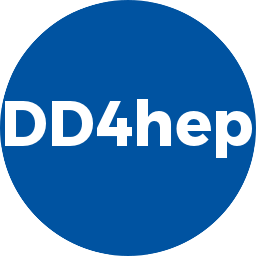 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4EVENTACTION_H
15 #define DDG4_GEANT4EVENTACTION_H
30 class Geant4EventAction;
31 class Geant4SharedEventAction;
32 class Geant4EventActionSequence;
67 virtual void begin(
const G4Event* event);
69 virtual void end(
const G4Event* event);
104 virtual void begin(
const G4Event* event)
override;
106 virtual void end(
const G4Event* event)
override;
151 template <
typename Q,
typename T>
156 template <
typename Q,
typename T>
161 template <
typename Q,
typename T>
168 virtual void begin(
const G4Event* event);
170 virtual void end(
const G4Event* event);
175 #endif // DDG4_GEANT4EVENTACTION_H
virtual void begin(const G4Event *event)
Begin-of-event callback.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4EventAction)
Define standard assignments and constructors.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
Geant4EventActionSequence(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
void add(const Callback &cb, Location where)
Generically Add a new callback to the sequence depending on the location arguments.
Geant4EventAction * m_action
Reference to the shared action.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4SharedEventAction)
Define standard assignments and constructors.
void callAtEnd(Q *p, void(T::*f)(const G4Event *))
Register end-of-event callback.
Actors< Geant4EventAction > m_actors
The list of action objects to be called.
Implementation of the Geant4 shared event action.
Concrete basic implementation of the Geant4 event action.
Definition of an actor on sequences of callbacks.
Geant4EventAction(Geant4Context *context, const std::string &nam)
Standard constructor.
void callAtBegin(Q *p, void(T::*f)(const G4Event *))
Register begin-of-event callback.
virtual void use(Geant4EventAction *action)
Underlying object to be used during the execution of this thread.
CallbackSequence m_begin
Callback sequence for event initialization action.
virtual void begin(const G4Event *event)
Begin-of-event callback.
void callAtFinal(Q *p, void(T::*f)(const G4Event *))
Register event-cleanup callback (after end-of-event callback – unordered)
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4EventActionSequence)
Define standard assignments and constructors.
Geant4SharedEventAction shared_type
CallbackSequence m_end
Callback sequence for event finalization action.
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
virtual ~Geant4EventAction()
Default destructor.
virtual ~Geant4SharedEventAction()
Default destructor.
virtual void end(const G4Event *event)
End-of-event callback.
Namespace for the AIDA detector description toolkit.
virtual void begin(const G4Event *event) override
Begin-of-event callback.
Geant4EventAction * get(const std::string &name) const
Get an action by name.
virtual void configureFiber(Geant4Context *thread_context) override
Set or update client for the use in a new thread fiber.
CallbackSequence m_final
Callback sequence for event finalization action.
Actor class to manipulate action groups.
virtual void end(const G4Event *event)
End-of-event callback.
Concrete implementation of the Geant4 event action sequence.
Geant4SharedEventAction(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual ~Geant4EventActionSequence()
Default destructor.
virtual void end(const G4Event *event) override
End-of-event callback.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
void adopt(Geant4EventAction *action)
Add an actor responding to all callbacks. Sequence takes ownership.