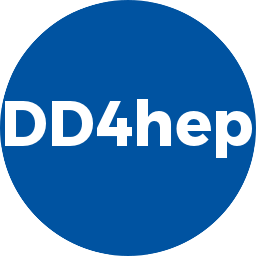 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4RUNACTION_H
15 #define DDG4_GEANT4RUNACTION_H
30 class Geant4RunAction;
31 class Geant4SharedRunAction;
32 class Geant4RunActionSequence;
59 virtual void begin(
const G4Run* run);
61 virtual void end(
const G4Run* run);
97 virtual void begin(
const G4Run* run)
override;
99 virtual void end(
const G4Run* run)
override;
143 template <
typename Q,
typename T>
148 template <
typename Q,
typename T>
155 virtual void begin(
const G4Run* run);
157 virtual void end(
const G4Run* run);
163 #endif // DDG4_GEANT4RUNACTION_H
void adopt(Geant4RunAction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
virtual ~Geant4SharedRunAction()
Default destructor.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4RunAction)
Define standard assignments and constructors.
Actors< Geant4RunAction > m_actors
The list of action objects to be called.
virtual void end(const G4Run *run)
End-of-run callback.
Geant4RunActionSequence(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void end(const G4Run *run) override
End-of-run callback.
void add(const Callback &cb, Location where)
Generically Add a new callback to the sequence depending on the location arguments.
void callAtBegin(Q *p, void(T::*f)(const G4Run *))
Register begin-of-run callback. Types Q and T must be polymorph!
virtual void begin(const G4Run *run)
Begin-of-run callback.
Implementation of the Geant4 shared run action.
void callAtEnd(Q *p, void(T::*f)(const G4Run *))
Register end-of-run callback. Types Q and T must be polymorph!
Definition of an actor on sequences of callbacks.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SharedRunAction)
Define standard assignments and constructors.
virtual ~Geant4RunActionSequence()
Default destructor.
virtual void begin(const G4Run *run) override
Begin-of-run callback.
virtual void end(const G4Run *run)
End-of-run callback.
Geant4SharedRunAction(Geant4Context *context, const std::string &nam)
Standard constructor.
Concrete basic implementation of the Geant4 run action sequencer.
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
virtual void begin(const G4Run *run)
Begin-of-run callback.
Geant4RunAction * m_action
Reference to the shared action.
virtual void use(Geant4RunAction *action)
Underlying object to be used during the execution of this thread.
CallbackSequence m_begin
Callback sequence for begin-run action.
Geant4SharedRunAction shared_type
Geant4RunAction * get(const std::string &name) const
Get an action by name.
Concrete basic implementation of the Geant4 run action base class.
Namespace for the AIDA detector description toolkit.
virtual ~Geant4RunAction()
Default destructor.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4RunActionSequence)
Define standard assignments and constructors.
Actor class to manipulate action groups.
CallbackSequence m_end
Callback sequence for end-run action.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
Geant4RunAction(Geant4Context *context, const std::string &nam)
Standard constructor.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.