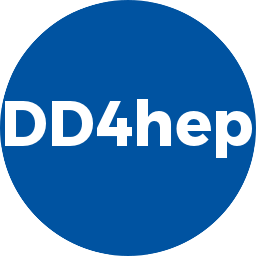 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4VOLUMEMANAGER_H
14 #define DDG4_GEANT4VOLUMEMANAGER_H
21 #include <G4VTouchable.hh>
23 class G4VPhysicalVolume;
33 class Geant4VolumeManager;
34 class Geant4GeometryInfo;
69 std::vector<const G4VPhysicalVolume*>
77 std::pair<
VolumeID,std::vector<std::pair<const BitFieldElement*, VolumeID> > >& volume_desc)
const;
80 std::pair<
VolumeID,std::vector<std::pair<const BitFieldElement*, VolumeID> > >& volume_desc)
const;
85 #endif // DDG4_GEANT4VOLUMEMANAGER_H
Geant4VolumeManager()=default
Default constructor.
std::size_t info(const std::string &src, const std::string &msg)
void exception(const std::string &src, const std::string &msg)
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Geant4VolumeManager(const Geant4VolumeManager &e)=default
Constructor to be used when reading the already parsed object.
static const VolumeID InvalidPath
static const VolumeID Insensitive
VolumeID volumeID(const G4VTouchable *touchable) const
Access CELLID by placement path.
Geant4VolumeManager & operator=(const Geant4VolumeManager &c)=default
Assignment operator.
static const VolumeID NonExisting
The Geant4VolumeManager to facilitate optimized lookups of cell IDs from touchables.
std::vector< const G4VPhysicalVolume * > placementPath(const G4VTouchable *touchable, bool exception=true) const
Helper: Generate placement path from touchable object.
Geant4VolumeManager(const Handle< Q > &e)
Constructor to be used when reading the already parsed object.
dd4hep::DDSegmentation::VolumeID VolumeID
Concreate class holding the relation information between geant4 objects and dd4hep objects.
Namespace for the AIDA detector description toolkit.
Geant4VolumeManager(const Handle< Geant4GeometryInfo > &e)
Constructor to be used when reading the already parsed object.
The main interface to the dd4hep detector description package.
void volumeDescriptor(const std::vector< const G4VPhysicalVolume * > &path, std::pair< VolumeID, std::vector< std::pair< const BitFieldElement *, VolumeID > > > &volume_desc) const
Accessfully decoded volume fields by placement path.
void volumeDescriptor(const G4VTouchable *touchable, std::pair< VolumeID, std::vector< std::pair< const BitFieldElement *, VolumeID > > > &volume_desc) const
Access fully decoded volume fields by Geant4 touchable object.
bool checkValidity() const
Check the validity of the information before accessing it.