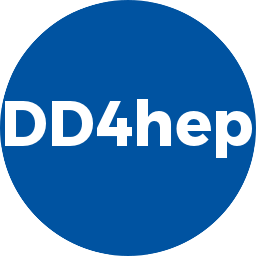 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_NAMEDOBJECT_H
14 #define DD4HEP_NAMEDOBJECT_H
42 NamedObject(
const std::string& nam,
const std::string& typ);
76 #endif // DD4HEP_NAMEDOBJECT_H
NamedObject & operator=(const NamedObject &c)=default
Assignment operator.
const char * GetName() const
Access name.
void SetName(const char *nam)
Set name (used by Handle)
NamedObject(NamedObject &&c)=default
Move constructor.
virtual ~NamedObject()=default
Default destructor.
NamedObject()=default
Standard constructor.
void SetTitle(const char *tit)
Set Title (used by Handle)
const char * GetTitle() const
Get name (used by Handle)
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
std::string name
The object name.
std::string type
The object type.
NamedObject & operator=(NamedObject &&c)=default
Move assignment operator.
NamedObject(const NamedObject &c)=default
Copy constructor.