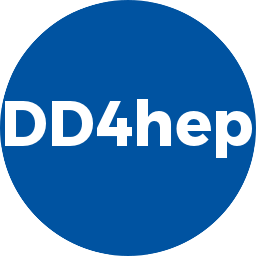 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_CONDITIONDERIVED_H
14 #define DD4HEP_CONDITIONDERIVED_H
34 class DependencyBuilder;
35 class ConditionResolver;
36 class ConditionDependency;
37 class ConditionUpdateCall;
38 class ConditionUpdateContext;
39 class ConditionUpdateUserContext;
84 bool throw_if_not) = 0;
97 virtual size_t registerMany(
const IOV& iov,
const std::vector<Condition>& values) = 0;
100 std::vector<Condition> conditions;
101 conditions.reserve(c.size());
102 std::copy(std::begin(c), std::end(c), std::back_inserter(conditions));
107 std::vector<Condition> conditions;
108 conditions.reserve(c.size());
109 std::transform(std::begin(c), std::end(c), std::back_inserter(conditions), detail::get_2nd<CONT>());
179 template<
typename Q> Q*
param()
const {
239 template<
typename T>
const T&
get(
const ConditionKey& key_value)
const;
263 std::vector<Condition> conds;
264 conds.reserve(values.size());
265 std::copy(std::begin(values), std::end(values), std::back_inserter(conds));
270 std::vector<Condition> conds;
271 conds.reserve(values.size());
272 std::transform(std::begin(values), std::end(values), std::back_inserter(conds), detail::get_2nd<CONT>());
317 friend std::default_delete<ConditionDependency>;
349 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
350 const char* name()
const {
return target.name.c_str(); }
389 : resolver(resolv), dependency(dep), iov(i), parameter(user_param)
400 template<
typename T>
inline T&
403 if (
cond.isValid() ) {
404 return cond.get<T>();
407 throw std::runtime_error(
"ConditionUpdateCall");
411 template<
typename T>
inline const T&
414 if (
cond.isValid() ) {
415 return cond.get<T>();
418 throw std::runtime_error(
"ConditionUpdateCall");
422 template<
typename T>
inline T&
428 template<
typename T>
inline const T&
435 #endif // DD4HEP_CONDITIONDERIVED_H
ConditionResolver class used by the derived conditions calculation mechanism.
virtual ~ConditionDependency()
Default destructor.
virtual Condition get(Condition::key_type key)=0
Interface to access conditions by hash value.
AlignmentCondition::Object * cond
virtual ~ConditionResolver()
Standard destructor.
virtual ConditionsMap & conditionsMap() const =0
Accessor for the current conditons mapping.
virtual Handle< NamedObject > manager() const =0
Access to the conditions manager.
virtual Condition get(const ConditionKey &key)=0
Interface to access conditions by conditions key.
ConditionResolver * resolver
Internal reference to the resolver to access other conditions (Be careful)
ConditionDependency & operator=(const ConditionDependency &c)=delete
Assignment operator.
Condition::key_type hash
Hashed key representation.
void accessFailure(const ConditionKey &key_value) const
Throw exception on conditions access failure.
ConditionUpdateUserContext * parameter
A refernce to the user parameter.
virtual std::vector< Condition > getByItem(Condition::itemkey_type key)=0
Interface to access conditions by hash value of the item (only valid at resolve!)
ConditionKey target
Key to the condition to be updated.
DetElement world() const
Access to the top level detector element.
T & get(const ConditionKey &key_value)
Access of other conditions data from the resolver.
size_t registerUnmapped(const IOV &iov, CONT &c)
Handle multi-condition inserts by callbacks with identical key. Handle unmapped containers.
ConditionDependency * release()
Release the created dependency and take ownership.
ConditionUpdateCall()
Standard destructor.
void release()
Release object. May not be used any longer.
IOV * iov
The reference to the combined IOV resulting from the cumputation.
DetElement detector
Reference to the target's detector element.
std::unique_ptr< ConditionDependency > m_dependency
The created dependency.
virtual bool registerOne(const IOV &iov, Condition cond)=0
Interface to handle multi-condition inserts by callbacks: One single insert.
Key definition to optimize ans simplyfy the access to conditions entities.
virtual Condition get(Condition::key_type key, bool throw_if_not)=0
Interface to access conditions by hash value.
const ConditionKey & key(size_t which) const
Access to dependency keys.
ConditionUpdateUserContext class used by the derived conditions calculation mechanism.
int m_refCount
Reference count.
ConditionUpdateCall & operator=(const ConditionUpdateCall ©)=delete
No assignment operator.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
ConditionUpdateCall(const ConditionUpdateCall ©)=delete
No copy constructor.
Condition dependency definition.
virtual std::vector< Condition > get(Condition::detkey_type key)=0
Interface to access conditions by hash value of the DetElement (only valid at resolve!...
Class describing the interval of validty.
size_t registerMany(const IOV &iov, const std::vector< Condition > &values)
Handle multi-condition inserts by callbacks: block insertions of conditions with identical IOV.
Condition condition(const ConditionKey &key_value) const
Access to condition object by dependency key.
Handle class describing a detector element.
virtual size_t registerMany(const IOV &iov, const std::vector< Condition > &values)=0
Handle multi-condition inserts by callbacks: block insertions of conditions with identical IOV.
Condition::key_type key() const
Access the dependency key.
ConditionDependency(const ConditionDependency &c)=delete
Copy constructor.
Q * param() const
Access user parameter.
virtual ~ConditionUpdateUserContext()
Default destructor.
size_t registerMapping(const IOV &iov, CONT &c)
Handle multi-condition inserts by callbacks with identical key. Handle mapped containers.
std::shared_ptr< ConditionUpdateCall > callback
Reference to the update callback. No auto pointer. callback may be shared.
bool registerOne(const IOV &iov, Condition cond)
Interface to handle multi-condition inserts by callbacks: One single insert.
size_t registerMapping(const IOV &iov_val, CONT &values)
Handle multi-condition inserts by callbacks with identical key. Handle mapped containers.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
virtual ~DependencyBuilder()
Default destructor.
unsigned long long int key_type
Forward definition of the key type.
virtual ~ConditionUpdateCall()
Standard destructor.
virtual Condition operator()(const ConditionKey &target, ConditionUpdateContext &ctxt)=0
Interface to client callback in order to update/create the condition.
Detector & detectorDescription() const
Access to the detector description instance.
void add(const ConditionKey &source_key)
Add a new dependency.
Condition dependency builder.
std::vector< ConditionKey > dependencies
Dependency keys this condition depends on.
virtual Condition get(const ConditionKey &key, bool throw_if_not)=0
Interface to access conditions by conditions key.
virtual void resolve(Condition, ConditionUpdateContext &)
Interface to client callback for resolving references or to use data from other conditions.
Namespace for the AIDA detector description toolkit.
virtual Detector & detectorDescription() const =0
Access to the detector description instance.
ConditionDependency * operator->()
Access underlying object directly.
ConditionsMap & conditionsMap() const
Accessor for the current conditons mapping.
const IOV & requiredValidity() const
Required IOV value for update cycle.
size_t registerUnmapped(const IOV &iov_val, CONT &values)
Handle multi-condition inserts by callbacks with identical key. Handle unmapped containers.
The main interface to the dd4hep detector description package.
virtual Condition get(Condition::key_type key, const ConditionDependency *dependency, bool throw_if_not)=0
Interface to access conditions by hash value.
DependencyBuilder(DetElement de, Condition::itemkey_type item_key, std::shared_ptr< ConditionUpdateCall > call)
Initializing constructor.
ConditionUpdateContext(ConditionResolver *r, const ConditionDependency *d, IOV *iov, ConditionUpdateUserContext *parameter)
Initializing constructor.
virtual std::vector< Condition > get(DetElement de)=0
Interface to access conditions by hash value of the DetElement (only valid at resolve!...
const ConditionDependency * dependency
The dependency to be handled within this context.
std::vector< Condition > getByItem(Condition::itemkey_type key) const
Access conditions by the condition item key.
virtual const IOV & requiredValidity() const =0
Required IOV value for update cycle.
ConditionUpdateContext class used by the derived conditions calculation mechanism.
ConditionDependency()
Default constructor.
ConditionDependency * addRef()
Add use count to the object.
std::vector< Condition > conditions(DetElement det) const
Access to all conditions of a detector element.