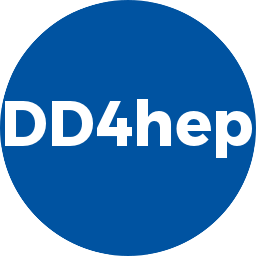 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_TYPE1_MANAGER_TYPE1_H
14 #define DDCOND_TYPE1_MANAGER_TYPE1_H
32 class ConditionsSlice;
33 class ConditionsIOVPool;
34 class ConditionsDataLoader;
122 virtual std::pair<bool, const IOVType*>
registerIOVType(
size_t iov_index,
const std::string& iov_name)
final;
131 virtual const IOVType*
iovType (
const std::string& iov_name)
const final;
150 virtual int clean(
const IOVType* typ,
int max_age)
final;
158 virtual std::pair<int,int>
clear() final;
197 #endif // DDCOND_TYPE1_MANAGER_TYPE1_H
std::vector< Condition > RangeConditions
std::string m_userType
Property: UserPool constructor type (default: DD4hep_ConditionsLinearUserPool)
AlignmentCondition::Object * cond
virtual const IOVType * iovType(size_t iov_index) const final
Access IOV by its type.
void initialize()
Access to managed pool of typed conditions indexed by IOV-type and IOV key.
std::string m_poolType
Property: ConditionsPool constructor type (default: empty. MUST BE SET!)
Implementation of an object supporting arbitrary user extensions.
virtual Result load(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) final
Load all updates to the clients with the defined IOV (1rst step of prepare)
virtual const std::vector< IOVType > & iovTypes() const final
Access IOV by its type.
std::string m_updateType
Property: UpdatePool constructor type (default: DD4hep_ConditionsLinearUpdatePool)
GlobalAlignmentStack::StackEntry Entry
virtual std::unique_ptr< UserPool > createUserPool(const IOVType *iovT) const
Create empty user pool object.
virtual Result compute(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) final
Compute all derived conditions with the defined IOV (2nd step of prepare)
std::pair< Key_value_type, Key_value_type > Key
virtual std::pair< int, int > clear() final
Full cleanup of all managed conditions.
ConditionUpdateUserContext class used by the derived conditions calculation mechanism.
The data class behind a conditions manager handle.
Class describing the interval of validty type.
std::recursive_mutex dd4hep_mutex_t
void __get_checked_pool(const IOV &required_validity, std::unique_ptr< UserPool > &user_pool)
Register a set of new managed condition for an IOV range. Called by __load_immediate.
std::unique_ptr< ConditionsCleanup > m_cleaner
Reference to the default conditions cleanup object (if registered)
Basic conditions manager implementation.
Main condition object handle.
Class describing the interval of validty.
Class implementing the conditions collection for a given IOV type.
Condition::key_type key_type
TypedConditionPool m_rawPool
Managed pool of typed conditions indexed by IOV-type and IOV key.
dd4hep_mutex_t m_poolLock
Lock to protect the pool of all known conditions.
Object encapsulating the result of a computation call to the alignments calculator.
Condition __queue_update(cond::Entry *data)
Manager_Type1(Detector &description)
Standard constructor.
std::vector< IOVType > m_iovTypes
Collection of IOV types managed.
int m_locked
Public access: if locked, DetElements stay intact and are not altered.
virtual Result prepare(const IOV &req_iov, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt) final
Prepare all updates for the given keys to the clients with the defined IOV.
int m_maxIOVTypes
Property: maximal number of IOV types to be handled.
virtual void adoptCleanup(ConditionsCleanup *cleaner) final
Adopt cleanup handler. If a handler is registered, it is invoked at every "prepare" step.
Base class to handle conditions cleanups.
virtual ~Manager_Type1()
Default destructor.
virtual bool registerUnlocked(ConditionsPool &pool, Condition cond) final
Register new condition with the conditions store. Unlocked version, not multi-threaded.
std::vector< ConditionsIOVPool * > TypedConditionPool
std::unique_ptr< UpdatePool > m_updatePool
Reference to update conditions pool.
virtual int clean(const IOVType *typ, int max_age) final
Clean conditions, which are above the age limit.
Namespace for the AIDA detector description toolkit.
dd4hep_mutex_t m_updateLock
Lock to protect the update/delayed conditions pool.
std::string m_loaderType
Property: Conditions loader type (default: "multi" -> DD4hep_Conditions_multi_Loader)
bool select(key_type key, const IOV &req_validity, RangeConditions &conditions)
Retrieve a condition set given a Detector Element and the conditions name according to their validity...
virtual size_t blockRegister(ConditionsPool &pool, const std::vector< Condition > &cond) const final
Register a whole block of conditions with identical IOV.
The main interface to the dd4hep detector description package.
virtual std::pair< bool, const IOVType * > registerIOVType(size_t iov_index, const std::string &iov_name) final
Register new IOV type if it does not (yet) exist.
std::string type
The object type.
virtual ConditionsIOVPool * iovPool(const IOVType &type) const final
Access conditions multi IOV pool by iov type.
Pool of conditions satisfying one IOV type (epoch, run, fill, etc)
virtual ConditionsPool * registerIOV(const IOVType &typ, IOV::Key key) final
Register IOV with type and key.
bool select_range(key_type key, const IOV &req_validity, RangeConditions &conditions)
Retrieve a condition set given a Detector Element and the conditions name according to their validity...
virtual void pushUpdates() final
Push all pending updates to the conditions store.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
The intermediate conditions data used to populate the DetElement conditions.