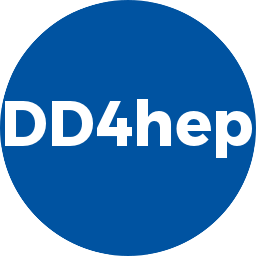 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4STEPPINGACTION_H
14 #define DDG4_GEANT4STEPPINGACTION_H
20 class G4SteppingManager;
30 class Geant4SteppingAction;
31 class Geant4SharedSteppingAction;
32 class Geant4SteppingActionSequence;
52 virtual void operator()(
const G4Step* step, G4SteppingManager* mgr);
86 virtual void operator()(
const G4Step* step, G4SteppingManager* mgr)
override;
126 template <
typename Q,
typename T>
127 void call(Q* p,
void (T::*f)(
const G4Step*, G4SteppingManager*)) {
133 virtual void operator()(
const G4Step* step, G4SteppingManager* mgr);
139 #endif // DDG4_GEANT4STEPPINGACTION_H
Geant4SharedSteppingAction shared_type
Concrete implementation of the Geant4 stepping action sequence.
virtual ~Geant4SteppingActionSequence()
Default destructor.
Geant4SteppingAction * get(const std::string &name) const
Get an action by name.
Geant4SteppingAction(Geant4Context *context, const std::string &name)
Standard constructor.
Actors< Geant4SteppingAction > m_actors
The list of action objects to be called.
virtual void operator()(const G4Step *step, G4SteppingManager *mgr) override
User stepping callback.
void add(const Callback &cb, Location where)
Generically Add a new callback to the sequence depending on the location arguments.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SteppingAction)
Define standard assignments and constructors.
virtual void operator()(const G4Step *step, G4SteppingManager *mgr)
User stepping callback.
Geant4SteppingActionSequence(Geant4Context *context, const std::string &name)
Standard constructor.
Geant4SharedSteppingAction(Geant4Context *context, const std::string &nam)
Standard constructor.
CallbackSequence m_calls
Callback sequence for user stepping action calls.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
Definition of an actor on sequences of callbacks.
virtual ~Geant4SharedSteppingAction()
Default destructor.
Implementation of the Geant4 shared stepping action.
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
void call(Q *p, void(T::*f)(const G4Step *, G4SteppingManager *))
Register stepping action callback. Types Q and T must be polymorph!
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
virtual void operator()(const G4Step *step, G4SteppingManager *mgr)
User stepping callback.
virtual ~Geant4SteppingAction()
Default destructor.
Namespace for the AIDA detector description toolkit.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SharedSteppingAction)
Define standard assignments and constructors.
Actor class to manipulate action groups.
virtual void use(Geant4SteppingAction *action)
Underlying object to be used during the execution of this thread.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SteppingActionSequence)
Define standard assignments and constructors.
void adopt(Geant4SteppingAction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
Concrete implementation of the Geant4 stepping action sequence.
Geant4SteppingAction * m_action
Reference to the shared action.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.