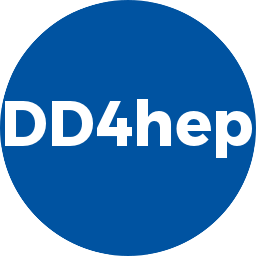 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <G4VUserDetectorConstruction.hh>
22 #include <G4SDManager.hh>
29 G4SDManager::GetSDMpointer()->AddNewDetector(sd);
30 vol->SetSensitiveDetector(sd);
46 const std::string& nam) {
50 print(
"+++ Subdetector: %-32s type: %s.", nam.c_str(), typ.c_str());
54 g4sd = PluginService::Create<G4VSensitiveDetector*>(typ, nam, &dsc);
56 except(
"createSensitiveDetector: FATAL Failed to "
57 "create Geant4 sensitive detector %s of type %s.",
58 nam.c_str(), typ.c_str());
60 print(
"+++ Subdetector: %-32s type: %s.", nam.c_str(), typ.c_str());
64 G4SDManager::GetSDMpointer()->AddNewDetector(g4sd);
108 except(
"++ Attempt to add an invalid actor!");
114 if ( i->name() == nam ) {
118 except(
"++ Attempt to access invalid actor %s!",nam.c_str());
138 const std::map<dd4hep::Region, G4Region*>&
142 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::regions: Access not possible. Geometry is not yet converted!");
146 const std::map<dd4hep::Volume, G4LogicalVolume*>&
150 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::volumes: Access not possible. Geometry is not yet converted!");
157 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::shapes: Access not possible. Geometry is not yet converted!");
161 const std::map<dd4hep::LimitSet, G4UserLimits*>&
165 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::limits: Access not possible. Geometry is not yet converted!");
169 const std::map<dd4hep::PlacedVolume, Geant4AssemblyVolume*>&
173 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::assemblies: Access not possible. Geometry is not yet converted!");
177 const std::map<dd4hep::PlacedVolume, G4VPhysicalVolume*>&
181 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::placements: Access not possible. Geometry is not yet converted!");
188 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::materials: Access not possible. Geometry is not yet converted!");
195 throw std::runtime_error(
"+++ Geant4DetectorConstructionSequence::elements: Access not possible. Geometry is not yet converted!");
virtual void updateContext(Geant4Context *ctxt)
Set or update client context.
bool m_needsControl
Default property: Flag to create control instance.
virtual void constructField(Geant4DetectorConstructionContext *ctxt)
Electromagnetic field construction callback. Called at "ConstructSDandField()".
Geant4GeometryMaps::AssemblyMap g4AssemblyVolumes
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
Detector & detectorDescription() const
Access to detector description.
std::map< Region, G4Region * > g4Regions
virtual G4VSensitiveDetector * createSensitiveDetector(const std::string &type, const std::string &name)
Create Geant4 sensitive detector object using the factory mechanism.
Geant4Context * m_context
Reference to the Geant4 context.
virtual ~Geant4DetectorConstructionSequence()
Default destructor.
Geant4GeometryMaps::MaterialMap g4Materials
const std::map< const TGeoShape *, G4VSolid * > & shapes() const
Access to the converted shapes.
static void increment(T *)
Increment count according to type information.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
Geant4DetectorConstruction(Geant4Context *context, const std::string &nam)
Standard Constructor.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt)
Geometry construction callback. Called at "Construct()".
Geant4GeometryInfo * ptr() const
Access to the data pointer.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Geant4GeometryMaps::PlacementMap g4Placements
static void decrement(T *)
Decrement count according to type information.
const std::map< Atom, G4Element * > & elements() const
Access to the converted elements.
void adopt(Geant4DetectorConstruction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
Helper to debug plugin manager calls.
const std::map< LimitSet, G4UserLimits * > & limits() const
Access to the converted limit sets.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
Actors< Geant4DetectorConstruction > m_actors
The list of action objects to be called.
std::map< Material, G4Material * > MaterialMap
std::map< Atom, G4Element * > ElementMap
virtual ~Geant4DetectorConstruction()
Default destructor.
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
std::map< LimitSet, G4UserLimits * > g4Limits
Geant4GeometryMaps::SolidMap g4Solids
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4DetectorConstruction * get(const std::string &nam) const
Access an actor by name.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt)
Geometry construction callback. Called at "Construct()".
void setSensitiveDetector(G4LogicalVolume *vol, G4VSensitiveDetector *sd)
Helper: Assign sensitive detector to logical volume.
const std::map< Material, G4Material * > & materials() const
Access to the converted materials.
virtual void constructField(Geant4DetectorConstructionContext *ctxt)
Electromagnetic field construction callback. Called at "ConstructSDandField()".
const std::map< Volume, G4LogicalVolume * > & volumes() const
Access to the converted volumes.
The main interface to the dd4hep detector description package.
const std::map< PlacedVolume, G4VPhysicalVolume * > & placements() const
Access to the converted placements.
Geant4GeometryMaps::ElementMap g4Elements
Basic implementation of the Geant4 detector construction action.
Geant4DetectorConstructionSequence(Geant4Context *context, const std::string &nam)
Standard Constructor.
const std::map< Region, G4Region * > & regions() const
Access to the converted regions.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4GeometryMaps::VolumeMap g4Volumes
const std::map< PlacedVolume, Geant4AssemblyVolume * > & assemblies() const
Access to the converted assemblys.