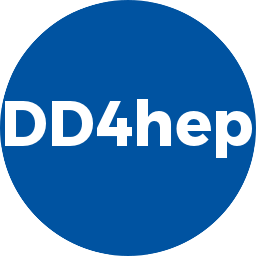 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 #include <G4SDManager.hh>
29 #include <G4VSensitiveDetector.hh>
34 #ifdef DD4HEP_USE_GEANT4_UNITS
45 G4SDManager* mgr = G4SDManager::GetSDMpointer();
48 dd4hep::except(
"Geant4Sensitive",
"DDG4: You requested to configure actions "
49 "for the sensitive detector %s,\nDDG4: which is not known to Geant4. "
50 "Are you sure you already converted the geometry?", name.c_str());
54 throw dd4hep::except(
"Geant4Sensitive",
"DDG4: You may only configure actions "
55 "for sensitive detectors of type Geant4ActionSD.\n"
56 "DDG4: The sensitive detector of %s is of type %s, which is incompatible.", name.c_str(),
57 typeName(
typeid(*sd)).c_str());
93 except(
"The filter action %s does not support the GFLASH/FastSim interface for Geant4.",
c_name());
102 if (!
det.isValid()) {
103 except(
"DDG4: Detector elemnt for %s is invalid.", nam.c_str());
132 except(
"Attempt to add invalid sensitive filter!");
148 except(
"Attempt to add invalid sensitive filter!");
153 bool (
Geant4Filter::*filter)(
const G4Step*)
const = &Geant4Filter::operator();
154 bool result =
m_filters.filter(filter, step);
161 bool result =
m_filters.filter(filter, spot);
176 except(
"DDG4: The sensitive detector for action %s was not properly configured.",
name().c_str());
177 throw std::runtime_error(
"Geant4Sensitive::detector");
224 except(
"The sensitive action %s does not support the GFLASH/FastSim interface for Geant4.",
c_name());
235 if ( truth ) truth->
mark(track);
241 if ( truth ) truth->
mark(step);
265 std::exception_ptr eptr;
267 G4ThreeVector local = h.
preTouchable()->GetHistory()->GetTopTransform().TransformPoint(global);
275 eptr = std::current_exception();
276 error(
"cellID: failed to access segmentation for VolumeID: %016lX [%ld] [%s]", volID, volID, e.what());
277 error(
"....... G4-local: (%f, %f, %f) G4-global: (%f, %f, %f)",
278 local.x(), local.y(), local.z(), global.x(), global.y(), global.z());
279 error(
"....... TGeo-local: (%f, %f, %f) TGeo-global: (%f, %f, %f)",
280 loc.x(), loc.y(), loc.z(), glob.x(), glob.y(), glob.z());
284 std::rethrow_exception(std::move(eptr));
295 std::exception_ptr eptr;
296 G4ThreeVector local = touchable->GetHistory()->GetTopTransform().TransformPoint(global);
304 auto* pvol = touchable->GetVolume();
305 auto* vol = pvol->GetLogicalVolume();
306 auto* sd = vol->GetSensitiveDetector();
307 eptr = std::current_exception();
308 error(
"cellID: failed to access segmentation for VolumeID: %016lX [%ld] [%s]", volID, volID, e.what());
309 error(
"....... G4-local: (%f, %f, %f) G4-global: (%f, %f, %f)",
310 local.x(), local.y(), local.z(), global.x(), global.y(), global.z());
311 error(
"....... TGeo-local: (%f, %f, %f) TGeo-global: (%f, %f, %f)",
312 loc.x(), loc.y(), loc.z(), glob.x(), glob.y(), glob.z());
313 error(
"....... Touchable: %s SD: %s", vol->GetName().c_str(), sd ? sd->GetName().c_str() :
"???");
314 std::rethrow_exception(std::move(eptr));
361 except(
"Attempt to add invalid sensitive actor!");
371 except(
"Attempt to add invalid sensitive filter!");
376 m_collections.emplace_back(collection_name, make_pair(owner,func));
381 std::size_t Geant4SensDetActionSequence::Geant4SensDetActionSequence::defineCollections(
Geant4ActionSD* sens_det) {
382 std::size_t count = 0;
383 m_detector = sens_det;
386 for (HitCollections::const_iterator i = m_collections.begin(); i != m_collections.end(); ++i) {
398 static std::string blank =
"";
399 except(
"The collection name index for subdetector %s is out of range!",
c_name());
410 except(
"The collection index for subdetector %s is wrong!",
c_name());
412 except(
"The collection name index for subdetector %s is out of range!",
c_name());
421 except(
"The collection index for subdetector %s is wrong!",
c_name());
427 bool (
Geant4Filter::*filter)(
const G4Step*)
const = &Geant4Filter::operator();
428 bool result =
m_filters.filter(filter, step);
435 bool result =
m_filters.filter(filter, spot);
443 if ( sensitive->accept(step) )
444 result |= sensitive->process(step, history);
454 if ( sensitive->accept(spot) )
455 result |= sensitive->processFastSim(spot, history);
467 for (std::size_t count = 0; count <
m_collections.size(); ++count) {
471 m_hce->AddHitsCollection(
id, col);
503 std::string n =
"SD_Seq_" + nam;
507 except(
"Attempt to access undefined SensDetActionSequence: %s ", nam.c_str());
513 std::string nam =
"SD_Seq_" + name;
523 std::string nam =
"SD_Seq_" + name;
528 except(
"Attempt to add invalid sensitive sequence with name:%s", name.c_str());
Geant4SensDetActionSequence * m_sequence
Reference to the containing action sequence.
SensitiveDetector m_sensitive
Reference to the sensitive detector element.
void adopt(Geant4Filter *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual void defineCollections()
Define collections created by this sensitivie action object.
bool m_needsControl
Default property: Flag to create control instance.
Detector & detectorDescription() const
Access to detector description.
void insert(const std::string &name, Geant4SensDetActionSequence *seq)
Insert sequence member.
CallbackSequence m_end
Callback sequence for event finalization action.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history)
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
Geant4SensDetActionSequence * sensitiveAction(const std::string &name)
Access to the sensitive detector action from the actioncontainer object.
Actors< Geant4Sensitive > m_actors
The list of sensitive detector objects.
virtual ~Geant4ActionSD()
Default destructor.
void exception(const std::string &src, const std::string &msg)
Actors< Geant4Filter > m_filters
The list of sensitive detector filter objects.
Geant4ActionSD & detector() const
Access to the sensitive detector object.
virtual void clear(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked if the event was aborted.
virtual void clear()
G4VSensitiveDetector interface: Method invoked if the event was aborted.
void clear()
Clear the sequence list.
Geant4Context * m_context
Reference to the Geant4 context.
const char * volName(const G4StepPoint *p, const char *undefined="") const
Generic hit container class using Geant4HitWrapper objects.
virtual bool process(const G4Step *step, G4TouchableHistory *history)
G4VSensitiveDetector interface: Method for generating hit(s) using the information of G4Step object.
Geant4Sensitive(Geant4Context *context, const std::string &name, DetElement det, Detector &description)
Constructor. The sensitive detector element is identified by the detector name.
bool isValid() const
Check the validity of the object held by the handle.
void adopt(Geant4Sensitive *sensitive)
Add an actor responding to all callbacks. Sequence takes ownership.
static void increment(T *)
Increment count according to type information.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
static Geant4Mapping & instance()
Possibility to define a singleton instance.
virtual ~Geant4Filter()
Standard destructor.
const char * name() const
Access the object name (or "" if not supported by the object)
Geant4SensDetSequences & sensitiveActions() const
Access to the sensitive detector sequences from the kernel object.
const G4ThreeVector & prePosG4() const
Returns the pre-step position as a G4ThreeVector.
Spot definition for fast simulation and GFlash.
long long int volumeID(const G4Step *step)
Returns the volumeID of the sensitive volume corresponding to the step.
Geant4HitCollection * collection(std::size_t which)
Retrieve the hits collection associated with this detector by its serial number.
virtual ~Geant4Sensitive()
Standard destructor.
CallbackSequence m_begin
Callback sequence for event initialization action.
Helper class to ease the extraction of information from a G4Step object.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4SensDetActionSequence(Geant4Context *context, const std::string &name)
Standard constructor.
void adoptFilter_front(Geant4Action *filter)
Add an actor responding to all callbacks to the sequence front. Sequence takes ownership.
VolumeID volumeID(const G4VTouchable *touchable) const
Access CELLID by placement path.
CallbackSequence m_process
Callback sequence for step processing.
virtual ~Geant4SensDetSequences()
Default destructor.
Actors< Geant4Filter > m_filters
The list of sensitive detector filter objects.
Geant4SensDetActionSequence * find(const std::string &name) const
Access sequence member by name.
Handle class describing a detector element.
void mark(const G4Track *track) const
Mark the track to be kept for MC truth propagation during hit processing.
virtual G4int GetCollectionID(G4int i)=0
This is a utility method which returns the hits collection ID.
void error(const char *fmt,...) const
Support of error messages.
Readout m_readout
Reference to the readout structure.
void setDetector(Geant4ActionSD *sens_det)
Access to the sensitive detector object.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
G4HCofThisEvent * m_hce
Geant4 hit collection context.
Detector & m_detDesc
Reference to the detector description object.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
bool accept(const G4Step *step) const
Callback before hit processing starts. Invoke all filters.
virtual void end(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the end of each event.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
Default Interface class to handle monte carlo truth records.
Geant4ActionSD * m_detector
Reference to G4 sensitive detector.
Geant4HitCollection * collection(std::size_t which) const
Retrieve the hits collection associated with this detector by its serial number.
int m_hitCreationMode
Property: Hit creation mode. Maybe one of the enum HitCreationFlags.
virtual void begin(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the begining of each event.
bool accept(const G4Step *step) const
Callback before hit processing starts. Invoke all filters.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
virtual ~Geant4SensDetActionSequence()
Default destructor.
The Geant4VolumeManager to facilitate optimized lookups of cell IDs from touchables.
Segmentation segmentation() const
Access segmentation structure.
CellID cellID(const Position &localPosition, const Position &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the local position
const std::string & name() const
Access name of the action.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
Geant4VolumeManager volumeManager() const
Access the volume manager.
std::string m_sensitiveType
The true sensitive type of the detector.
Geant4Filter(Geant4Context *context, const std::string &name)
Standard constructor.
HitCollections m_collections
Hit collection creators.
Interface class to access properties of the underlying Geant4 sensitive detector structure.
const G4ThreeVector & postPosG4() const
Returns the post-step position as a G4ThreeVector.
const std::string & hitCollectionName(std::size_t which) const
Access HitCollection container names.
std::string type() const
Access the type of the sensitive detector.
SensitiveDetector m_sensitive
Reference to the sensitive detector element.
ROOT::Math::XYZVector Position
dd4hep::DDSegmentation::VolumeID VolumeID
long addRef()
Increase reference count.
Geant4HitCollection * collectionByID(std::size_t id) const
Retrieve the hits collection associated with this detector by its collection identifier.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
std::pair< std::string, std::pair< Geant4Sensitive *, create_t > > HitCollection
Geant4Kernel & kernel() const
Access to the kernel object.
const char * c_name() const
Access name of the action.
The sequencer to host Geant4 sensitive actions called if particles interact with sensitive elements.
long long int cellID(const G4Step *step)
Returns the cellID of the sensitive volume corresponding to the step.
DetElement m_detector
Reference to the detector element describing this sensitive element.
void adoptFilter(Geant4Action *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
void adoptFilter(Geant4Action *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history)
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
Readout readout() const
Access readout structure of the sensitive detector.
const std::string & hitCollectionName(std::size_t which) const
Access HitCollection container names.
std::size_t defineCollection(Geant4Sensitive *owner, const std::string &name, create_t func)
Initialize the usage of a hit collection. Returns the collection identifier.
Base class to construct filters for Geant4 sensitive detectors.
std::string sdName(const G4StepPoint *p, const std::string &undefined="") const
The main interface to the dd4hep detector description package.
The base class for Geant4 sensitive detector actions implemented by users.
void adopt_front(Geant4Filter *filter)
Add an actor responding to all callbacks to the sequence front. Sequence takes ownership.
virtual void begin(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the begining of each event.
Segmentation m_segmentation
Reference to segmentation.
Geant4SensDetActionSequence * operator[](const std::string &name) const
Access sequence member by name.
virtual void mark(const G4Track *track)=0
Mark a Geant4 track to be kept for later MC truth analysis.
const G4VTouchable * preTouchable() const
Geant4ActionSD(const std::string &name)
Standard action constructor.
Geant4ActionSD * m_sensitiveDetector
Reference to G4 sensitive detector.
Geant4HitCollection * collectionByID(std::size_t id)
Retrieve the hits collection associated with this detector by its collection identifier.
virtual void end(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the end of each event.
Geant4SensDetActionSequence & sequence() const
Access to the hosting sequence.
CallbackSequence m_clear
Callback sequence to invoke the event deletion.
Generic context to extend user, run and event information.
virtual bool process(const G4Step *step, G4TouchableHistory *history)
G4VSensitiveDetector interface: Method for generating hit(s) using the information of G4Step object.
virtual std::size_t defineCollection(const std::string &name)=0
Initialize the usage of a hit collection. Returns the collection identifier.
Geant4Context * context() const
Access the context.
virtual bool operator()(const G4Step *step) const
Filter action. Return true if hits should be processed. Default returns true.
Detector & detectorDescription() const
Access the detector desciption object.