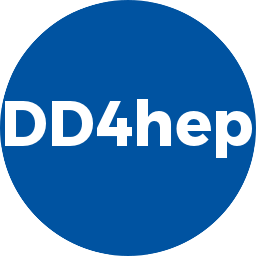 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_WAFERGRIDXY_H
20 #define DDSEGMENTATION_WAFERGRIDXY_H
24 #define MAX_GROUPS 100
25 #define MAX_WAFERS 100
28 namespace DDSegmentation {
146 #endif // DDSEGMENTATION_WAFERGRIDXY_H
void setFieldNameX(const std::string &fieldName)
set the field name used for X
void setOffsetY(double offset)
set the coordinate offset in Y
double offsetX() const
access the coordinate offset in X
std::string _identifierWafer
encoding field used for the wafer
Simple container for a physics vector.
double _waferOffsetY[MAX_GROUPS][MAX_WAFERS]
list of wafer y offset for each group
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
std::string _yId
the field name used for Y
void setGridSizeY(double cellSize)
set the grid size in Y
double gridSizeX() const
access the grid size in X
double _offsetX
the coordinate offset in X
double waferOffsetX(int inGroup, int inWafer) const
access the coordinate waferOffset for inGroup in X
void setOffsetX(double offset)
set the coordinate offset in X
const std::string & fieldNameX() const
access the field name used for X
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
void setWaferOffsetX(int inGroup, int inWafer, double offset)
set the coordinate waferOffset for inlayer in X
double _offsetY
the coordinate offset in Y
std::string _identifierMGWaferGroup
encoding field used for the Magic Wafer group
double gridSizeY() const
access the grid size in Y
double _waferOffsetX[MAX_GROUPS][MAX_WAFERS]
list of wafer x offset for each group
virtual ~WaferGridXY()
destructor
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
WaferGridXY(const std::string &cellEncoding="")
Default constructor passing the encoding string.
double _gridSizeX
the grid size in X
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
double offsetY() const
access the coordinate offset in Y
void setWaferOffsetY(int inGroup, int inWafer, double offset)
set the coordinate waferOffset for inGroup in Y
Namespace for the AIDA detector description toolkit.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
std::string _xId
the field name used for X
const std::string & fieldNameY() const
access the field name used for Y
double waferOffsetY(int inGroup, int inWafer) const
access the coordinate waferOffset for inGroup in Y
double _gridSizeY
the grid size in Y
void setFieldNameY(const std::string &fieldName)
set the field name used for Y
A segmentation class to describe wafer grids in X-Y.
Segmentation base class describing cartesian grid segmentation.
void setGridSizeX(double cellSize)
set the grid size in X