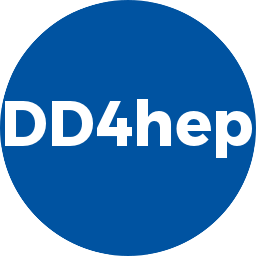 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #include <DD4hep/detail/Handle.inl>
44 static void* ddcond_create_manager_instance(
dd4hep::Detector& description,
int,
char**) {
55 int s_debug = dd4hep::INFO;
80 if ( typ && !iov->
has_range() )
return typ;
87 if ( typ && iov->
has_range() )
return typ;
95 dd4hep::except(
"ConditionsMgr",
"+++ Invalid IOV to access condition: %16llX. [Null-reference]",
key);
101 dd4hep::except(
"ConditionsMgr",
"+++ Invalid IOV type [%d] to access condition: %16llX.",
107 bool is_range_complete(
const dd4hep::IOV& iov,
const RC& conditions) {
108 if ( !conditions.empty() ) {
114 for( std::size_t j = 0; j < conditions.size(); ++j ) {
115 for(
const auto&
cond : conditions ) {
117 if ( k.first <= test.first+1 && k.second >= test.first ) test.first = k.second;
118 if ( k.first+1 <= test.second && k.second >= test.second ) test.second = k.first;
120 if ( test.first >= test.second )
return true;
122 if ( test.first <= iov.
keyData.first && test.second >= iov.
keyData.second )
return false;
128 template <
typename PMF>
130 for(
const auto& listener : listeners )
131 (listener.first->*pmf)(
cond, listener.second);
138 m_updateLock(), m_poolLock(), m_updatePool(), m_rawPool(), m_locked(0)
152 for_each(
m_rawPool.begin(),
m_rawPool.end(), detail::DestroyObject<ConditionsIOVPool*>());
159 const void* argv_loader[] = {
"ConditionsDataLoader",
this, 0};
160 const void* argv_pool[] = {
this, 0, 0};
164 except(
"ConditionsMgr",
"+++ The update pool of type %s cannot be created. [%s]",
174 std::pair<bool, const dd4hep::IOVType*>
178 bool eq_type = typ.
type == iov_index;
179 bool eq_name = typ.
name == iov_name;
180 if ( eq_type && eq_name ) {
181 return {
false, &typ };
183 else if ( typ.
type != 0 && eq_type && !eq_name ) {
184 except(
"ConditionsMgr",
"Cannot register IOV %s. Type %d already in use!",
185 iov_name.c_str(), iov_index);
188 typ.
type = iov_index;
190 return {
true, &typ };
192 except(
"ConditionsMgr",
"Cannot register IOV section %d of type %d. Value out of bounds: [%d,%d]",
193 iov_name.c_str(), iov_index, 0,
int(
m_iovTypes.size()));
194 return {
false,
nullptr };
201 if ( typ.
type == iov_index )
return &typ;
203 except(
"ConditionsMgr",
"Request to access an unregistered IOV type: %d.", iov_index);
210 if ( i.name == iov_name )
return &i;
211 except(
"ConditionsMgr",
"Request to access an unregistered IOV type: %s.", iov_name.c_str());
223 ConditionsIOVPool::Elements::const_iterator i = pool->
elements.find(
key);
225 return (*i).second.get();
230 const void* argv_pool[] = {
this, iov, 0};
231 std::shared_ptr<ConditionsPool> cond_pool(createPlugin<ConditionsPool>(
m_poolType,
m_detDesc,2,argv_pool));
233 printout(INFO,
"ConditionsMgr",
"Created IOV Pool for:%s",iov->
str().c_str());
234 return cond_pool.get();
244 if (
cond.isValid() ) {
248 #if !defined(DD4HEP_MINIMAL_CONDITIONS) && defined(DD4HEP_CONDITIONS_HAVE_NAME)
249 printout(DEBUG,
"ConditionsMgr",
"Register condition %016lX %s [%s] IOV:%s",
251 #elif defined(DD4HEP_CONDITIONS_HAVE_NAME)
252 printout(DEBUG,
"ConditionsMgr",
"Register condition %016lX %s IOV:%s",
255 printout(DEBUG,
"ConditionsMgr",
"Register condition %016lX IOV:%s",
263 else if ( !
cond.isValid() )
264 except(
"ConditionsMgr",
"+++ Invalid condition objects may not be registered. [%s]",
271 std::size_t result = 0;
283 except(
"ConditionsMgr",
284 "+++ Invalid condition objects may not be registered. [%s]",
298 #if !defined(DD4HEP_MINIMAL_CONDITIONS)
306 if ( s_debug > INFO ) {
307 #if defined(DD4HEP_MINIMAL_CONDITIONS)
309 printout(INFO,
"Conditions",
"+++ Loaded condition: %s %08X.%08X to %s",
313 printout(INFO,
"Conditions",
"+++ Loaded condition: %s.%s to %s [%s] V: %s",
325 std::unique_ptr<UserPool>& up)
327 const IOVType* typ = check_iov_type<Discrete>(
this, &req_iov);
330 if ( 0 == up.get() ) {
331 const void* argv[] = {
this, pool, 0};
338 except(
"ConditionsMgr",
"+++ Unknown IOV type requested to enable conditions. [%s]",
353 count += pool->
clean(max_age);
360 std::pair<int,int> count(0,0);
363 if ( p && cleaner(*p) ) {
365 count.second += p->
clean(cleaner);
373 std::pair<int,int> count(0,0);
378 count.second += p->
clean(0);
393 for(
const auto& iov_iter :
entries ) {
395 if ( !ents.empty() ) {
398 except(
"ConditionsMgr",
399 "+++ We should never end up here [%s]. FIXME!!!!",
409 const IOV& req_validity,
415 p->
select(
key, req_validity, conditions);
421 return !conditions.empty();
426 const IOV& req_validity,
439 return is_range_complete(req_validity,conditions);
447 __check_values__<Discrete>(
this,
key, &iov);
453 if ( conditions.size() == 1 ) {
455 return conditions[0];
457 else if ( conditions.empty() ) {
458 except(
"ConditionsMgr",
"+++ Condition %16llX for the requested IOV %s do not exist.",
461 else if ( conditions.size() > 1 ) {
462 RC::const_iterator start = conditions.begin();
463 Condition first = *start;
464 printout(ERROR,
"ConditionsMgr",
"+++ Condition %s [%16llX] is ambiguous for IOV %s:",
465 first.name(),
key, iov.
str().c_str());
466 for(RC::const_iterator i=start; i!=conditions.end(); ++i) {
468 printout(ERROR,
"ConditionsMgr",
"+++ %s [%s] = %s",
469 c.name(), c->iov->str().c_str(), c->value.c_str());
471 except(
"ConditionsMgr",
"+++ Condition %s [%16llX] is ambiguous for IOV %s:",
472 first.name(),
key, iov.
str().c_str());
482 __check_values__<Range>(
this,
key, &iov);
490 if ( conditions.empty() ) {
491 except(
"ConditionsMgr",
"+++ Conditions %16llX for IOV %s do not exist.",
492 key, iov.str().c_str());
498 except(
"ConditionsMgr",
"+++ Conditions %16llX for IOV %s do not exist.",
499 key, iov.str().c_str());
513 Result res = slice.
pool->prepare(req_iov, slice, ctx);
528 Result res = slice.
pool->load(req_iov, slice, ctx);
535 Result res = slice.
pool->compute(req_iov, slice, ctx);
547 const void* argv[] = {
this, p, 0};
552 except(
"ConditionsMgr",
"+++ Unknown IOV type requested to enable conditions. [%s]",
554 return std::unique_ptr<UserPool>();
std::string value
Condition value (in string form)
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
std::vector< Condition > RangeConditions
std::string m_userType
Property: UserPool constructor type (default: DD4hep_ConditionsLinearUserPool)
std::string name
String name.
AlignmentCondition::Object * cond
virtual const IOVType * iovType(size_t iov_index) const final
Access IOV by its type.
void declareProperty(const std::string &nam, T &val)
Declare property.
void initialize()
Access to managed pool of typed conditions indexed by IOV-type and IOV key.
Condition::key_type hash
Hash value of the name.
std::vector< Condition > ConditionEntries
std::string m_poolType
Property: ConditionsPool constructor type (default: empty. MUST BE SET!)
Implementation of an object supporting arbitrary user extensions.
virtual Result load(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) final
Load all updates to the clients with the defined IOV (1rst step of prepare)
Loader m_loader
Reference to the data loader userd by this instance.
std::string m_updateType
Property: UpdatePool constructor type (default: DD4hep_ConditionsLinearUpdatePool)
virtual std::unique_ptr< UserPool > createUserPool(const IOVType *iovT) const
Create empty user pool object.
virtual bool insert(Condition cond)=0
Register a new condition to this pool.
std::string str() const
Create string representation of the IOV.
virtual Result compute(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) final
Compute all derived conditions with the defined IOV (2nd step of prepare)
std::pair< Key_value_type, Key_value_type > Key
static void increment(T *)
Increment count according to type information.
std::string address
Condition address.
Elements elements
Container of IOV dependent conditions pools.
int clean(int max_age)
Remove all key based pools with an age beyon the minimum age.
virtual std::pair< int, int > clear() final
Full cleanup of all managed conditions.
ConditionUpdateUserContext class used by the derived conditions calculation mechanism.
Helper union to interprete conditions keys.
Listeners m_onRegister
Conditions listeners on registration of new conditions.
The data class behind a conditions manager handle.
Class describing the interval of validty type.
std::string value
The actual conditions data.
void SetName(const char *nam)
Set name (used by Handle)
dd4hep::RangeConditions RC
void __get_checked_pool(const IOV &required_validity, std::unique_ptr< UserPool > &user_pool)
Register a set of new managed condition for an IOV range. Called by __load_immediate.
std::unique_ptr< ConditionsCleanup > m_cleaner
Reference to the default conditions cleanup object (if registered)
std::string invalidArg()
System error string for EINVAL. Sets errno accordingly.
Basic conditions manager implementation.
Main condition object handle.
Namespace for implementation details of the AIDA detector description toolkit.
Class describing the interval of validty.
Do-nothing compatibility std::unique_ptr emulation for cxx-98.
Class implementing the conditions collection for a given IOV type.
static void decrement(T *)
Decrement count according to type information.
Key keyData
IOV key (if second==first, discrete, otherwise range)
TypedConditionPool m_rawPool
Managed pool of typed conditions indexed by IOV-type and IOV key.
#define DECLARE_DD4HEP_CONSTRUCTOR(name, func)
IOV * iov
The IOV of the conditions hosted.
DetElement detector
Reference to the detector element.
void SetTitle(const char *tit)
Set Title (used by Handle)
dd4hep_mutex_t m_poolLock
Lock to protect the pool of all known conditions.
size_t select(Condition::key_type key, const IOV &req_validity, RangeConditions &result)
Retrieve a condition set given the key according to their validity.
bool has_range() const
Check if the IOV corresponds to a range.
Condition __queue_update(cond::Entry *data)
std::string noSys()
System error string for ENOSYS. Sets errno accordingly.
Manager_Type1(Detector &description)
Standard constructor.
std::string comment
Comment string.
std::vector< IOVType > m_iovTypes
Collection of IOV types managed.
Detector & m_detDesc
Reference to main detector description object.
std::string validity
Condition validity (in string form)
virtual Result prepare(const IOV &req_iov, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt) final
Prepare all updates for the given keys to the clients with the defined IOV.
unsigned long long int key_type
Forward definition of the key type.
std::string validity
The validity string to be interpreted by the updating engine.
ConditionsPool * registerIOV(const std::string &data)
Register IOV using new string data.
The data class behind a conditions handle.
unsigned int type
IOV buffer type: Must be a bitmap!
Interface for conditions pool optimized to host conditions updates.
int m_maxIOVTypes
Property: maximal number of IOV types to be handled.
std::unique_ptr< UserPool > pool
Reference to the user pool managing all conditions of this slice.
Result of a prepare call to the conditions manager.
DD4HEP_INSTANTIATE_HANDLE_NAMED(Manager_Type1)
virtual void adoptCleanup(ConditionsCleanup *cleaner) final
Adopt cleanup handler. If a handler is registered, it is invoked at every "prepare" step.
Base class to handle conditions cleanups.
virtual ~Manager_Type1()
Default destructor.
virtual bool registerUnlocked(ConditionsPool &pool, Condition cond) final
Register new condition with the conditions store. Unlocked version, not multi-threaded.
T * ptr() const
Access to the held object.
std::unique_ptr< UpdatePool > m_updatePool
Reference to update conditions pool.
virtual int clean(const IOVType *typ, int max_age) final
Clean conditions, which are above the age limit.
const IOVType * iovType
Reference to IOV type.
UpdatePool::UpdateEntries Updates
virtual void onRegisterCondition(Condition, void *)
ConditionsListener dummy implementation: onRegister new condition.
dd4hep_mutex_t m_updateLock
Lock to protect the update/delayed conditions pool.
std::string m_loaderType
Property: Conditions loader type (default: "multi" -> DD4hep_Conditions_multi_Loader)
std::set< Listener > Listeners
bool select(key_type key, const IOV &req_validity, RangeConditions &conditions)
Retrieve a condition set given a Detector Element and the conditions name according to their validity...
virtual size_t blockRegister(ConditionsPool &pool, const std::vector< Condition > &cond) const final
Register a whole block of conditions with identical IOV.
std::map< const IOV *, ConditionEntries > UpdateEntries
Update container specification.
The main interface to the dd4hep detector description package.
std::string name
The object name.
virtual std::pair< bool, const IOVType * > registerIOVType(size_t iov_index, const std::string &iov_name) final
Register new IOV type if it does not (yet) exist.
std::string type
The object type.
virtual ConditionsIOVPool * iovPool(const IOVType &type) const final
Access conditions multi IOV pool by iov type.
unsigned int type
integer identifier used internally
const IOV * iov
Interval of validity.
Pool of conditions satisfying one IOV type (epoch, run, fill, etc)
virtual ConditionsPool * registerIOV(const IOVType &typ, IOV::Key key) final
Register IOV with type and key.
size_t selectRange(Condition::key_type key, const IOV &req_validity, RangeConditions &result)
Retrieve a condition set given the key according to their validity.
bool select_range(key_type key, const IOV &req_validity, RangeConditions &conditions)
Retrieve a condition set given a Detector Element and the conditions name according to their validity...
virtual void pushUpdates() final
Push all pending updates to the conditions store.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
The intermediate conditions data used to populate the DetElement conditions.