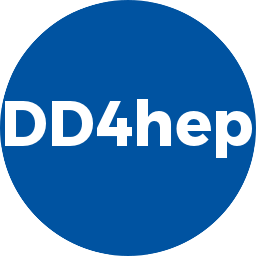 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
36 printout(ERROR,
"ConditionsIOVPool",
"+++ Unexpected exception in destructor(ConditionsIOVPool): %s",e.what());
44 size_t len = result.size();
48 e.second->select(
key, result);
51 return result.size() - len;
58 size_t len = result.size();
64 e.second->select(
key, result);
67 e.second->select(
key, result);
70 e.second->select(
key, result);
72 return result.size() - len;
82 count += e.second->
size();
83 e.second->print(
"Remove");
97 if ( e.second->age_value >= max_age ) {
98 count += e.second->size();
99 e.second->print(
"Remove");
114 size_t num_selected = 0;
119 ++i.second->age_value;
123 num_selected += i.second->select_all(
valid);
124 i.second->age_value = 0;
135 size_t num_selected = 0, pool_selected = 0;
140 ++i.second->age_value;
144 pool_selected = i.second->select_all(predicate_processor);
145 num_selected += pool_selected;
146 i.second->age_value = 0;
159 for(
const auto& i :
valid )
167 size_t num_selected = 0;
174 valid[i.first] = i.second;
183 std::vector<Element>&
valid,
188 for(
const auto& i :
valid )
196 size_t num_selected = 0;
203 valid.emplace_back(i.second);
void iov_intersection(const IOV &comparator)
Set the intersection of this IOV with the argument IOV.
std::vector< Condition > RangeConditions
ConditionsIOVPool(const IOVType *type)
Not ROOT persistent.
void exception(const std::string &src, const std::string &msg)
std::map< IOV::Key, Element > Elements
Shortcut name for the actual conditions container.
IOV & invert()
Invert the key values (first=second and second=first)
std::pair< Key_value_type, Key_value_type > Key
static void increment(T *)
Increment count according to type information.
Elements elements
Container of IOV dependent conditions pools.
int clean(int max_age)
Remove all key based pools with an age beyon the minimum age.
Conditions selector functor. Default implementation selects everything evaluated.
Class describing the interval of validty type.
Namespace for implementation details of the AIDA detector description toolkit.
Class describing the interval of validty.
virtual ~ConditionsIOVPool()
Default destructor.
Class implementing the conditions collection for a given IOV type.
static bool key_overlaps_lower_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the lower interval edge with IOV 'key'.
static void decrement(T *)
Decrement count according to type information.
static bool key_overlaps_higher_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
size_t select(Condition::key_type key, const IOV &req_validity, RangeConditions &result)
Retrieve a condition set given the key according to their validity.
virtual size_t size() const =0
Total entry count.
static bool key_is_contained(const Key &key, const Key &test)
Check if IOV 'test' is fully contained in IOV 'key'.
Key key() const
Get the local key of the IOV.
unsigned long long int key_type
Forward definition of the key type.
Base class to handle conditions cleanups.
IOV & reset()
Set keys to unphysical values (LONG_MAX, LONG_MIN)
static bool key_contains_range(const Key &key, const Key &test)
Same as above, but reverse logic. Gives sometimes more understandable logic.
size_t selectRange(Condition::key_type key, const IOV &req_validity, RangeConditions &result)
Retrieve a condition set given the key according to their validity.