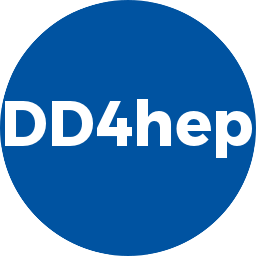 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #include <CLHEP/Random/EngineFactory.h>
20 #include <CLHEP/Random/RandGamma.h>
21 #include <CLHEP/Random/Random.h>
32 unsigned long crc32ul(
const std::string& s);
38 class RNDM :
public TRandom {
42 CLHEP::HepRandomEngine* m_engine;
47 m_engine = m_generator->
engine();
52 virtual void SetSeed(UInt_t seed=0) final {
54 m_generator->
setSeed((
long)seed);
57 virtual void SetSeed(ULong_t seed=0) final {
59 m_generator->
setSeed((
long)seed);
62 virtual Double_t Rndm() final {
63 return m_engine->flat();
66 virtual Double_t Rndm(Int_t)
final {
67 return m_engine->flat();
70 virtual void RndmArray(Int_t size, Float_t *array)
final {
71 for (Int_t i=0;i<size;i++) array[i] = m_engine->flat();
74 virtual void RndmArray(Int_t size, Double_t *array)
final {
75 m_engine->flatArray(size,array);
83 :
Geant4Action(ctxt,nam), m_engine(0), m_rootRandom(0), m_rootOLD(0),
92 m_engine = CLHEP::HepRandom::getTheEngine();
106 if ( s_instance ==
this ) s_instance = 0;
114 if ( !s_instance && throw_exception ) {
115 dd4hep::except(
"Geant4Random",
"No global random number generator defined!");
125 if ( s_instance != ptr ) {
127 dd4hep::except(
"Geant4Random",
"Attempt to declare invalid Geant4Random instance.");
130 dd4hep::except(
"Geant4Random",
"Attempt to declare uninitialized Geant4Random instance.");
133 CLHEP::HepRandomEngine* curr = CLHEP::HepRandom::getTheEngine();
135 ptr->
printP2(
"Moving CLHEP random instance from %p to %p",curr,ptr->
m_engine);
136 CLHEP::HepRandom::setTheEngine(ptr->
m_engine);
148 #include <CLHEP/Random/DualRand.h>
149 #include <CLHEP/Random/JamesRandom.h>
150 #include <CLHEP/Random/MTwistEngine.h>
151 #include <CLHEP/Random/RanecuEngine.h>
152 #include <CLHEP/Random/Ranlux64Engine.h>
153 #include <CLHEP/Random/RanluxEngine.h>
154 #include <CLHEP/Random/RanshiEngine.h>
155 #include <CLHEP/Random/NonRandomEngine.h>
160 std::ifstream in(
m_file.c_str(), std::ios::in);
161 m_engine = CLHEP::EngineFactory::newEngine(in);
163 except(
"Failed to create CLHEP random engine from file:%s.",
m_file.c_str());
169 if (
m_engineType == CLHEP::HepJamesRandom::engineName() )
170 m_engine =
new CLHEP::HepJamesRandom();
171 else if (
m_engineType == CLHEP::RanecuEngine::engineName() )
172 m_engine =
new CLHEP::RanecuEngine();
173 else if (
m_engineType == CLHEP::Ranlux64Engine::engineName() )
174 m_engine =
new CLHEP::Ranlux64Engine();
175 else if (
m_engineType == CLHEP::MTwistEngine::engineName() )
176 m_engine =
new CLHEP::MTwistEngine();
177 else if (
m_engineType == CLHEP::DualRand::engineName() )
179 else if (
m_engineType == CLHEP::RanluxEngine::engineName() )
180 m_engine =
new CLHEP::RanluxEngine();
181 else if (
m_engineType == CLHEP::RanshiEngine::engineName() )
182 m_engine =
new CLHEP::RanshiEngine();
183 else if (
m_engineType == CLHEP::NonRandomEngine::engineName() )
184 m_engine =
new CLHEP::NonRandomEngine();
193 if ( 0 == s_instance ) {
218 except(
"Failed to save RandomGenerator status. [Not-inited]");
232 error(
"Failed to show RandomGenerator status. [Not-inited]");
235 printP2(
"Random engine status of object of type Geant4Random @ 0x%p",
this);
239 printP2(
" Special instance created of type:%s @ 0x%p",
242 printP2(
" Reused HepRandom engine instance %s @ 0x%p",
245 if (
m_engine == CLHEP::HepRandom::getTheEngine() )
246 printP2(
" Instance is identical to Geant4's HepRandom instance.");
248 printP2(
" Instance is %sidentical to ROOT's gRandom instance.",
255 error(
" Geant4Random instance has not engine attached!");
270 return gRandom->Rndm(i);
276 gRandom->RndmArray(n,array);
282 gRandom->RndmArray(n,array);
288 return gRandom->Uniform(x1);
294 return gRandom->Uniform(x1,x2);
300 return gRandom->Exp(tau);
306 return gRandom->Gaus(mean,sigma);
312 return gRandom->Landau(mean,sigma);
318 gRandom->Circle(x,y,r);
324 gRandom->Sphere(x,y,z,r);
330 return gRandom->PoissonD(mean);
336 return gRandom->BreitWigner(mean,
gamma);
342 return CLHEP::RandGamma::shoot(this->m_engine, k, lambda);
double poisson(double mean=1e0)
Create poisson distributed random numbers.
void printP2(const char *fmt,...) const
Support for messages with variable output level using output level+2.
bool m_replace
Property: Indicator to replace the ROOT gRandom instance.
double rndm(int i=0)
Create flat distributed random numbers in the interval ]0,1].
static Geant4Random * instance(bool throw_exception=true)
Access the main Geant4 random generator instance. Must be created before used!
void sphere(double &x, double &y, double &z, double r)
Create tuple of randum number on a sphere with radius r.
CLHEP::HepRandomEngine * engine()
CLHEP random number engine (valid after initialization only)
double uniform(double x1=1)
Create uniformly disributed random numbers in the interval ]0,x1].
std::string m_file
Property: File name if initialized from file. If set, engine name and seeds are ignored.
static void increment(T *)
Increment count according to type information.
double rndm_clhep()
Create flat distributed random numbers in the interval ]0,1] calling CLHEP.
virtual void setSeed(long seed)
Should initialise the status of the algorithm according to seed.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
void error(const char *fmt,...) const
Support of error messages.
double gamma(double k, double lambda)
Create gamma distributed random numbers.
virtual void showStatus() const
Should dump the current engine status on the screen.
virtual ~Geant4Random()
Default destructor.
double breit_wigner(double mean=0e0, double gamma=1e0)
Create breit wigner distributed random numbers.
static Geant4Random * setMainInstance(Geant4Random *ptr)
Make this random generator instance the one used by Geant4.
unsigned long crc32ul(const std::string &s)
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
Geant4Random(Geant4Context *context, const std::string &name)
Standard constructor.
Mini interface to THE random generator of the application.
Default base class for all Geant 4 actions and derivates thereof.
void rndmArray(int n, float *array)
Create a float array of flat distributed random numbers in the interval ]0,1].
virtual void saveStatus(const char filename[]="Config.conf") const
Should save on a file specific to the instantiated engine in use the current status.
void circle(double &x, double &y, double r)
Create tuple of randum number around a circle with radius r.
double gauss(double mean=0, double sigma=1)
Create gaussian distributed random numbers.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
TRandom * m_rootRandom
Reference to ROOT random instance.
CLHEP::HepRandomEngine * m_engine
Reference to the CLHEP random number engine (valid only after initialization)
virtual void setSeeds(const long *seeds, int size)
Should initialise the status of the algorithm.
std::string m_engineType
Property: Engine type. default: "HepJamesRandom".
virtual void restoreStatus(const char filename[]="Config.conf")
Should read from a file and restore the last saved engine configuration.
long m_seed
Property: Initial random seed. Default: 123456789.
bool m_inited
Flag to remember initialization.
double exp(double tau)
Create exponentially distributed random numbers.
void initialize()
Initialize the instance.
double landau(double mean=0, double sigma=1)
Create landau distributed random numbers.
Generic context to extend user, run and event information.