const double* values =
access()->GetVertices();
1758 std::pair<double, double>
vertex(
int which)
const {
1759 const double* values =
access()->GetVertices();
1760 return std::make_pair(values[2*which], values[2*which+1]);
1776 void make(
const std::string& nam,
int num_facets);
1778 void make(
const std::string& nam,
const std::vector<Object::Vertex_t>& vertices);
1798 { this->
make(
"", num_facets); }
1802 { this->
make(
"", vertices); }
1806 { this->
make(nam, num_facets); }
1810 { this->
make(nam, vertices); }
1821 bool addFacet(
const int pt0,
const int pt1,
const int pt2)
const;
1823 bool addFacet(
const int pt0,
const int pt1,
const int pt2,
const int pt3)
const;
1853 template <
typename Q>
2013 #endif // DD4HEP_SHAPES_H
Cone(const std::string &nam, double z, double rmin1, double rmax1, double rmin2, double rmax2)
Constructor to create a new anonymous object with attribute initialization.
Polycone(const Polycone &e)=default
Copy constructor.
void _assign(T *n, const std::string &nam, const std::string &tit, bool cbbox)
Assign pointrs and register solid to geometry.
PolyhedraRegular(int nsides, double rmin, double rmax, double zlen)
Constructor to create a new object. Phi(start)=0, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
double high1() const
Half length in y at low z.
Scale(const std::string &nam, Solid base_solid, const X &x_scale, const Y &y_scale, const Z &z_scale)
Constructor to create a named new Scale object (retrieves name from volume)
Box(const std::string &nam, double x_val, double y_val, double z_val)
Constructor to create a named new box object (retrieves name from volume)
Solid_type & operator=(const Solid_type ©)=default
Assignment copy operator.
double endPhi() const
Accessor: end-phi value.
Torus & setDimensions(double r, double rmin, double rmax, double startPhi, double deltaPhi)
Set the Torus dimensions.
double rMax() const
Accessor: r-max value.
HalfSpace(const double *const point, const double *const normal)
Constructor to create an new halfspace object from a point on a plane and the plane normal.
UnionSolid()=default
Default constructor.
IntersectionSolid(IntersectionSolid &&e)=default
Move Constructor.
bool cutInside() const
Accessor: cut-inside value.
Trd2()=default
Default constructor.
IntersectionSolid & operator=(const IntersectionSolid ©)=default
Copy Assignment operator.
Direction normal() const
Accessor: normal vector spanning plane at positioning point.
void make(const std::string &nam, double r_low, double r_high, double delta_z)
Constructor function to create a new anonymous object with attribute initialization.
PolyhedraRegular(const std::string &nam, int nsides, double phi_start, double rmin, double rmax, double zlen)
Constructor to create a new object with phi_start, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
Hyperboloid & operator=(const Hyperboloid ©)=default
Copy Assignment operator.
Polycone(Polycone &&e)=default
Move constructor.
double startPhi() const
Accessor: start-phi value.
Cone(const Z &z, const RMIN1 &rmin1, const RMAX1 &rmax1, const RMIN2 &rmin2, const RMAX2 &rmax2)
Constructor to create a new anonymous object with attribute initialization.
T * operator->() const
Overloaded operator -> to access underlying object.
Cone(const Handle< Q > &e)
Constructor to be used when passing an already created object.
TwistedTube & operator=(TwistedTube &©)=default
Move Assignment operator.
Scale(const Handle< Q > &e)
Copy Constructor to be used with an existing object handle.
double rMin() const
Accessor: r-min value.
void make(const std::string &nam, double x1, double x2, double y1, double y2, double z)
Internal helper method to support object construction.
Scale(Solid base_solid, double x_scale, double y_scale, double z_scale)
Constructor to create an anonymous new Scale object (retrieves name from volume)
TessellatedSolid & operator=(TessellatedSolid &©)=default
Move Assignment operator.
double deltaPhi() const
Accessor: delta-phi value.
double dZ() const
Half length in dZ.
Class describing a truncated tube shape (CMS'ism)
PolyhedraRegular(const Q *p)
Constructor to be used with an existing object.
const char * title() const
Access to shape title (GetTitle accessor of the TGeoShape)
void make(const std::string &nam, double x1, double x2, double y1, double y2, double z, double radius, bool minusZ)
Internal helper method to support object construction.
UnionSolid(const UnionSolid &e)=default
Copy Constructor.
Sphere(Sphere &&e)=default
Move Constructor.
ExtrudedPolygon & operator=(ExtrudedPolygon &©)=default
Move Assignment operator.
Scale(Solid base_solid, const X &x_scale, const Y &y_scale, const Z &z_scale)
Constructor to create an anonymous new Scale object (retrieves name from volume)
UnionSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
double rMin() const
Accessor: r-min value.
Cone(const Q *p)
Constructor to be used with an existing object.
Trap & setDimensions(double z, double theta, double phi, double h1, double bl1, double tl1, double alpha1, double h2, double bl2, double tl2, double alpha2)
Set the trap dimensions.
void make(const std::string &name, double pz, double py, double px, double pLTX)
Internal helper method to support object construction.
Trap(double pz, double py, double px, double pLTX)
Constructor to create a new anonymous object for right angular wedge from STEP (Se G4 manual for deta...
PolyhedraRegular(int nsides, double rmin, double rmax, double zplanes[2])
Constructor to create a new object. Phi(start)=0, deltaPhi=2PI, Z-planes a zplanes[0] and zplanes[1].
int num_vertex() const
Access the number of vertices in the shape.
TruncatedTube()=default
Default constructor.
double dZ() const
Accessor: delta-z value.
double endPhi() const
Accessor: end-phi value.
double high2() const
Half length in y at high z.
Class describing a regular polyhedron shape.
double rMax(int which) const
Accessor: r-max value.
PolyhedraRegular(const std::string &nam, int nsides, double rmin, double rmax, double zlen)
Constructor to create a new object. Phi(start)=0, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
std::vector< double > zPlaneRmax() const
Accessor: vector of rMax-values for Z-planes value.
Class describing a pseudo trap shape (CMS'ism)
TGeoHalfSpace Object
Extern accessible definition of the contained element type.
TwistedTube(double twist_angle, double rmin, double rmax, double dz, int nsegments, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
Solid leftShape() const
Access left solid of the boolean.
Class describing a Torus shape.
Class describing half-space.
Cone()=default
Default constructor.
double rMin() const
Accessor: r-min value.
Sphere(const Q *p)
Constructor to be used with an existing object.
Tube(Tube &&e)=default
Move Constructor.
Solid_type(const Solid_type &e)=default
Copy constructor.
Class describing a shape-less solid shape.
PolyhedraRegular(const std::string &nam, int nsides, double rmin, double rmax, double zplanes[2])
Constructor to create a new object. Phi(start)=0, deltaPhi=2PI, Z-planes a zplanes[0] and zplanes[1].
UnionSolid & operator=(const UnionSolid ©)=default
Copy Assignment operator.
Hyperboloid(RIN rin, STIN stin, ROUT rout, STOUT stout, DZ dz)
Constructor to create a new anonymous object with attribute initialization.
double rMax(int which) const
Accessor: r-max value.
Tube(double rmin, double rmax, double dz, double startPhi, double endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
Class describing a twisted tube shape.
double x() const
Access half "length" of the box.
Trap & operator=(Trap &©)=default
Move Assignment operator.
EllipticalTube(double a, double b, double dz)
Constructor to create a new anonymous tube object with attribute initialization.
std::vector< double > dimensions(const Handle< TGeoShape > &solid)
Access Shape dimension parameters (As in TGeo, but angles in radians rather than degrees)
Trd2 & operator=(Trd2 &©)=default
Copy Assignment operator.
ConeSegment(const Q *p)
Constructor to be used with an existing object.
Polyhedra & operator=(const Polyhedra ©)=default
Copy Assignment operator.
Scale(Scale &&e)=default
Move constructor.
double rMin(int which) const
Accessor: r-min value.
double rMax() const
Accessor: r-max value (outer radius)
Polyhedra(const Polyhedra &e)=default
Copy Constructor.
std::pair< double, double > vertex(int which) const
Accessor: single vertex.
PseudoTrap(double x1, double x2, double y1, double y2, double z, double radius, bool minusZ)
Constructor to create a new anonymous object with attribute initialization.
std::vector< double > vertices() const
Accessor: all vertices as STL vector.
Paraboloid & operator=(Paraboloid &©)=default
Move Assignment operator.
Torus()=default
Default constructor.
Cone(const Cone &e)=default
Copy Constructor.
std::vector< double > highNormal() const
Accessor: upper normal vector of cut plane.
Sphere()=default
Default constructor.
PolyhedraRegular(const Handle< Q > &e)
Constructor to be used when passing an already created object.
double startTheta() const
Accessor: start-theta value.
Class describing a Hyperboloid shape.
SubtractionSolid & operator=(SubtractionSolid &©)=default
Move Assignment operator.
Trap(PZ pz, PY py, PX px, PLTX pLTX)
Constructor to create a new anonymous object with attribute initialization.
ExtrudedPolygon(ExtrudedPolygon &&e)=default
Move Constructor.
Torus(const Handle< Q > &e)
Constructor to be used when passing an already created object.
double startPhi() const
Accessor: start-phi value.
double dZ() const
Accessor: z-half value.
Tube(double rmin, double rmax, double dz, double endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
bool isA(const Handle< TGeoShape > &solid)
Type check of various shapes. Do not allow for polymorphism. Types must match exactly.
double dX1() const
Accessor: delta-x1 value.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Tube(const std::string &nam, double rmin, double rmax, double dz, double startPhi, double endPhi)
Legacy: Constructor to create a new identifiable tube object with attribute initialization.
Scale & operator=(Scale &©)=default
Move Assignment operator.
double bottomLow2() const
Half length in x at high z and y low edge.
ROOT::Math::Rotation3D Rotation3D
double z(int which) const
Accessor: z value.
Tube & operator=(Tube &©)=default
Move Assignment operator.
Tube(const Q *p)
Constructor to be used with an existing object.
Class describing a trap shape.
Torus(double r, double rmin, double rmax, double startPhi=M_PI, double deltaPhi=2.*M_PI)
Constructor to create a new anonymous object with attribute initialization.
Base class describing boolean (=union,intersection,subtraction) solids.
Base class for Solid (shape) objects.
ConeSegment & setDimensions(double dz, double rmin1, double rmax1, double rmin2, double rmax2, double startPhi=0.0, double endPhi=2.0 *M_PI)
Set the cone segment dimensions.
double rMin2() const
Accessor: r-min-2 value.
TruncatedTube & operator=(const TruncatedTube ©)=default
Copy Assignment operator.
Sphere(const std::string &nam, double rmin, double rmax, double startTheta=0.0, double endTheta=M_PI, double startPhi=0.0, double endPhi=2. *M_PI)
Constructor to create a new identified object with attribute initialization.
Solid_type(const Handle< Q > &e)
Constructor to be used when passing an already created object: need to check pointers.
double rMin2() const
Accessor: r-min-2 value.
Box(const Handle< Q > &e)
Copy Constructor to be used with an existing object handle.
Polyhedra()=default
Default constructor.
CutTube & operator=(CutTube &©)=default
Move Assignment operator.
PolyhedraRegular(const PolyhedraRegular &e)=default
Copy Constructor.
Polyhedra(int nsides, double start, double delta, const std::vector< double > &z, const std::vector< double > &r)
Constructor to create a new object. Phi(start), deltaPhi, Z-planes at specified positions.
Hyperboloid(const Hyperboloid &e)=default
Copy Constructor.
EightPointSolid(EightPointSolid &&e)=default
Move Constructor.
Torus(const std::string &nam, double r, double rmin, double rmax, double startPhi=M_PI, double deltaPhi=2.*M_PI)
Constructor to create a new identified object with attribute initialization.
SubtractionSolid()=default
Default constructor.
BooleanSolid(BooleanSolid &&b)=default
Move Constructor.
double dY1() const
Accessor: delta-y1 value.
Hyperboloid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
TGeoVolume * divide(const Volume &voldiv, const std::string &divname, int iaxis, int ndiv, double start, double step) const
Divide volume into subsections (See the ROOT manuloa for details)
std::vector< double > z() const
Paraboloid(const Q *p)
Constructor to be used with an existing object.
void make(const std::string &name, const double *const point, const double *const normal)
Internal helper method to support object construction.
Trd1(const Q *p)
Constructor to be used with an existing object.
std::vector< double > zPlaneRmin() const
Accessor: vector of rMin-values for Z-planes value.
int numEdges() const
Accessor: Number of edges.
double rMax1() const
Accessor: r-max-1 value.
double rMax() const
Accessor: r-max value.
double z() const
Access half "depth" of the box.
Torus(const std::string &nam, R r, RMIN rmin, RMAX rmax, STARTPHI startPhi=M_PI, DELTAPHI deltaPhi=2.*M_PI)
Constructor to create a new identified object with attribute initialization.
Class describing a regular polyhedron shape.
std::vector< double > zscale() const
double y() const
Access half "width" of the box.
Class describing boolean intersection solid.
double rMin(int which) const
Accessor: r-min value
ConeSegment(const std::string &nam, double dz, double rmin1, double rmax1, double rmin2, double rmax2, double startPhi=0.0, double endPhi=2.0 *M_PI)
Constructor to create a new named ConeSegment object.
IntersectionSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
Paraboloid(R_LOW r_low, R_HIGH r_high, DELTA_Z delta_z)
Constructor to create a new anonymous object with attribute initialization.
Tube()=default
Default constructor.
Scale & operator=(const Scale ©)=default
Copy Assignment operator.
void make(const std::string &name, Solid base_solid, double x_scale, double y_scale, double z_scale)
Internal helper method to support object construction.
Solid_type(const Handle< T > &e)
Copy Constructor from handle.
double deltaPhi() const
Accessor: delta-phi value.
TessellatedSolid(const TessellatedSolid &e)=default
Copy Constructor.
ExtrudedPolygon & operator=(const ExtrudedPolygon ©)=default
Copy Assignment operator.
Class describing a cone segment shape.
double dZ() const
Accessor: delta-z value.
Tube(const Tube &e)=default
Copy Constructor.
Trd2(const Handle< Q > &e)
Constructor to be used when passing an already created object.
ConeSegment(const Handle< Q > &e)
Constructor to be used when reading the already parsed ConeSegment object.
Sphere(const Handle< Q > &e)
Constructor to be used when passing an already created object.
HalfSpace & operator=(const HalfSpace ©)=default
Copy Assignment operator.
CutTube(const Q *p)
Constructor to be used with an existing object.
ExtrudedPolygon()=default
Default constructor.
Torus(const Torus &e)=default
Copy Constructor.
double cutAtStart() const
Accessor: cut at start value.
TruncatedTube(const Q *p)
Constructor to be used with an existing object.
Class describing a Trd2 shape.
std::vector< double > zPlaneZ() const
Accessor: vector of z-values for Z-planes value.
std::string toStringSolid(const TGeoShape *shape, int precision=2)
Pretty print of solid attributes.
PseudoTrap(PseudoTrap &&e)=default
Move Constructor.
double stereoOuter() const
Stereo angle for outer surface.
TwistedTube(const TwistedTube &e)=default
Copy Constructor.
ExtrudedPolygon(const Q *p)
Constructor to be used with an existing object.
TwistedTube(double twist_angle, double rmin, double rmax, double zneg, double zpos, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
void make(const std::string &nam, double x1, double x2, double y, double z)
Internal helper method to support object construction.
EightPointSolid(const EightPointSolid &e)=default
Copy Constructor.
Trap(const std::string &nam, PZ pz, PY py, PX px, PLTX pLTX)
Constructor to create a new identified object with attribute initialization.
TwistedTube(const Q *p)
Constructor to be used with an existing object.
double dX1() const
Accessor: delta-x1 value.
ConeSegment(ConeSegment &&e)=default
Move Constructor.
Position position() const
Accessor: positioning point.
IntersectionSolid()=default
Default constructor.
Cone(double z, double rmin1, double rmax1, double rmin2, double rmax2)
Constructor to create a new anonymous object with attribute initialization.
bool addFacet(const Vertex &pt0, const Vertex &pt1, const Vertex &pt2) const
Add new facet to the shape.
EightPointSolid(const std::string &nam, double dz, const double *vertices)
Constructor to create a new identified object with attribute initialization.
Trd2(X1 x1, X2 x2, Y1 y1, Y2 y2, Z z)
Constructor to create a new anonymous object with attribute initialization.
Trap(const Handle< Q > &e)
Constructor to be used when passing an already created object.
Box(Box &&e)=default
Move constructor.
Trd2 Trapezoid
Shortcut name definition.
Cone & operator=(const Cone ©)=default
Copy Assignment operator.
Trd1(const std::string &nam, double x1, double x2, double y, double z)
Constructor to create a new anonymous object with attribute initialization.
TessellatedSolid(const Q *p)
Constructor to be used with an existing object.
Box & operator=(Box &©)=default
Move Assignment operator.
Paraboloid & setDimensions(double r_low, double r_high, double delta_z)
Set the Paraboloid dimensions.
double endPhi() const
Accessor: end-phi value.
double startPhi() const
Accessor: start-phi value.
BooleanSolid & operator=(const BooleanSolid ©)=default
Copy Assignment operator.
Box(const X &x_val, const Y &y_val, const Z &z_val)
Constructor to create an anonymous new box object (retrieves name from volume)
Trd2(const Trd2 &e)=default
Copy Constructor.
friend void set_dimensions(Q ptr, const std::vector< double > ¶ms)
void make(const std::string &name, double rmin, double rmax, double dz, double startPhi, double endPhi, double lx, double ly, double lz, double tx, double ty, double tz)
Internal helper method to support object construction.
Box(double x_val, double y_val, double z_val)
Constructor to create an anonymous new box object (retrieves name from volume)
ExtrudedPolygon(const std::vector< double > &pt_x, const std::vector< double > &pt_y, const std::vector< double > &sec_z, const std::vector< double > &sec_x, const std::vector< double > &sec_y, const std::vector< double > &zscale)
Constructor to create a new object.
Torus(R r, RMIN rmin, RMAX rmax, STARTPHI startPhi=M_PI, DELTAPHI deltaPhi=2.*M_PI)
Constructor to create a new anonymous object with attribute initialization.
Hyperboloid()=default
Default constructor.
PseudoTrap(const std::string &nam, double x1, double x2, double y1, double y2, double z, double radius, bool minusZ)
Constructor to create a new identified object with attribute initialization.
ShapelessSolid & operator=(ShapelessSolid &©)=default
Move Assignment operator.
Handle class holding a placed volume (also called physical volume)
void make(const std::string &nam, int num_facets)
Internal helper method to support object construction.
Tube & operator=(const Tube ©)=default
Copy Assignment operator.
ConeSegment & operator=(ConeSegment &©)=default
Move Assignment operator.
double dY() const
Accessor: delta-y value.
const char * name() const
Access to shape name.
EllipticalTube()=default
Default constructor.
double startPhi() const
Accessor: start-phi value.
Class describing a Scale shape.
Tube(double rmin, double rmax, double dz)
Constructor to create a new anonymous tube object with attribute initialization.
TessellatedSolid(const std::string &nam, int num_facets)
Constructor to create a new anonymous object with attribute initialization.
std::vector< double > zPlaneZ() const
Accessor: vector of z-values for Z-planes value.
double endTheta() const
Accessor: end-theta value.
TwistedTube(const std::string &nam, double twist_angle, double rmin, double rmax, double zneg, double zpos, int nsegments, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
Cone & setDimensions(double z, double rmin1, double rmax1, double rmin2, double rmax2)
Set the box dimensions.
Trd1(const Handle< Q > &e)
Constructor to be used when passing an already created object.
ShapelessSolid(const ShapelessSolid &e)=default
Copy constructor from handle.
double rMin() const
Accessor: r-min value.
TwistedTube()=default
Default constructor.
Tube(const std::string &nam, RMIN rmin, RMAX rmax, DZ dz)
Constructor to create a new anonymous tube object with attribute initialization.
Sphere(double rmin, double rmax, double startTheta=0.0, double endTheta=M_PI, double startPhi=0.0, double endPhi=2. *M_PI)
Constructor to create a new anonymous object with attribute initialization.
Sphere(const std::string &nam, RMIN rmin, RMAX rmax, STARTTHETA startTheta=0.0, ENDTHETA endTheta=M_PI, STARTPHI startPhi=0.0, ENDPHI endPhi=2. *M_PI)
Constructor to create a new identified object with generic attribute initialization.
ShapelessSolid(const Handle< Q > &e)
Constructor to be used with an existing object.
double theta() const
Accessor: theta value.
double b() const
Accessor: b value (semi axis along y)
TwistedTube(const Handle< Q > &e)
Constructor to assign an object.
double z(int which) const
Accessor: z value.
HalfSpace(HalfSpace &&e)=default
Move Constructor.
Trap & operator=(const Trap ©)=default
Copy Assignment operator.
IntersectionSolid(const IntersectionSolid &e)=default
Copy Constructor.
Hyperboloid(double rin, double stin, double rout, double stout, double dz)
Constructor to create a new anonymous object with attribute initialization.
void make(const std::string &nam, double rin, double stin, double rout, double stout, double dz)
Constructor function to create a new anonymous object with attribute initialization.
CutTube(const Handle< Q > &e)
Constructor to assign an object.
PseudoTrap()=default
Default constructor.
Cone(Cone &&e)=default
Move Constructor.
PolyhedraRegular & operator=(PolyhedraRegular &©)=default
Move Assignment operator.
PolyhedraRegular(NSIDES nsides, RMIN rmin, RMAX rmax, ZLEN zlen)
Constructor to create a new object. Phi(start)=0, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
Polyhedra & operator=(Polyhedra &©)=default
Move Assignment operator.
Tube(RMIN rmin, RMAX rmax, DZ dz)
Constructor to create a new anonymous tube object with attribute initialization.
double topLow1() const
Half length in x at low z and y high edge.
Trd1 & setDimensions(double x1, double x2, double y, double z)
Set the Trd1 dimensions.
ConeSegment(DZ dz, RMIN1 rmin1, RMAX1 rmax1, RMIN2 rmin2, RMAX2 rmax2, STARTPHI startPhi=0.0, ENDPHI endPhi=2.0 *M_PI)
Constructor to create a new ConeSegment object.
Trd1(Trd1 &&e)=default
Move Constructor.
void _setDimensions(double *param) const
Polycone & operator=(Polycone &©)=default
Move Assignment operator.
Paraboloid()=default
Default constructor.
CutTube & operator=(const CutTube ©)=default
Copy Assignment operator.
void make(const std::string &nam, double rmin, double rmax, double startTheta, double endTheta, double startPhi, double endPhi)
Constructor function to be used when creating a new object with attribute initialization.
TessellatedSolid(TessellatedSolid &&e)=default
Move Constructor.
double alpha2() const
Angle between centers of x edges and y axis at low z.
void make(const std::string &nam, double r, double rmin, double rmax, double startPhi, double deltaPhi)
Internal helper method to support object construction.
PolyhedraRegular & operator=(const PolyhedraRegular ©)=default
Copy Assignment operator.
void make(const std::string &nam, double twist_angle, double rmin, double rmax, double zneg, double zpos, int nsegments, double totphi)
Internal helper method to support TwistedTube object construction.
double rMin() const
Accessor: r-min value.
double stereoInner() const
Stereo angle for inner surface.
SubtractionSolid(SubtractionSolid &&e)=default
Move Constructor.
std::vector< double > zPlaneRmin() const
Accessor: vector of rMin-values for Z-planes value.
ConeSegment(const std::string &nam, DZ dz, RMIN1 rmin1, RMAX1 rmax1, RMIN2 rmin2, RMAX2 rmax2, STARTPHI startPhi=0.0, ENDPHI endPhi=2.0 *M_PI)
Constructor to create a new named ConeSegment object.
Polyhedra(int nsides, double start, double delta, const std::vector< double > &z, const std::vector< double > &rmin, const std::vector< double > &rmax)
Constructor to create a new object. Phi(start), deltaPhi, Z-planes at specified positions.
double r() const
Accessor: r value (torus axial radius)
void set_shape_dimensions(TGeoShape *shape, const std::vector< double > ¶ms)
Set the shape dimensions (As for the TGeo shape, but angles in rad rather than degrees)
EightPointSolid(double dz, const double *vertices)
Constructor to create a new anonymous object with attribute initialization.
Sphere & operator=(Sphere &©)=default
Move Assignment operator.
BooleanSolid & operator=(BooleanSolid &©)=default
Move Assignment operator.
double startPhi() const
Accessor: start-phi value.
CutTube(const CutTube &e)=default
Copy Constructor.
Paraboloid(double r_low, double r_high, double delta_z)
Constructor to create a new anonymous object with attribute initialization.
Class describing a cone shape.
Trap(const Trap &e)=default
Copy Constructor.
Polycone()=default
Default constructor.
void addZPlanes(const std::vector< double > &rmin, const std::vector< double > &rmax, const std::vector< double > &z)
Add Z-planes to the Polycone.
Paraboloid & operator=(const Paraboloid ©)=default
Copy Assignment operator.
TruncatedTube(const Handle< Q > &e)
Constructor to assign an object.
Tube(const std::string &nam, RMIN rmin, RMAX rmax, DZ dz, ENDPHI endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
double rMin1() const
Accessor: r-min-1 value.
const Vertex & vertex(int index) const
Access a single vertex from the shape.
Hyperboloid & operator=(Hyperboloid &©)=default
Move Assignment operator.
double scale_y() const
Access y-scale factor.
PseudoTrap & operator=(PseudoTrap &©)=default
Move Assignment operator.
std::vector< double > get_shape_dimensions(TGeoShape *shape)
Access Shape dimension parameters (As in TGeo, but angles in radians rather than degrees)
Trd1 & operator=(const Trd1 ©)=default
Copy Assignment operator.
Solid_type(Handle< T > &&e)
Move Constructor from handle.
T * m_element
Single and only data member: Reference to the actual element.
EightPointSolid & operator=(EightPointSolid &©)=default
Move Assignment operator.
Class describing a extruded polygon shape.
void set_dimensions(SOLID solid, const std::vector< double > ¶ms)
Set the shape dimensions. As for the TGeo shape, but angles in rad rather than degrees.
Class describing a Trd1 shape.
EllipticalTube(const A &a, const B &b, const DZ &dz)
Constructor to create a new anonymous tube object with attribute initialization.
std::vector< double > zPlaneZ(const SOLID *solid)
Trd2 & operator=(const Trd2 ©)=default
Move Assignment operator.
Hyperboloid(const std::string &nam, RIN rin, STIN stin, ROUT rout, STOUT stout, DZ dz)
Constructor to create a new identified object with attribute initialization.
Trd2(Trd2 &&e)=default
Move Constructor.
Trd1(double x1, double x2, double y, double z)
Constructor to create a new anonymous object with attribute initialization.
std::vector< double > zPlaneRmax(const SOLID *solid)
EllipticalTube(const std::string &nam, double a, double b, double dz)
Constructor to create a new identified tube object with attribute initialization.
void make(const std::string &name, double z, double rmin1, double rmax1, double rmin2, double rmax2)
Internal helper method to support object construction.
double deltaPhi() const
Accessor: delta-phi value.
std::vector< double > zx() const
Scale(const std::string &nam, Solid base_solid, double x_scale, double y_scale, double z_scale)
Constructor to create a named new Scale object (retrieves name from volume)
const Facet & facet(int index) const
Access a facet from the built shape.
TwistedTube(const std::string &nam, double twist_angle, double rmin, double rmax, double zneg, double zpos, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
double rMax1() const
Accessor: r-max-1 value.
Solid_type(T *p)
Direct assignment using the implementation pointer.
Sphere & operator=(const Sphere ©)=default
Copy Assignment operator.
HalfSpace(const HalfSpace &e)=default
Copy Constructor.
Torus(const Q *p)
Constructor to be used with an existing object.
Box(const Q *p)
Constructor to be used with an existing object.
Tube(RMIN rmin, RMAX rmax, DZ dz, STARTPHI startPhi, ENDPHI endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
void make(const std::string &nam, int nsides, double rmin, double rmax, double zpos, double zneg, double start, double delta)
Helper function to create the polyhedron.
std::vector< double > y() const
Class describing boolean union solid.
IntersectionSolid & operator=(IntersectionSolid &©)=default
Move Assignment operator.
void make(const std::string &nam, double dz, const double *vtx)
Internal helper method to support object construction.
TwistedTube(const std::string &nam, const A &a, const B &b, const DZ &dz)
Constructor to create a new identified tube object with attribute initialization.
HalfSpace(const std::string &nam, const double *const point, const double *const normal)
Constructor to create an new named halfspace object from a point on a plane and the plane normal.
EllipticalTube & operator=(EllipticalTube &©)=default
Move Assignment operator.
double rMin() const
Accessor: r-min value (inner radius)
Trd2 & setDimensions(double x1, double x2, double y1, double y2, double z)
Set the Trd2 dimensions.
HalfSpace(const Q *p)
Constructor to be used with an existing object.
double rMax(int which) const
Accessor: r-max value.
Trd1(X1 x1, X2 x2, Y y, Z z)
Constructor to create a new anonymous object with attribute initialization.
void make(const std::string &nam, const std::vector< double > &pt_x, const std::vector< double > &pt_y, const std::vector< double > &sec_z, const std::vector< double > &sec_x, const std::vector< double > &sec_y, const std::vector< double > &zscale)
Helper function to create the polygon.
Scale()=default
Default constructor.
double dY2() const
Accessor: delta-y2 value.
double dX2() const
Accessor: delta-x2 value.
Class describing a tube shape of a section of a cut tube segment.
Tube(const std::string &nam, double rmin, double rmax, double dz)
Legacy: Constructor to create a new identifiable tube object with attribute initialization.
double dZ() const
Accessor: delta-z value.
Polycone(const Handle< Q > &e)
Constructor to be used when reading the already parsed polycone object.
ROOT::Math::Transform3D Transform3D
TruncatedTube & operator=(TruncatedTube &©)=default
Move Assignment operator.
Cone(const std::string &nam, const Z &z, const RMIN1 &rmin1, const RMAX1 &rmax1, const RMIN2 &rmin2, const RMAX2 &rmax2)
Constructor to create a new anonymous object with attribute initialization.
Class describing a twisted tube shape.
TruncatedTube(TruncatedTube &&e)=default
Move Constructor.
Class describing an arbitray solid defined by 8 vertices.
HalfSpace()=default
Default constructor.
UnionSolid(UnionSolid &&e)=default
Move Constructor.
std::vector< double > zPlaneRmin(const SOLID *solid)
TessellatedSolid()=default
Default constructor.
Class describing a Polycone shape.
ExtrudedPolygon(const Handle< Q > &e)
Constructor to be used when passing an already created object.
ShapelessSolid(ShapelessSolid &&e)=default
Move constructor from handle.
Hyperboloid(const Q *p)
Constructor to be used with an existing object.
double dZ() const
Accessor: delta-z value.
EllipticalTube(const Handle< Q > &e)
Constructor to assign an object.
CutTube()=default
Default constructor.
TwistedTube(const std::string &nam, double twist_angle, double rmin, double rmax, double dz, double dphi)
Constructor to create a new anonymous tube object with attribute initialization.
double topLow2() const
Half length in x at high z and y high edge.
ROOT::Math::XYZVector Position
TwistedTube & operator=(const TwistedTube ©)=default
Copy Assignment operator.
EightPointSolid(const Q *p)
Constructor to be used with an existing object.
double dZ() const
Accessor: delta-z value.
void make(const std::string &name, double x_val, double y_val, double z_val)
Internal helper method to support object construction.
std::vector< double > zPlaneRmax() const
Accessor: vector of rMax-values for Z-planes value.
Paraboloid(const std::string &nam, R_LOW r_low, R_HIGH r_high, DELTA_Z delta_z)
Constructor to create a new identified object with attribute initialization.
CutTube(CutTube &&e)=default
Move Constructor.
Tube(const std::string &nam, double rmin, double rmax, double dz, double endPhi)
Legacy: Constructor to create a new identifiable tube object with attribute initialization.
double _toDouble(const std::string &value)
String conversions: string to double value.
double startPhi() const
Accessor: start-phi value.
EllipticalTube(const EllipticalTube &e)=default
Copy Constructor.
SubtractionSolid(const SubtractionSolid &e)=default
Copy Constructor.
double rMin(int which) const
Accessor: r-min value.
EllipticalTube(const std::string &nam, const A &a, const B &b, const DZ &dz)
Constructor to create a new identified tube object with attribute initialization.
double dZ() const
Accessor: delta-z value.
Tube(const Handle< Q > &e)
Constructor to assign an object.
Class describing a box shape.
double startPhi() const
Accessor: start-phi value.
double phi() const
Accessor: phi value.
TGeoHalfSpace * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
double scale_x() const
Access x-scale factor.
double deltaPhi() const
Accessor: delta-phi value.
ExtrudedPolygon(const std::string &nam, const std::vector< double > &pt_x, const std::vector< double > &pt_y, const std::vector< double > &sec_z, const std::vector< double > &sec_x, const std::vector< double > &sec_y, const std::vector< double > &zscale)
Constructor to create a new identified object.
Trap(Trap &&e)=default
Move Constructor.
Trd1(const std::string &nam, X1 x1, X2 x2, Y y, Z z)
Constructor to create a new anonymous object with attribute initialization.
PseudoTrap(const Handle< Q > &e)
Constructor to be used when passing an already created object.
Polyhedra(Polyhedra &&e)=default
Move Constructor.
double z(int which) const
Accessor: r-min value.
Box()=default
Default constructor.
Scale(const Q *p)
Constructor to be used with an existing object.
Trd1 & operator=(Trd1 &©)=default
Move Assignment operator.
EightPointSolid()=default
Default constructor.
T * ptr() const
Access to the held object.
double startPhi() const
Accessor: start-phi value.
double rMin1() const
Accessor: r-min-1 value.
Trd2(const Q *p)
Constructor to be used with an existing object.
TruncatedTube(const TruncatedTube &e)=default
Copy Constructor.
void make(const std::string &name, double dz, double rmin1, double rmax1, double rmin2, double rmax2, double startPhi, double endPhi)
Constructor to be used when creating a new cone segment object.
Polyhedra(const std::string &nam, int nsides, double start, double delta, const std::vector< double > &z, const std::vector< double > &rmin, const std::vector< double > &rmax)
Constructor to create a new object. Phi(start), deltaPhi, Z-planes at specified positions.
Tube(const std::string &nam, RMIN rmin, RMAX rmax, DZ dz, STARTPHI startPhi, ENDPHI endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
TessellatedSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
double startPhi() const
Accessor: start-phi value.
Box & setDimensions(double x_val, double y_val, double z_val)
Set the box dimensions.
Polyhedra(const Handle< Q > &e)
Constructor to be used when passing an already created object.
Paraboloid(const Paraboloid &e)=default
Copy Constructor.
EllipticalTube(const Q *p)
Constructor to be used with an existing object.
BooleanSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
std::vector< double > x() const
double cutAtDelta() const
Accessor: cut at delta value.
PseudoTrap & operator=(const PseudoTrap ©)=default
Copy Assignment operator.
Namespace for the AIDA detector description toolkit.
Class describing a sphere shape.
std::string toString(int precision=2) const
Conversion to string for pretty print.
Tube(RMIN rmin, RMAX rmax, DZ dz, ENDPHI endPhi)
Constructor to create a new anonymous tube object with attribute initialization.
Solid_type< TGeoShape > Solid
Polycone & operator=(const Polycone ©)=default
Copy Assignment operator.
Class describing a tessellated shape.
Solid_type & setDimensions(const std::vector< double > ¶ms)
Set the shape dimensions. As for the TGeo shape, but angles in rad rather than degrees.
Sphere(const Sphere &e)=default
Copy Constructor.
PseudoTrap(const PseudoTrap &e)=default
Copy Constructor.
Solid_type()=default
Default constructor for uninitialized object.
const char * type() const
Access to shape type (The TClass name of the ROOT implementation)
TessellatedSolid(int num_facets)
Constructor to create a new anonymous object with attribute initialization.
TessellatedSolid & operator=(const TessellatedSolid ©)=default
Copy Assignment operator.
double a() const
Accessor: a value (semi axis along x)
TwistedTube(double twist_angle, double rmin, double rmax, double zneg, double zpos, int nsegments, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
EightPointSolid & operator=(const EightPointSolid ©)=default
Copy Assignment operator.
PolyhedraRegular(int nsides, double phi_start, double rmin, double rmax, double zlen)
Constructor to create a new object with phi_start, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
Torus & operator=(const Torus ©)=default
Copy Assignment operator.
Paraboloid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
double dZ() const
Accessor: delta-z value.
double bottomLow1() const
Half length in x at low z and y low edge.
Tube & setDimensions(double rmin, double rmax, double dz, double startPhi=0.0, double endPhi=2 *M_PI)
Set the tube dimensions.
UnionSolid & operator=(UnionSolid &©)=default
Move Assignment operator.
double dZ() const
Accessor: delta-z value.
Torus(Torus &&e)=default
Move Constructor.
TessellatedSolid(const std::vector< Vertex > &vertices)
Constructor to create a new identified object with attribute initialization.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
Trap(const std::string &nam, double pz, double py, double px, double pLTX)
Constructor to create a new identified object for right angular wedge from STEP (Se G4 manual for det...
double deltaPhi() const
Accessor: delta-phi value.
bool isInstance(const Handle< TGeoShape > &solid)
Type check of various shapes. Result like dynamic_cast. Compare with python's isinstance(obj,...
PolyhedraRegular(NSIDES nsides, PHI_START phi_start, RMIN rmin, RMAX rmax, ZLEN zlen)
Constructor to create a new object with phi_start, deltaPhi=2PI, Z-planes at -zlen/2 and +zlen/2.
Polyhedra(const Q *p)
Constructor to be used with an existing object.
BooleanSolid()=default
Default constructor.
const TGeoMatrix * rightMatrix() const
Access right positioning matrix of the boolean.
SubtractionSolid & operator=(const SubtractionSolid ©)=default
Copy Assignment operator.
Cone & operator=(Cone &©)=default
Move Assignment operator.
void make(const std::string &nam, double a, double b, double dz)
Internal helper method to support object construction.
Sphere(RMIN rmin, RMAX rmax, STARTTHETA startTheta=0.0, ENDTHETA endTheta=M_PI, STARTPHI startPhi=0.0, ENDPHI endPhi=2. *M_PI)
Constructor to create a new anonymous object with generic attribute initialization.
EightPointSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
PolyhedraRegular()=default
Default constructor.
ShapelessSolid()=default
Default constructor.
Solid rightShape() const
Access right solid of the boolean.
ROOT::Math::RotationZYX RotationZYX
std::vector< double > zy() const
Hyperboloid & setDimensions(double rin, double stin, double rout, double stout, double dz)
Set the Hyperboloid dimensions.
PolyhedraRegular(PolyhedraRegular &&e)=default
Move Constructor.
ConeSegment & operator=(const ConeSegment ©)=default
Copy Assignment operator.
Trd2(double x1, double x2, double y1, double y2, double z)
Constructor to create a new anonymous object with attribute initialization.
EllipticalTube(EllipticalTube &&e)=default
Move Constructor.
SubtractionSolid(const Handle< Q > &e)
Constructor to be used when passing an already created object.
Trd2(const std::string &nam, double x1, double x2, double y1, double y2, double z)
Constructor to create a new identified object with attribute initialization.
double dZ() const
Accessor: delta-z value.
void make(const std::string &nam, double rmin, double rmax, double z, double startPhi, double endPhi)
Internal helper method to support object construction.
double dX2() const
Accessor: delta-x2 value.
ShapelessSolid & operator=(const ShapelessSolid ©)=default
Copy Assignment operator.
std::vector< double > lowNormal() const
Accessor: lower normal vector of cut plane.
void make(const std::string &nam, int nsides, double start, double delta, const std::vector< double > &z, const std::vector< double > &rmin, const std::vector< double > &rmax)
Helper function to create the polyhedron.
std::vector< double > _extract_vector(const SOLID *solid, double(SOLID::*extract)(Int_t) const, Int_t(SOLID::*len)() const)
double rMax() const
Accessor: r-max value.
Solid_type< T > & setName(const char *value)
Set new shape name.
EllipticalTube & operator=(const EllipticalTube ©)=default
Copy Assignment operator.
Class describing a tube shape of a section of a tube.
TwistedTube & setDimensions(double a, double b, double dz)
Set the tube dimensions.
int num_facet() const
Access the number of facets in the shape.
Box(const std::string &nam, const X &x_val, const Y &y_val, const Z &z_val)
Constructor to create a named new box object (retrieves name from volume)
TwistedTube(TwistedTube &&e)=default
Move Constructor.
std::vector< double > dimensions()
Access the dimensions of the shape: inverse of the setDimensions member function.
Box & operator=(const Box ©)=default
Copy Assignment operator.
Hyperboloid(const std::string &nam, double rin, double stin, double rout, double stout, double dz)
Constructor to create a new identified object with attribute initialization.
Solid_type & operator=(Solid_type &©)=default
Assignment move operator.
PseudoTrap(const Q *p)
Constructor to be used with an existing object.
std::string get_shape_tag(const TGeoShape *shape)
Retrieve tag name from shape type.
std::vector< double > _make_vector(const double *values, size_t length)
BooleanSolid(const BooleanSolid &b)=default
Copy Constructor.
Scale(const Scale &e)=default
Copy constructor.
std::vector< double > zPlaneZ() const
Accessor: vector of z-values for Z-planes value.
double rHigh() const
Accessor: r-max value.
double rMax2() const
Accessor: r-max-2 value.
Paraboloid(const std::string &nam, double r_low, double r_high, double delta_z)
Constructor to create a new identified object with attribute initialization.
double scale_z() const
Access z-scale factor.
HalfSpace & operator=(HalfSpace &©)=default
Move Assignment operator.
HalfSpace(const Handle< Q > &e)
Constructor to be used with an existing object.
std::string toStringMesh(const TGeoShape *shape, int precision=2)
Output mesh vertices to string.
ExtrudedPolygon(const ExtrudedPolygon &e)=default
Copy Constructor.
Sphere & setDimensions(double rmin, double rmax, double startTheta, double endTheta, double startPhi, double endPhi)
Set the Sphere dimensions.
Polycone(const Q *p)
Constructor to be used with an existing object.
ConeSegment(const ConeSegment &e)=default
Copy Constructor.
Trap(const Q *p)
Constructor to be used with an existing object.
double rMax2() const
Accessor: r-max-2 value.
Trap()=default
Default constructor.
Class describing boolean subtraction solid.
TwistedTube(double twist_angle, double rmin, double rmax, double dz, double dphi)
Constructor to create a new anonymous tube object with attribute initialization.
double dZ() const
Accessor: delta-z value.
int numEdges() const
Accessor: Number of edges.
Polyhedra(const std::string &nam, int nsides, double start, double delta, const std::vector< double > &z, const std::vector< double > &r)
Constructor to create a new object. Phi(start), deltaPhi, Z-planes at specified positions.
double rMax() const
Accessor: r-max value.
Solid_type(Solid_type &&e)=default
Move constructor.
std::vector< double > zPlaneRmax() const
Accessor: vector of rMax-values for Z-planes value.
ShapelessSolid(const Q *p)
Constructor to be used with an existing object.
Hyperboloid(Hyperboloid &&e)=default
Move Constructor.
double rMax() const
Accessor: r-max value.
double endPhi() const
Accessor: end-phi value.
std::vector< double > zPlaneRmin() const
Accessor: vector of rMin-values for Z-planes value.
Paraboloid(Paraboloid &&e)=default
Move Constructor.
void make(const std::string &name, double dz, double rmin, double rmax, double startPhi, double deltaPhi, double cutAtStart, double cutAtDelta, bool cutInside)
Internal helper method to support object construction.
Torus & operator=(Torus &©)=default
Move Assignment operator.
Class describing a Paraboloid shape.
Trd1()=default
Default constructor.
const TGeoMatrix * leftMatrix() const
Access left positioning matrix of the boolean.
double alpha1() const
Angle between centers of x edges and y axis at low z.
Trd2(const std::string &nam, X1 x1, X2 x2, Y1 y1, Y2 y2, Z z)
Constructor to create a new identified object with attribute initialization.
Trd1(const Trd1 &e)=default
Copy Constructor.
TwistedTube(const std::string &nam, double twist_angle, double rmin, double rmax, double dz, int nsegments, double totphi)
Constructor to create a new anonymous tube object with attribute initialization.
EllipticalTube & setDimensions(double a, double b, double dz)
Set the tube dimensions.
ConeSegment(double dz, double rmin1, double rmax1, double rmin2, double rmax2, double startPhi=0.0, double endPhi=2.0 *M_PI)
Constructor to create a new ConeSegment object.
double rLow() const
Accessor: r-min value.
TessellatedSolid(const std::string &nam, const std::vector< Vertex > &vertices)
Constructor to create a new identified object with attribute initialization.
Box(const Box &e)=default
Copy constructor.
ConeSegment()=default
Default constructor.