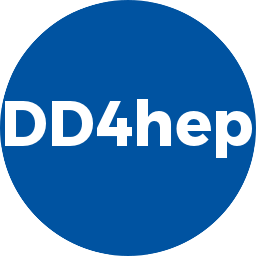 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4RANDOM_H
14 #define DDG4_GEANT4RANDOM_H
26 namespace CLHEP {
class HepRandomEngine; }
119 virtual void setSeed(
long seed);
125 virtual void setSeeds(
const long * seeds,
int size);
127 virtual void saveStatus(
const char filename[] =
"Config.conf")
const;
129 virtual void restoreStatus(
const char filename[] =
"Config.conf" );
142 double rndm(
int i=0);
150 double uniform(
double x1,
double x2);
153 double exp(
double tau);
155 double gauss(
double mean=0,
double sigma=1);
157 double gamma(
double k,
double lambda);
159 double landau(
double mean=0,
double sigma=1);
161 void circle(
double &x,
double &y,
double r);
163 void sphere(
double &x,
double &y,
double &z,
double r);
165 double poisson(
double mean=1e0 );
172 #endif // DDG4_GEANT4RANDOM_H
double poisson(double mean=1e0)
Create poisson distributed random numbers.
bool m_replace
Property: Indicator to replace the ROOT gRandom instance.
double rndm(int i=0)
Create flat distributed random numbers in the interval ]0,1].
static Geant4Random * instance(bool throw_exception=true)
Access the main Geant4 random generator instance. Must be created before used!
void sphere(double &x, double &y, double &z, double r)
Create tuple of randum number on a sphere with radius r.
CLHEP::HepRandomEngine * engine()
CLHEP random number engine (valid after initialization only)
double uniform(double x1=1)
Create uniformly disributed random numbers in the interval ]0,x1].
std::string m_file
Property: File name if initialized from file. If set, engine name and seeds are ignored.
double rndm_clhep()
Create flat distributed random numbers in the interval ]0,1] calling CLHEP.
virtual void setSeed(long seed)
Should initialise the status of the algorithm according to seed.
double gamma(double k, double lambda)
Create gamma distributed random numbers.
virtual void showStatus() const
Should dump the current engine status on the screen.
virtual ~Geant4Random()
Default destructor.
double breit_wigner(double mean=0e0, double gamma=1e0)
Create breit wigner distributed random numbers.
static Geant4Random * setMainInstance(Geant4Random *ptr)
Make this random generator instance the one used by Geant4.
Geant4Random(Geant4Context *context, const std::string &name)
Standard constructor.
Mini interface to THE random generator of the application.
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
void rndmArray(int n, float *array)
Create a float array of flat distributed random numbers in the interval ]0,1].
virtual void saveStatus(const char filename[]="Config.conf") const
Should save on a file specific to the instantiated engine in use the current status.
void circle(double &x, double &y, double r)
Create tuple of randum number around a circle with radius r.
double gauss(double mean=0, double sigma=1)
Create gaussian distributed random numbers.
TRandom * m_rootRandom
Reference to ROOT random instance.
Main executor steering the Geant4 execution.
CLHEP::HepRandomEngine * m_engine
Reference to the CLHEP random number engine (valid only after initialization)
virtual void setSeeds(const long *seeds, int size)
Should initialise the status of the algorithm.
Namespace for the AIDA detector description toolkit.
std::string m_engineType
Property: Engine type. default: "HepJamesRandom".
virtual void restoreStatus(const char filename[]="Config.conf")
Should read from a file and restore the last saved engine configuration.
long m_seed
Property: Initial random seed. Default: 123456789.
bool m_inited
Flag to remember initialization.
double exp(double tau)
Create exponentially distributed random numbers.
void initialize()
Initialize the instance.
double landau(double mean=0, double sigma=1)
Create landau distributed random numbers.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.