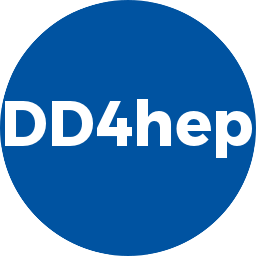 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4SENSDETACTION_H
14 #define DDG4_GEANT4SENSDETACTION_H
22 #include <G4ThreeVector.hh>
23 #include <G4VTouchable.hh>
29 class G4HCofThisEvent;
32 class G4TouchableHistory;
34 class G4VReadOutGeometry;
42 class SensitiveDetector;
48 class Geant4Sensitive;
49 class Geant4FastSimSpot;
50 class Geant4StepHandler;
51 class Geant4HitCollection;
52 class Geant4SensDetActionSequence;
53 class Geant4SensDetSequences;
81 virtual std::string
path()
const = 0;
106 virtual bool operator()(
const G4Step* step)
const;
138 #if defined(G__ROOT) || defined(__CLING__) || defined(__ROOTCLING__)
211 void mark(
const G4Track* track)
const;
214 void mark(
const G4Step* step)
const;
234 bool accept(
const G4Step* step)
const;
242 template <
typename TYPE> std::size_t
defineCollection(
const std::string& coll_name);
260 virtual void begin(G4HCofThisEvent* hce);
263 virtual void end(G4HCofThisEvent* hce);
271 virtual void clear(G4HCofThisEvent* hce);
277 long long int volumeID(
const G4Step* step);
283 long long int volumeID(
const G4VTouchable* touchable);
290 long long int cellID(
const G4Step* step);
297 long long int cellID(
const G4VTouchable* touchable,
const G4ThreeVector& global);
300 virtual bool process(
const G4Step* step, G4TouchableHistory* history);
320 typedef std::pair<std::string, std::pair<Geant4Sensitive*,create_t> >
HitCollection;
348 template <
typename TYPE>
static
380 return defineCollection(owner, coll_name, Geant4SensDetActionSequence::_create<TYPE>);
393 template <
typename T>
void callAtBegin(T* p,
void (T::*f)(G4HCofThisEvent*)) {
398 template <
typename T>
void callAtEnd(T* p,
void (T::*f)(G4HCofThisEvent*)) {
403 template <
typename T>
void callAtProcess(T* p,
void (T::*f)(G4Step*, G4TouchableHistory*)) {
408 template <
typename T>
void callAtClear(T* p,
void (T::*f)(G4HCofThisEvent*)) {
422 bool accept(
const G4Step* step)
const;
431 virtual void begin(G4HCofThisEvent* hce);
435 virtual void end(G4HCofThisEvent* hce);
442 virtual void clear();
445 virtual bool process(
const G4Step* step, G4TouchableHistory* history);
468 typedef std::map<std::string, Geant4SensDetActionSequence*>
Members;
535 const std::string&
name,
565 template <
typename HIT>
574 virtual
void begin(G4HCofThisEvent* hce) final;
576 virtual
void end(G4HCofThisEvent* hce) final;
578 virtual
void clear(G4HCofThisEvent* hce) final;
581 virtual
bool process(const G4Step* step,G4TouchableHistory* history) final;
589 #endif // DDG4_GEANT4SENSDETACTION_H
virtual void begin(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked at the begining of each event.
Geant4SensDetActionSequence * m_sequence
Reference to the containing action sequence.
SensitiveDetector m_sensitive
Reference to the sensitive detector element.
std::string fullPath() const
G4VSensitiveDetector internals: Access to the detector path name.
static Geant4HitCollection * _create(const std::string &det, const std::string &coll, Geant4Sensitive *sd)
Create a new typed hit collection.
virtual std::string path() const =0
G4VSensitiveDetector internals: Access to the detector path name.
virtual void defineCollections()
Define collections created by this sensitivie action object.
void callAtProcess(T *p, void(T::*f)(G4Step *, G4TouchableHistory *))
Register process-hit callback.
void adopt(Geant4Filter *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual void defineCollections()
Define collections created by this sensitivie action object.
void callAtBegin(T *p, void(T::*f)(G4HCofThisEvent *))
Register begin-of-event callback.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4Filter)
Define standard assignments and constructors.
G4VReadOutGeometry * readoutGeometry() const
Access to the readout geometry of the sensitive detector.
void insert(const std::string &name, Geant4SensDetActionSequence *seq)
Insert sequence member.
bool isActive() const
G4VSensitiveDetector internals: Is the detector active?
CallbackSequence m_end
Callback sequence for event finalization action.
Handle class to hold the information of a sensitive detector.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history)
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
virtual bool isActive() const =0
Is the detector active?
std::size_t m_collectionID
Collection identifier.
Actors< Geant4Sensitive > m_actors
The list of sensitive detector objects.
virtual ~Geant4ActionSD()
Default destructor.
virtual std::string fullPath() const =0
G4VSensitiveDetector internals: Access to the detector path name.
Actors< Geant4Filter > m_filters
The list of sensitive detector filter objects.
Geant4ActionSD & detector() const
Access to the sensitive detector object.
virtual void clear(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked if the event was aborted.
virtual void clear()
G4VSensitiveDetector interface: Method invoked if the event was aborted.
void clear()
Clear the sequence list.
virtual void initialize() final
Initialization overload for specialization.
Generic hit container class using Geant4HitWrapper objects.
virtual const std::string & sensitiveType() const
Access to the sensitive type of the detector.
virtual bool process(const G4Step *step, G4TouchableHistory *history)
G4VSensitiveDetector interface: Method for generating hit(s) using the information of G4Step object.
Geant4Sensitive(Geant4Context *context, const std::string &name, DetElement det, Detector &description)
Constructor. The sensitive detector element is identified by the detector name.
std::size_t defineCollection(Geant4Sensitive *owner, const std::string &coll_name)
Define a named collection containing hist of a specified type.
void add(const Callback &cb, Location where)
Generically Add a new callback to the sequence depending on the location arguments.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
void adopt(Geant4Sensitive *sensitive)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual ~Geant4Filter()
Standard destructor.
std::string m_collectionName
Property: collection name. If not set default is readout name!
std::size_t defineCollections(Geant4ActionSD *sens_det)
Called at construction time of the sensitive detector to declare all hit collections.
Spot definition for fast simulation and GFlash.
std::map< std::string, Geant4SensDetActionSequence * > Members
long long int volumeID(const G4Step *step)
Returns the volumeID of the sensitive volume corresponding to the step.
std::vector< HitCollection > HitCollections
virtual void end(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked at the end of each event.
Geant4HitCollection * collection(std::size_t which)
Retrieve the hits collection associated with this detector by its serial number.
virtual ~Geant4Sensitive()
Standard destructor.
virtual bool process(const G4Step *step, G4TouchableHistory *history) final
G4VSensitiveDetector interface: Method for generating hit(s) using the G4Step object.
CallbackSequence m_begin
Callback sequence for event initialization action.
virtual G4VReadOutGeometry * readoutGeometry() const =0
Access to the readout geometry of the sensitive detector.
virtual void finalize() final
Finalization overload for specialization.
virtual void clear(G4HCofThisEvent *hce) final
G4VSensitiveDetector interface: Method invoked if the event was aborted.
std::size_t defineReadoutCollection(const std::string collection_name)
Define readout specific hit collection. matching name must be present in readout structure.
Definition of an actor on sequences of callbacks.
Geant4SensDetActionSequence(Geant4Context *context, const std::string &name)
Standard constructor.
void adoptFilter_front(Geant4Action *filter)
Add an actor responding to all callbacks to the sequence front. Sequence takes ownership.
CallbackSequence m_process
Callback sequence for step processing.
virtual ~Geant4SensDetSequences()
Default destructor.
Actors< Geant4Filter > m_filters
The list of sensitive detector filter objects.
Geant4SensDetActionSequence * find(const std::string &name) const
Access sequence member by name.
Handle class describing a detector element.
void mark(const G4Track *track) const
Mark the track to be kept for MC truth propagation during hit processing.
virtual G4int GetCollectionID(G4int i)=0
This is a utility method which returns the hits collection ID.
UserData m_userData
User data block.
std::size_t defineCollection(const std::string &coll_name)
Initialize the usage of a single hit collection. Returns the collection ID.
Readout m_readout
Reference to the readout structure.
void setDetector(Geant4ActionSD *sens_det)
Access to the sensitive detector object.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4Sensitive)
Define standard assignments and constructors.
G4HCofThisEvent * m_hce
Geant4 hit collection context.
void callAtEnd(T *p, void(T::*f)(G4HCofThisEvent *))
Register end-of-event callback.
Detector & m_detDesc
Reference to the detector description object.
bool accept(const G4Step *step) const
Callback before hit processing starts. Invoke all filters.
virtual void end(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the end of each event.
SensitiveDetector sensitiveDetector() const
Access the dd4hep sensitive detector.
Geant4SensitiveAction(Geant4Context *context, const std::string &name, DetElement det, Detector &description)
Standard , initializing constructor.
Geant4ActionSD * m_detector
Reference to G4 sensitive detector.
Geant4HitCollection * collection(std::size_t which) const
Retrieve the hits collection associated with this detector by its serial number.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4ActionSD)
Define standard assignments and constructors.
int m_hitCreationMode
Property: Hit creation mode. Maybe one of the enum HitCreationFlags.
Geant4SensDetSequences: class to access groups of sensitive actions.
virtual void begin(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the begining of each event.
bool accept(const G4Step *step) const
Callback before hit processing starts. Invoke all filters.
virtual const std::string & sensitiveType() const =0
Access to the sensitive type of the detector.
void callAtClear(T *p, void(T::*f)(G4HCofThisEvent *))
Register clear callback.
Template class to ease the construction of sensitive detectors using particle template specialization...
Default base class for all Geant 4 actions and derivates thereof.
Geant4HitCollection *(* create_t)(const std::string &, const std::string &, Geant4Sensitive *)
virtual ~Geant4SensDetActionSequence()
Default destructor.
std::string detectorName() const
G4VSensitiveDetector internals: Access to the detector name.
Readout readout()
Access the readout object. Note: if m_readoutName is set, the m_readout != m_sensitive....
const std::string & name() const
Access name of the action.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
std::string m_sensitiveType
The true sensitive type of the detector.
Geant4Filter(Geant4Context *context, const std::string &name)
Standard constructor.
HitCollections m_collections
Hit collection creators.
Interface class to access properties of the underlying Geant4 sensitive detector structure.
const std::string & hitCollectionName(std::size_t which) const
Access HitCollection container names.
SensitiveDetector m_sensitive
Reference to the sensitive detector element.
std::size_t declareReadoutFilteredCollection()
Define readout specific hit collection with volume ID filtering.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SensDetActionSequence)
Define standard assignments and constructors.
void declareOptionalProperties()
Declare optional properties from embedded structure.
Geant4HitCollection * collectionByID(std::size_t id) const
Retrieve the hits collection associated with this detector by its collection identifier.
std::pair< std::string, std::pair< Geant4Sensitive *, create_t > > HitCollection
The sequencer to host Geant4 sensitive actions called if particles interact with sensitive elements.
Members & sequences()
Access to the members.
Class, which allows all Geant4Action to be stored.
long long int cellID(const G4Step *step)
Returns the cellID of the sensitive volume corresponding to the step.
DetElement m_detector
Reference to the detector element describing this sensitive element.
void adoptFilter(Geant4Action *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
void adoptFilter(Geant4Action *filter)
Add an actor responding to all callbacks. Sequence takes ownership.
int hitCreationMode() const
Property access to the hit creation mode.
const Members & sequences() const
Access to the members CONST.
virtual ~Geant4SensitiveAction()
Default destructor.
Namespace for the AIDA detector description toolkit.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history)
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
const std::string & hitCollectionName(std::size_t which) const
Access HitCollection container names.
Geant4SensDetSequences()=default
Default constructor.
std::size_t defineCollection(Geant4Sensitive *owner, const std::string &name, create_t func)
Initialize the usage of a hit collection. Returns the collection identifier.
virtual SensitiveDetector sensitiveDetector() const =0
Access to the Detector sensitive detector handle.
Base class to construct filters for Geant4 sensitive detectors.
The main interface to the dd4hep detector description package.
Actor class to manipulate action groups.
Handle to the implementation of the readout structure of a subdetector.
Handle class supporting generic Segmentations of sensitive detectors.
The base class for Geant4 sensitive detector actions implemented by users.
void adopt_front(Geant4Filter *filter)
Add an actor responding to all callbacks to the sequence front. Sequence takes ownership.
virtual void begin(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the begining of each event.
Segmentation m_segmentation
Reference to segmentation.
Geant4SensDetActionSequence * operator[](const std::string &name) const
Access sequence member by name.
Geant4ActionSD(const std::string &name)
Standard action constructor.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SensitiveAction)
Define standard assignments and constructors.
Geant4ActionSD * m_sensitiveDetector
Reference to G4 sensitive detector.
virtual bool processFastSim(const Geant4FastSimSpot *spot, G4TouchableHistory *history) final
GFLASH/FastSim interface: Method for generating hit(s) using the information of the fast simulation s...
Geant4HitCollection * collectionByID(std::size_t id)
Retrieve the hits collection associated with this detector by its collection identifier.
virtual void end(G4HCofThisEvent *hce)
G4VSensitiveDetector interface: Method invoked at the end of each event.
Geant4SensDetActionSequence & sequence() const
Access to the hosting sequence.
std::string path() const
G4VSensitiveDetector internals: Access to the detector path name.
CallbackSequence m_clear
Callback sequence to invoke the event deletion.
Generic context to extend user, run and event information.
virtual bool process(const G4Step *step, G4TouchableHistory *history)
G4VSensitiveDetector interface: Method for generating hit(s) using the information of G4Step object.
virtual std::size_t defineCollection(const std::string &name)=0
Initialize the usage of a hit collection. Returns the collection identifier.
Geant4Context * context() const
Access the context.
virtual bool operator()(const G4Step *step) const
Filter action. Return true if hits should be processed. Default returns true.
Detector & detectorDescription() const
Access the detector desciption object.
std::string m_readoutName
Property: segmentation name for parallel readouts. If not set readout segmentation is used!