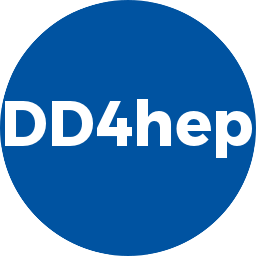 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
33 #include <G4Version.hh>
34 #include <G4UserRunAction.hh>
35 #include <G4UserEventAction.hh>
36 #include <G4UserTrackingAction.hh>
37 #include <G4UserStackingAction.hh>
38 #include <G4UserSteppingAction.hh>
39 #include <G4VUserPhysicsList.hh>
40 #include <G4VModularPhysicsList.hh>
41 #include <G4VUserPrimaryGeneratorAction.hh>
42 #include <G4VUserActionInitialization.hh>
43 #include <G4VUserDetectorConstruction.hh>
50 G4Mutex action_mutex=G4MUTEX_INITIALIZER;
126 detail::deletePtr(r);
139 detail::deletePtr(e);
266 return this->G4UserStackingAction::ClassifyNewTrack(track);
317 (*m_sequence)(s, fpSteppingManager);
329 public SequenceHdl<Geant4DetectorConstructionSequence>
346 virtual G4VPhysicalVolume*
Construct() final;
368 virtual void Build() const final;
370 virtual
void BuildForMaster() const final;
405 (*m_sequence)(event);
408 throw std::runtime_error(
"GeneratePrimaries: Panic! No action sequencer defined. "
409 "No primary particles can be produced.");
414 G4AutoLock protection_lock(&action_mutex);
428 G4AutoLock protection_lock(&action_mutex);
432 m_sequence->
except(
"+++ Executing G4 detector construction did not result in a valid world volume!");
440 G4AutoLock protection_lock(&action_mutex);
446 m_sequence->
info(
"+++ Executing Geant4UserActionInitialization::Build. "
447 "Context:%p Kernel:%p [%ld]", (
void*)ctx, (
void*)&krnl, krnl.
id());
455 SetUserAction(gen_action);
459 SetUserAction(run_action);
465 SetUserAction(evt_action);
471 SetUserAction(action);
477 SetUserAction(action);
483 SetUserAction(action);
491 m_sequence->
info(
"+++ Executing Geant4UserActionInitialization::BuildForMaster....");
523 #include <G4RunManager.hh>
524 #include <G4PhysListFactory.hh>
532 printout(WARNING,
"Geant4Exec",
"+++ Building default Geant4DetectorConstruction for single threaded compatibility.");
535 cr = PluginService::Create<Geant4Action*>(
"Geant4DetectorGeometryConstruction",ctx,std::string(
"ConstructGeometry"));
540 throw std::runtime_error(
"Panic! Failed to build Geant4DetectorGeometryConstruction.");
542 cr = PluginService::Create<Geant4Action*>(
"Geant4DetectorSensitivesConstruction",ctx,std::string(
"ConstructSensitives"));
547 throw std::runtime_error(
"Panic! Failed to build Geant4DetectorSensitivesConstruction.");
566 G4RunManager& runManager = kernel.
runManager();
570 printout(WARNING,
"Geant4Exec",
"+++ Only %d subdetectors present. "
571 "You sure you loaded the geometry properly?",
578 throw std::runtime_error(
"Panic! No valid detector construction sequencer present. [Mandatory MT]");
584 runManager.SetUserInitialization(det_seq);
588 if (
nullptr == phys_seq ) {
589 std::string phys_model =
"QGSP_BERT";
594 if (
nullptr == physics) {
595 throw std::runtime_error(
"Panic! No valid user physics list present!");
598 printout(INFO,
"Geant4Exec",
"+++ PhysicsList RangeCut: %f", phys_seq->
m_rangecut );
600 physics->SetDefaultCutValue(phys_seq->
m_rangecut);
602 if( DEBUG == printLevel() ) physics->DumpCutValuesTable();
604 phys_seq->
enable(physics);
605 runManager.SetUserInitialization(physics);
610 throw std::runtime_error(
"Panic! No valid user initialization sequencer present. [Mandatory MT]");
617 runManager.SetUserInitialization(init);
624 G4RunManager& runManager = kernel.
runManager();
630 runManager.Initialize();
637 std::string value = p.
value<std::string>();
640 if ( !value.empty() ) {
649 ui->
except(
"++ Geant4Exec: Failed to start UI interface.");
651 throw std::runtime_error(format(
"Geant4Exec",
"++ Failed to locate UI interface %s.",value.c_str()));
Geant4Context * context() const
Access reference to the current active Geant4Context structure.
Geant4UserGeneratorAction(Geant4Context *ctxt, Geant4GeneratorActionSequence *seq)
Standard constructor.
virtual G4ClassificationOfNewTrack ClassifyNewTrack(const G4Track *track) override final
Geant4Compatibility()=default
Default constructor.
G4ClassificationOfNewTrack value
void setEvent(Geant4Event *new_event)
Set the geant4 event reference.
virtual void constructField(Geant4DetectorConstructionContext *ctxt)
Electromagnetic field construction callback. Called at "ConstructSDandField()".
Concrete implementation of the Geant4 event action.
virtual void end(const G4Track *track)
Post-tracking action callback.
void setWorld(G4VPhysicalVolume *volume)
Set the geometry world.
Callback interface class with argument.
The property class to assign options to actions.
static int configure(Geant4Kernel &kernel)
Configure the application.
User run context for DDG4.
static Geant4Random * instance(bool throw_exception=true)
Access the main Geant4 random generator instance. Must be created before used!
virtual void ConstructSDandField() final
Call the actions for the construction of the sensitive detectors and the field.
Class to orchestrate a modular initializion of a multi- or single threaded Geant4 application.
Concrete implementation of the Geant4 stacking action sequence.
Concrete implementation of the Geant4 stacking action sequence.
unsigned long id() const
Access worker identifier.
Geant4RunActionSequence * runAction(bool create)
Access run action sequence.
static int run(Geant4Kernel &kernel)
Run the application and simulate events.
G4VUserPhysicsList * extensionList()
Extend physics list from factory:
Concrete implementation of the Geant4 run action.
void setRun(Geant4Run *new_run)
Set the geant4 run reference.
virtual ~Geant4UserStackingAction()
Default destructor.
Geant4UserDetectorConstruction(Geant4Context *ctxt, Geant4DetectorConstructionSequence *seq)
Standard constructor.
virtual void BuildForMaster() const final
Build the action sequences for the master thread.
Geant4StackingActionSequence * stackingAction(bool create)
Access stacking action sequence.
Geant4UserStackingAction(Geant4Context *ctxt, Geant4StackingActionSequence *seq)
Standard constructor.
Property & property(const std::string &name)
Access single property.
virtual void PrepareNewEvent() override final
Preparation callback.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
virtual ~SequenceHdl()
Default destructor.
Geant4TrackingActionSequence * trackingAction(bool create)
Access tracking action sequence.
virtual bool executePhase(const std::string &name, const void **args) const
Execute phase action if it exists.
Property & property(const std::string &name)
Access single property.
Concrete implementation of the Geant4 tracking action.
virtual ~Geant4Compatibility()=default
Default destructor.
static void increment(T *)
Increment count according to type information.
virtual void PreUserTrackingAction(const G4Track *trk) final
Pre-track action callback.
void destroyClientContext(const G4Event *)
Destroy Geant4 event context.
virtual void begin(const G4Track *track)
Pre-tracking action callback.
virtual void EndOfEventAction(const G4Event *evt) final
End-of-event callback.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
virtual void Build() const final
Build the actions for the worker thread.
Geant4Context * workerContext()
Thread's Geant4 execution context.
virtual void UserSteppingAction(const G4Step *s) final
User stepping callback.
void createClientContext(const G4Run *run)
Create Geant4 run context.
Concrete implementation of the Geant4 tracking action sequence.
Detector & detectorDescription() const
Access to detector description.
Geant4Kernel & worker(unsigned long thread_identifier, bool create_if=false)
Access worker instance by its identifier.
G4VPhysicalVolume * world
Reference to the world after construction.
void info(const char *fmt,...) const
Support of info messages.
Concrete implementation of the Geant4 stepping action.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
double m_rangecut
global range cut for secondary productions
Concrete implementation of the Geant4 generator action sequence.
Geant4DetectorConstructionSequence * detectorConstruction(bool create)
Access detector construcion action sequence (geometry+sensitives+field)
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt)
Geometry construction callback. Called at "Construct()".
Geant4UserRunAction(Geant4Context *ctxt, Geant4RunActionSequence *seq)
Standard constructor.
virtual void BeginOfEventAction(const G4Event *evt) final
Begin-of-event callback.
Geant4UserSteppingAction(Geant4Context *ctxt, Geant4SteppingActionSequence *seq)
Standard constructor.
Geant4EventActionSequence * eventAction(bool create)
Access run action sequence.
Geant4UserRunAction * runAction
static Geant4Random * setMainInstance(Geant4Random *ptr)
Make this random generator instance the one used by Geant4.
Concrete implementation of the Geant4 generator action.
static void decrement(T *)
Decrement count according to type information.
Concrete basic implementation of the Geant4 run action sequencer.
Sequence handler implementing common actions to all sequences.
void destroyClientContext(const G4Run *)
Destroy Geant4 run context.
virtual void prepare(G4StackManager *stackManager)
Preparation callback.
virtual G4VPhysicalVolume * Construct() final
Call the actions to construct the detector geometry.
void adopt(Geant4DetectorConstruction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
The implementation of the single Geant4 physics list action sequence.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
static int terminate(Geant4Kernel &kernel)
Terminate the application.
virtual ~Geant4UserDetectorConstruction()
Default destructor.
virtual const HandleMap & sensitiveDetectors() const =0
Accessor to the map of sub-detectors.
virtual void PostUserTrackingAction(const G4Track *trk) final
Post-track action callback.
void configureFiber(Geant4Context *ctxt)
G4 callback in multi threaded mode to configure thread fiber.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Mini interface to THE random generator of the application.
Default base class for all Geant 4 actions and derivates thereof.
virtual void BeginOfRunAction(const G4Run *run) final
Begin-of-run callback.
static unsigned long int thread_self()
Access thread identifier.
void createClientContext(const G4Event *evt)
Create Geant4 event context.
Concrete basic implementation of the Geant4 detector construction sequencer.
virtual ~Geant4UserSteppingAction()
Default destructor.
SequenceHdl()
Default constructor.
Concrete implementation of the Geant4 user detector construction action sequence.
virtual void EndOfRunAction(const G4Run *run) final
End-of-run callback.
Geant4UserInitializationSequence * userInitialization(bool create)
Access to the user initialization object.
static int initialize(Geant4Kernel &kernel)
Initialize the application.
virtual ~Geant4UserRunAction()
Default destructor.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
bool isMultiThreaded() const
Geant4Action * globalAction(const std::string &action_name, bool throw_if_not_present=true)
Retrieve action from repository.
TYPE value() const
Retrieve value.
virtual ~Geant4UserGeneratorAction()
Default destructor.
Geant4Run * runPtr() const
Access the geant4 run by ptr. Must be checked by clients!
void set(const TYPE &value)
Set value of this property.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
SequenceHdl(Geant4Context *ctxt, T *seq)
Initializing constructor.
Geant4Kernel & kernel() const
Access to the kernel object.
Geant4SteppingActionSequence * steppingAction(bool create)
Access stepping action sequence.
Geant4UserEventAction(Geant4Context *ctxt, Geant4EventActionSequence *seq)
Standard constructor.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Geant4Kernel & kernel() const
Access reference to the current active Geant4Kernel structure.
virtual ~Geant4UserEventAction()
Default destructor.
Geant4PhysicsListActionSequence * physicsList(bool create)
Access to the physics list.
Namespace for the AIDA detector description toolkit.
Geant4Context * m_activeContext
void updateContext(Geant4Context *ctxt)
Update Geant4Context for current call.
void setTrackMgr(G4TrackingManager *mgr)
Access the tracking manager.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual void enable(G4VUserPhysicsList *physics)
Enable physics list: actions necessary to be propagated to Geant4.
Geant4Event * eventPtr() const
Access the geant4 event by ptr. Must be checked by clients!
The main interface to the dd4hep detector description package.
Concrete implementation of the Geant4 event action sequence.
Geant4DetectorConstructionContext m_ctxt
virtual void newStage(G4StackManager *stackManager)
New-stage callback.
Geant4DetectorConstructionSequence * buildDefaultDetectorConstruction(Geant4Kernel &kernel)
Detector construction invocation in compatibility mode.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Concrete implementation of the Geant4 user initialization action sequence.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
G4RunManager & runManager()
Access to the Geant4 run manager.
Compatibility actions for running Geant4 in single threaded mode.
Basic implementation of the Geant4 detector construction action.
virtual void GeneratePrimaries(G4Event *event) final
Generate primary particles.
Geant4UserActionInitialization(Geant4Context *ctxt, Geant4UserInitializationSequence *seq)
Standard constructor.
virtual ~Geant4UserTrackingAction()
Default destructor.
Concrete implementation of the Geant4 stepping action sequence.
Geant4UserTrackingAction(Geant4Context *ctxt, Geant4TrackingActionSequence *seq)
Standard constructor.
void initialize()
Initialize the instance.
virtual void NewStage() override final
New-stage callback.
User event context for DDG4.
virtual TrackClassification classifyNewTrack(G4StackManager *stackManager, const G4Track *track)
Classify new track: The first call in the sequence returning non-null pointer wins!
void _release()
Release object.
void applyInterruptHandlers()
(Re-)apply registered interrupt handlers to override potentially later registrations by other librari...
virtual ~Geant4UserActionInitialization()
Default destructor.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4GeneratorActionSequence * generatorAction(bool create)
Access generator action sequence.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
void _aquire(T *s)
Aquire object reference.
Geant4UserEventAction * eventAction