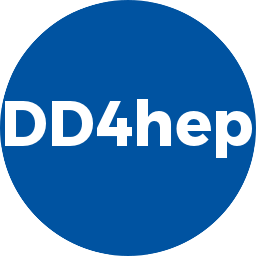 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #ifndef DD4HEP_DETAIL_CONDITIONSINTERNA_H
22 #define DD4HEP_DETAIL_CONDITIONSINTERNA_H
65 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
72 #if defined(DD4HEP_CONDITIONS_DEBUG) || !defined(DD4HEP_MINIMAL_CONDITIONS)
131 #endif // DD4HEP_DETAIL_CONDITIONSINTERNA_H
std::string value
Condition value (in string form)
bool is_bound() const
Check if object is already bound....
void * payload() const
Access the bound data payload. Exception id object is unbound.
Condition::key_type hash
Hash value of the name.
ConditionObject & operator=(const ConditionObject &)=delete
No assignment operation.
Condition::mask_type flags
Flags.
Class describing an opaque conditions data block.
std::string address
Condition address.
ConditionObject * addRef()
Increase reference counter (Used by persistency mechanism)
bool is_bound() const
Check if object is already bound....
Class describing the interval of validty type.
void unFlag(Condition::mask_type option)
Flag operations: UN-Set a conditons flag.
virtual ~ConditionObject()
Standard Destructor.
Class describing the interval of validty.
ConditionObject()
Default constructor.
ConditionObject(ConditionObject &&)=delete
const IOVType * iovType() const
Access safely the IOV-type.
ConditionObject & move(ConditionObject &from)
Move data content: 'from' will be reset to NULL.
void release()
Release object (Used by persistency mechanism)
int refCount
Reference count.
static size_t offset()
Data offset from the opaque data block pointer to the condition.
std::string comment
Comment string.
std::string validity
Condition validity (in string form)
ConditionObject & operator=(ConditionObject &&)=delete
No move assignment operator.
void setFlag(Condition::mask_type option)
Flag operations: Set a conditons flag.
unsigned long long int key_type
Forward definition of the key type.
const IOV * iovData() const
Access safely the IOV.
unsigned int mask_type
Forward definition of the object properties.
The data class behind a conditions handle.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
OpaqueDataBlock data
Data block.
ConditionObject(const ConditionObject &)=delete
No copy constructor.
const IOV * iov
Interval of validity.
bool testFlag(Condition::mask_type option) const
Flag operations: Test for a given a conditons flag.