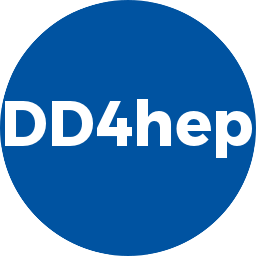 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4HITCOLLECTION_H
14 #define DDG4_GEANT4HITCOLLECTION_H
19 #include <G4VHitsCollection.hh>
36 class Geant4Sensitive;
37 class Geant4HitCollection;
38 class Geant4HitWrapper;
67 typedef std::pair<void*, HitManipulator*>
Wrapper;
69 const ComponentCast*
cast;
82 ComponentCast& me = ComponentCast::instance<TYPE>();
88 wrap.first = me.apply_dynCast(
cast, obj);
91 throw std::runtime_error(
"Invalid hit pointer passed to collection!");
95 TYPE* p = (TYPE*) obj.first;
101 static HitManipulator hm(ComponentCast::instance<TYPE>(), ComponentCast::instance<std::vector<TYPE*> >());
115 m_data.second = manipulator<InvalidHit>();
131 void *
operator new(size_t);
133 void operator delete(
void *ptr);
152 return HitManipulator::instance<TYPE>();
164 template <
typename TYPE>
operator TYPE*()
const {
165 return (TYPE*)
m_data.second->
166 cast.apply_dynCast(ComponentCast::instance<TYPE>(),
m_data.first);
209 typedef std::map<VolumeID, size_t>
Keys;
261 void releaseData(
const ComponentCast& cast, std::vector<void*>* result);
263 void getData(
const ComponentCast& cast, std::vector<void*>* result);
275 template <
typename TYPE>
286 template <
typename TYPE>
299 const ComponentCast&
type()
const;
303 virtual void clear();
333 template <
typename TYPE>
void add(TYPE* hit_pointer) {
347 throw std::runtime_error(
"Attempt to insert hit with same key to G4 hit-collection "+
GetName());
356 if ( i ==
m_keys.end() )
return 0;
363 std::vector<TYPE*> vec;
365 releaseData(ComponentCast::instance<TYPE>(), (std::vector<void*>*) &vec);
375 template <
typename TYPE> std::vector<TYPE*>
getHits() {
376 std::vector<TYPE*> vec;
378 getData(ComponentCast::instance<TYPE>(), (std::vector<void*>*) &vec);
404 template <
typename TYPE,
typename POS>
407 return pos == h->position ? h : 0;
427 template <
typename TYPE>
430 if (
id == h->cellID )
438 #endif // DDG4_GEANT4HITCOLLECTION_H
size_t m_lastHit
Memorize for speedup the last searched hit.
void * data() const
Pointer/Object access (CONST)
virtual size_t GetSize() const override
Access the collection size.
Union defining the hit collection flags for processing.
Geant4HitWrapper::HitManipulator Manip
Hit manipulator.
Generic class template to compare/select hits in Geant4HitCollection objects.
static HitManipulator * instance()
Geant4HitWrapper()
Default constructor.
virtual void clear()
Clear the collection (Deletes all valid references to real hits)
HitManipulator(const ComponentCast &c, const ComponentCast &v)
Initializing Constructor.
std::pair< void *, HitManipulator * > Wrapper
std::vector< Geant4HitWrapper > WrappedHits
Hit wrapper.
void * release()
Pointer/Object release.
Generic hit container class using Geant4HitWrapper objects.
TYPE * find(const Compare &cmp)
Find hits in a collection by comparison of attributes.
Geant4HitWrapper & hit(size_t which)
Access the hit wrapper.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
std::map< VolumeID, size_t > Keys
Hit key map for fast random lookup.
void getHitsUnchecked(std::vector< void * > &result)
Release all hits from the Geant4 container. Ownership stays with the container.
void * findHit(const Compare &cmp)
Find hit in a collection by comparison of attributes.
CellIDCompare(long long int i)
Constructor.
void add(VolumeID key, TYPE *hit_pointer)
Add a new hit with a check, that the hit is of the same type.
void * data()
Pointer/Object access.
static HitManipulator * manipulator()
Generate manipulator object.
Specialized hit selector based on the hit's position.
Geant4HitCollection(const std::string &det, const std::string &coll, Geant4Sensitive *sd, const TYPE *)
Initializing constructor.
virtual ~Geant4HitWrapper()
Default destructor.
Generic wrapper class for hit structures created in Geant4 sensitive detectors.
Geant4Sensitive * sensitive() const
Access the sensitive detector.
HitManipulator::Wrapper Wrapper
const char * GetName(T *p)
const ComponentCast & vector_type() const
Type information of the vector type for extracting data.
virtual ~Compare()
Default destructor.
TYPE * findByKey(VolumeID key)
Find hits in a collection by comparison of key value.
CollectionFlags m_flags
Optimization flags.
Geant4HitWrapper * findHitByKey(VolumeID key)
Find hit in a collection by comparison of the key.
std::vector< TYPE * > getHits()
Release all hits from the Geant4 container. Ownership stays with the container.
Wrapper releaseData()
Release data for copy.
void releaseHitsUnchecked(std::vector< void * > &result)
Release all hits from the Geant4 container and pass ownership to the caller.
void newInstance()
Notification to increase the instance counter.
Individual hit collection bits.
@ OPTIMIZE_REPEATEDLOOKUP
Manip * m_manipulator
The type of the objects in this collection. Set by the constructor.
WrappedHits m_hits
The collection of hit pointers in the wrapped format.
OptimizationFlags
Enumeration for collection optimization types.
const ComponentCast & type() const
Type information of the object stored.
const ComponentCast & vec_type
PositionCompare(const POS &p)
Constructor.
virtual ~Geant4HitCollection()
Default destructor.
Geant4HitWrapper(const Wrapper &v)
Copy constructor.
dd4hep::DDSegmentation::VolumeID VolumeID
Geant4Sensitive * m_detector
Handle to the sensitive detector.
const Geant4HitWrapper & hit(size_t which) const
Access the hit wrapper (CONST)
Specialized hit selector based on the hit's cell identifier.
Wrapper castHit(TYPE *obj)
Check pointer to be of proper type.
const ComponentCast & cast
Generic type manipulation class for generic hit structures created in Geant4 sensitive detectors.
static void deleteHit(Wrapper &obj)
Static function to delete contained hits.
virtual void * operator()(const Geant4HitWrapper &w) const
Comparison function.
Keys m_keys
Hit key map for fast random lookup.
Namespace for the AIDA detector description toolkit.
Helper class to indicate invalid hit wrappers or containers.
Geant4HitWrapper & operator=(const Geant4HitWrapper &v)
Assignment transfers the pointer ownership.
void releaseData(const ComponentCast &cast, std::vector< void * > *result)
Release all hits from the Geant4 container and pass ownership to the caller.
void setOptimize(int flag)
Set optimization flags.
virtual void * operator()(const Geant4HitWrapper &w) const
Comparison function.
HitManipulator * manip() const
Access to cast grammar.
void add(TYPE *hit_pointer)
Add a new hit with a check, that the hit is of the same type.
Geant4HitWrapper(const Geant4HitWrapper &v)
Copy constructor.
~HitManipulator()
Default destructor.
The base class for Geant4 sensitive detector actions implemented by users.
unsigned long value
Full value.
virtual void * operator()(const Geant4HitWrapper &w) const =0
Comparison function.
void setSensitive(Geant4Sensitive *detector)
Set the sensitive detector.
void getData(const ComponentCast &cast, std::vector< void * > *result)
Release all hits from the Geant4 container. Ownership stays with the container.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
struct dd4hep::sim::Geant4HitCollection::CollectionFlags::BitItems bits
std::vector< TYPE * > releaseHits()
Release all hits from the Geant4 container and pass ownership to the caller.
virtual G4VHit * GetHit(size_t which) const override
Access individual hits.
Wrapper m_data
Wrapper data.
Geant4HitCollection(const std::string &det, const std::string &coll, Geant4Sensitive *sd)
Initializing constructor (C++ version)