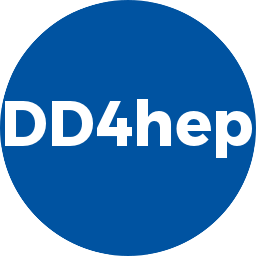 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSPOOL_H
14 #define DDCOND_CONDITIONSPOOL_H
30 class ConditionsSlice;
31 class ConditionsIOVPool;
32 class ConditionDependency;
83 void print(
const std::string& opt)
const;
85 virtual size_t size()
const = 0;
125 const IOV& req_validity,
137 typedef std::map<Condition::key_type,const ConditionDependency*>
Dependencies;
171 virtual void print(
const std::string& opt)
const = 0;
173 virtual size_t size()
const = 0;
192 virtual size_t registerMany(
const IOV& iov,
const std::vector<Condition>& values) = 0;
195 std::vector<Condition> conditions;
196 conditions.reserve(c.size());
197 std::copy(std::begin(c), std::end(c), std::back_inserter(conditions));
202 std::vector<Condition> conditions;
203 conditions.reserve(c.size());
204 std::transform(std::begin(c), std::end(c), std::back_inserter(conditions), detail::get_2nd<CONT>());
253 #endif // DDCOND_CONDITIONSPOOL_H
std::vector< Condition > RangeConditions
virtual bool exists(Condition::key_type key) const =0
Check a condition for existence.
virtual size_t compute(const Dependencies &dependencies, ConditionUpdateUserContext *user_param, bool force)=0
Evaluate and register all derived conditions from the dependency list.
virtual std::vector< Condition > get(Condition::key_type lower, Condition::key_type upper) const =0
No ConditionsMap overload: Access all conditions within a key range in the interval [lower,...
AlignmentCondition::Object * cond
virtual size_t registerMany(const IOV &iov, const std::vector< Condition > &values)=0
Do block insertions of conditions with identical IOV.
int age_value
Aging value.
const IOV * validityPtr() const
Access the interval of validity for this user pool.
std::vector< Condition > ConditionEntries
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition)=0
ConditionsMap overload: Add a condition directly to the slice.
void onRegister(Condition condition)
Listener invocation when a condition is registered to the cache.
virtual bool insert(Condition cond)=0
Register a new condition to this pool.
virtual bool exists(const ConditionKey &key) const =0
Check a condition for existence.
virtual void scan(DetElement detector, Condition::itemkey_type lower, Condition::itemkey_type upper, const Condition::Processor &processor) const =0
No ConditionsMap overload: Interface to scan data content of the conditions mapping.
Key definition to optimize ans simplyfy the access to conditions entities.
ConditionsManager m_manager
Handle to conditions manager object.
ConditionUpdateUserContext class used by the derived conditions calculation mechanism.
const IOV & validity() const
Access the interval of validity for this user pool.
Conditions selector functor. Default implementation selects everything evaluated.
virtual size_t popEntries(UpdateEntries &entries)=0
Adopt all entries sorted by IOV. Entries will be removed from the pool.
virtual Condition get(const ConditionKey &key) const =0
Check if a condition exists in the pool and return it to the caller.
virtual bool remove(Condition::key_type hash_key)=0
Remove condition by key from pool.
virtual ConditionsManager::Result load(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0)=0
Load all updates to the clients with the defined IOV (1rst step of prepare)
virtual size_t select_all(RangeConditions &result)=0
Select all conditions contained.
Main condition object handle.
virtual ~ConditionsPool()
Default destructor. Note: pool must be cleared by the subclass!
virtual void scan(Condition::key_type lower, Condition::key_type upper, const Condition::Processor &processor) const =0
No ConditionsMap overload: Interface to scan data content of the conditions mapping.
Class describing the interval of validty.
virtual void print(const std::string &opt) const =0
Print pool content.
unsigned int flags
Processing flags (printout etc.)
Handle class describing a detector element.
UserPool(ConditionsManager mgr)
Default constructor.
size_t registerUnmapped(const IOV &iov, CONT &c)
Insert multiple conditions. Note: the conditions must already have a hash key.
virtual bool insert(Condition condition)=0
ConditionsMap overload: Add a condition directly to the slice.
Class implementing the conditions collection for a given IOV type.
virtual bool registerOne(const IOV &iov, Condition cond)=0
Do single insertion of condition including registration to the manager.
virtual Condition exists(Condition::key_type key) const =0
Check if a condition exists in the pool.
virtual size_t select_all(ConditionsPool &selection_pool)=0
Select all conditions contained.
void print() const
Print pool basics.
IOV m_iov
The pool's interval of validity.
IOV * iov
The IOV of the conditions hosted.
virtual size_t select(Condition::key_type key, RangeConditions &result)=0
Select the conditions matching the DetElement and the conditions name.
virtual size_t size() const =0
Total entry count.
void onRemove(Condition condition)
Listener invocation when a condition is deregistered from the cache.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
virtual size_t select_all(const ConditionsSelect &predicate)=0
Select the conditions, passing a predicate.
virtual ConditionsManager::Result compute(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0)=0
Compute all derived conditions with the defined IOV (2nd step of prepare)
virtual size_t size() const =0
Total entry count.
unsigned long long int key_type
Forward definition of the key type.
virtual std::vector< Condition > get(DetElement detector, Condition::itemkey_type lower, Condition::itemkey_type upper) const =0
No ConditionsMap overload: Access all conditions within a key range in the interval [lower,...
virtual ~UpdatePool()
Default destructor.
virtual void select_range(Condition::key_type key, const IOV &req_validity, RangeConditions &result)=0
Select the conditions matching the key.
Manager class for condition handles.
Interface for conditions pool optimized to host conditions updates.
virtual void scan(const Condition::Processor &processor) const =0
ConditionsMap overload: Interface to scan data content of the conditions mapping.
Result of a prepare call to the conditions manager.
ConditionsManager m_manager
Handle to conditions manager object.
virtual void clear()=0
Full cleanup of all managed conditions.
virtual void insert(RangeConditions &cond)=0
Register a new condition to this pool. May overload for performance reasons.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
std::map< const IOV *, ConditionEntries > UpdateEntries
Update container specification.
UpdatePool(ConditionsManager mgr, IOV *iov)
Default constructor.
virtual bool remove(const ConditionKey &key)=0
Remove condition by key from pool.
virtual Condition get(Condition::key_type key) const =0
Check if a condition exists in the pool and return it to the caller.
virtual ~UserPool()
Default destructor.
Interface for conditions pool optimized to host conditions updates.
virtual Condition get(DetElement detector, Condition::itemkey_type key) const =0
ConditionsMap overload: Access a single condition.
virtual ConditionsManager::Result prepare(const IOV &required, ConditionsSlice &slice, ConditionUpdateUserContext *user_param=0)=0
Prepare user pool for usage (load, fill etc.) according to required IOV.
size_t registerMapping(const IOV &iov, CONT &c)
Insert multiple conditions. Note: the conditions must already have a hash key.
Abstract base for processing callbacks to conditions objects.
virtual void clear()=0
Full cleanup of all managed conditions.
std::map< Condition::key_type, const ConditionDependency * > Dependencies
Forward definition of the key type.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
ConditionsPool(ConditionsManager mgr, IOV *iov)
Default constructor.