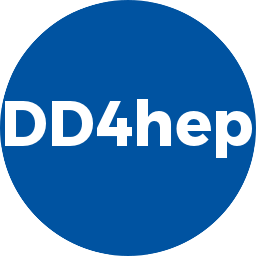 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <G4VPhysicsConstructor.hh>
25 #include <G4PhysListFactory.hh>
26 #include <G4ProcessManager.hh>
27 #include <G4ParticleTable.hh>
28 #include <G4RunManager.hh>
29 #include <G4VProcess.hh>
31 #include <G4EmParameters.hh>
32 #include <G4HadronicParameters.hh>
42 struct MyPhysics : G4VUserPhysicsList {
43 void AddTransportation() { this->G4VUserPhysicsList::AddTransportation(); }
46 struct EmptyPhysics :
public G4VModularPhysicsList {
47 EmptyPhysics() =
default;
48 virtual ~EmptyPhysics() =
default;
52 G4VUserPhysicsList* phys;
54 virtual ~ParticlePhysics() =
default;
55 virtual void ConstructProcess() {
58 MyPhysics* ph = (MyPhysics*)phys;
59 ph->AddTransportation();
62 virtual void ConstructParticle() {
70 : ordAtRestDoIt(-1), ordAlongSteptDoIt(-1), ordPostStepDoIt(-1) {
74 :
name(p.
name), ordAtRestDoIt(p.ordAtRestDoIt), ordAlongSteptDoIt(p.ordAlongSteptDoIt), ordPostStepDoIt(p.ordPostStepDoIt) {
107 printout(ALWAYS,
name(),
"+++ Geant4PhysicsList Dump");
110 printout(ALWAYS,
name(),
"+++ PhysicsConstructor: %s",p.c_str());
112 printout(ALWAYS,
name(),
"+++ ParticleConstructor: %s",p.c_str());
114 printout(ALWAYS,
name(),
"+++ ParticleGroupConstructor: %s",p.c_str());
116 printout(ALWAYS,
name(),
"+++ DiscretePhysicsProcesses of particle %s",part_name.c_str());
117 for (ParticleProcesses::const_iterator ip = procs.begin(); ip != procs.end(); ++ip) {
118 printout(ALWAYS,
name(),
"+++ Process %s", (*ip).name.c_str());
121 for (
const auto& [part_name, procs] :
m_processes ) {
122 printout(ALWAYS,
name(),
"+++ PhysicsProcesses of particle %s",part_name.c_str());
123 for (
const Process& p : procs ) {
124 printout(ALWAYS,
name(),
"+++ Process %s ordAtRestDoIt=%d ordAlongSteptDoIt=%d ordPostStepDoIt=%d",
125 p.name.c_str(),p.ordAtRestDoIt,p.ordAlongSteptDoIt,p.ordPostStepDoIt);
142 const std::string& proc_name,
144 int ordAlongSteptDoIt,
157 const std::string& proc_name)
166 physics().emplace_back(phys_name);
174 return (*(ret.first)).second;
181 except(
"Failed to access the physics process '%s' [Unknown-Process]", nam.c_str());
182 throw std::runtime_error(
"Failed to access the physics process");
190 return (*(ret.first)).second;
197 except(
"Failed to access the physics process '%s' [Unknown-Process]", nam.c_str());
198 throw std::runtime_error(
"Failed to access the physics process");
205 if (
nullptr == ctor.pointer )
206 except(
"Failed to instaniate the physics for constructor '%s'", nam.c_str());
210 except(
"Failed to access the physics for constructor '%s' [Unknown physics]", nam.c_str());
211 throw std::runtime_error(
"Failed to access the physics process");
224 except(
"Failed to adopt action object %s as physics constructor. [Invalid-Base]",action->
c_name());
226 except(
"Failed to adopt invalid Geant4Action as PhysicsConstructor. [Invalid-object]");
231 debug(
"constructPhysics %p", physics_pointer);
233 if ( 0 == ctor.pointer ) {
237 except(
"Failed to create the physics for G4VPhysicsConstructor '%s'", ctor.c_str());
239 physics_pointer->RegisterPhysics(ctor.pointer);
240 info(
"Registered Geant4 physics constructor %s to physics list", ctor.c_str());
246 debug(
"constructParticles %p", physics_pointer);
249 G4ParticleDefinition* def = PluginService::Create<G4ParticleDefinition*>(ctor);
252 long* result = (
long*) PluginService::Create<long>(ctor);
253 if ( !result || *result != 1L ) {
254 except(
"Failed to create particle type '%s' result=%d", ctor.c_str(), result);
256 info(
"Constructed Geant4 particle %s [using signature long (*)()]",ctor.c_str());
262 long* result = (
long*) PluginService::Create<long>(ctor);
263 if ( !result || *result != 1L ) {
264 except(
"Failed to create particle type '%s' result=%d", ctor.c_str(), result);
266 info(
"Constructed Geant4 particle group %s [using signature long (*)()]",ctor.c_str());
272 debug(
"constructProcesses %p", physics_pointer);
275 if ( defs.empty() ) {
276 except(
"Particle:%s Cannot find the corresponding entry in the particle table.", part_name.c_str());
278 for (
const Process& p : procs ) {
279 if ( G4VProcess* g4 = PluginService::Create<G4VProcess*>(p.name) ) {
280 for ( G4ParticleDefinition* particle : defs ) {
281 G4ProcessManager* mgr = particle->GetProcessManager();
282 mgr->AddDiscreteProcess(g4);
283 info(
"Particle:%s -> [%s] added discrete process %s",
284 part_name.c_str(), particle->GetParticleName().c_str(), p.name.c_str());
288 except(
"Cannot create discrete physics process %s", p.name.c_str());
291 for (
const auto& [part_name, procs] :
m_processes ) {
294 except(
"Particle:%s Cannot find the corresponding entry in the particle table.", part_name.c_str());
296 for (
const Process& p : procs ) {
297 if ( G4VProcess* g4 = PluginService::Create<G4VProcess*>(p.name) ) {
298 for ( G4ParticleDefinition* particle : defs ) {
299 G4ProcessManager* mgr = particle->GetProcessManager();
300 mgr->AddProcess(g4, p.ordAtRestDoIt, p.ordAlongSteptDoIt, p.ordPostStepDoIt);
301 info(
"Particle:%s -> [%s] added process %s with flags (%d,%d,%d)",
302 part_name.c_str(), particle->GetParticleName().c_str(), p.name.c_str(),
303 p.ordAtRestDoIt, p.ordAlongSteptDoIt, p.ordPostStepDoIt);
307 except(
"Cannot create physics process %s", p.name.c_str());
337 #include <G4FastSimulationPhysics.hh>
341 G4VModularPhysicsList* physics = (
m_extends.empty() )
343 : G4PhysListFactory().GetReferencePhysList(
m_extends);
346 G4FastSimulationPhysics* fastSimulationPhysics =
new G4FastSimulationPhysics();
352 fastSimulationPhysics->ActivateFastSimulation(
"e-");
353 fastSimulationPhysics->ActivateFastSimulation(
"e+");
359 physics->RegisterPhysics( fastSimulationPhysics );
367 physics->RegisterPhysics(
new ParticlePhysics(
this,physics));
371 G4EmParameters::Instance()->SetVerbose(
m_verbosity);
372 G4HadronicParameters::Instance()->SetVerboseLevel(
m_verbosity);
384 printout(ALWAYS,
name(),
"+++ Dump of physics list component(s)");
385 printout(ALWAYS,
name(),
"+++ Extension name %s",
m_extends.c_str());
387 printout(ALWAYS,
name(),
"+++ Program decays: %d",
m_decays);
400 except(
"Geant4EventActionSequence: Attempt to add invalid actor!");
426 G4ParticleTable* pt = G4ParticleTable::GetParticleTable();
427 G4ParticleTable::G4PTblDicIterator* iter = pt->GetIterator();
429 G4Decay* decay =
new G4Decay();
430 info(
"ConstructDecays %p",physics_pointer);
433 G4ParticleDefinition* p = iter->value();
434 G4ProcessManager* mgr = p->GetProcessManager();
435 if (decay->IsApplicable(*p)) {
436 mgr->AddProcess(decay);
438 mgr->SetProcessOrdering(decay, idxPostStep);
439 mgr->SetProcessOrdering(decay, idxAtRest);
G4VPhysicsConstructor * pointer
Pointer to physics constructor object.
ParticleConstructors & particlegroups()
Access all physics particlegroups.
bool m_needsControl
Default property: Flag to create control instance.
virtual void constructPhysics(G4VModularPhysicsList *physics)
constructPhysics callback
void addParticleGroup(const std::string &part_name)
Add physics particle group constructor by name (Leptons, Bosons, Mesons, etc.)
virtual void constructParticles(G4VUserPhysicsList *physics)
Callback to construct particles.
Structure describing a G4 process.
Geant4UIMessenger * control() const
Access to the UI messenger.
CallbackSequence m_physics
Callback sequence for G4 physics constructors.
Process & operator=(const Process &p)
Assignment operator.
G4VUserPhysicsList * extensionList()
Extend physics list from factory:
virtual void installCommandMessenger() override
Install command control messenger if wanted.
static std::vector< G4ParticleDefinition * > g4DefinitionsRegEx(const std::string &expression)
Access Geant4 particle definitions by regular expression.
virtual void constructProcesses(G4VUserPhysicsList *physics)
Execute sequence of G4 physics constructors.
void adopt(Geant4PhysicsList *action)
Add an actor responding to all callbacks. Sequence takes ownership.
Process()
Default constructor.
static void increment(T *)
Increment count according to type information.
Actors< Geant4PhysicsList > m_actors
The list of action objects to be called.
virtual void constructParticles(G4VUserPhysicsList *physics)
Execute sequence of G4 particle constructors.
void constructPhysics(Q *p, void(T::*f)(G4VModularPhysicsList *))
Register physics construction callback.
std::vector< Process > ParticleProcesses
bool m_transportation
Flag if particle transportation is to be added.
void info(const char *fmt,...) const
Support of info messages.
ParticleConstructors & particles()
Access all physics particles.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
virtual void installCommandMessenger() override
Install command control messenger if wanted.
double m_rangecut
global range cut for secondary productions
Geant4PhysicsList(Geant4Context *context, const std::string &nam)
Standard constructor with initailization parameters.
virtual void constructDecays(G4VUserPhysicsList *physics)
Callback to construct particle decays.
CallbackSequence m_particle
Callback sequence for G4 particle constructors.
void addPhysicsConstructor(const std::string &physics_name)
Add PhysicsConstructor by name.
PhysicsConstructors & physics()
Access all physics constructors.
void dump()
Dump content to stdout.
Definition of the generic callback structure for member functions.
ParticleConstructors m_particles
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
CallbackSequence m_process
Callback sequence for G4 process constructors.
PhysicsProcesses & processes()
Access all physics processes.
The implementation of the single Geant4 physics list action sequence.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
virtual ~Geant4PhysicsList()
Default destructor.
const std::string & name() const
Access name of the action.
void dump()
Dump content to stdout.
void addParticleProcess(const std::string &part_name, const std::string &proc_name, int ordAtRestDoIt, int ordAlongSteptDoIt, int ordPostStepDoIt)
Add particle process by name with arguments.
PhysicsProcesses m_processes
void clear()
Clear the sequence and remove all callbacks.
Structure describing a G4 physics constructor.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
PhysicsProcesses m_discreteProcesses
bool m_decays
Flag if particle decays are to be added.
Concrete basic implementation of a Geant4 physics list action.
virtual ~Geant4PhysicsListActionSequence()
Default destructor.
bool transportation() const
Access the transportation flag.
Geant4PhysicsListActionSequence(Geant4Context *context, const std::string &nam)
Standard constructor.
int m_verbosity
verbosity level for the physics list
void adoptPhysicsConstructor(Geant4Action *action)
Add PhysicsConstructor as Geant4Action object.
PhysicsProcesses & discreteProcesses()
Access all physics discrete processes.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
virtual void constructProcesses(G4VUserPhysicsList *physics)
Callback to construct processes (uses the G4 particle table)
const char * c_name() const
Access name of the action.
void addDiscreteParticleProcess(const std::string &part_name, const std::string &proc_name)
Add discrete particle process by name with arguments.
PhysicsConstructors m_physics
void addCall(const std::string &name, const std::string &description, const Callback &cb, size_t npar=0)
Add a new callback structure.
void debug(const char *fmt,...) const
Support of debug messages.
virtual void enable(G4VUserPhysicsList *physics)
Enable physics list: actions necessary to be propagated to Geant4.
void addParticleConstructor(const std::string &part_name)
Add physics particle constructor by name.
ParticleConstructors m_particlegroups
virtual void enable(G4VUserPhysicsList *physics)
Enable physics list: actions necessary to be propagated to Geant4.
Generic context to extend user, run and event information.
std::string m_extends
Property: Store name of basic predefined Geant4 physics list.