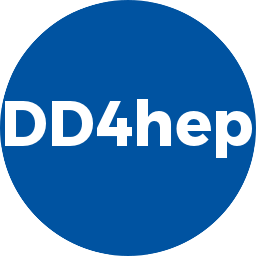 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #ifndef DDSEGMENTATION_CYLINDRICALGRIDPHIZ_H
19 #define DDSEGMENTATION_CYLINDRICALGRIDPHIZ_H
24 namespace DDSegmentation {
131 #endif // DDSEGMENTATION_CYLINDRICALGRIDPHIZ_H
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
Simple container for a physics vector.
Segmentation base class describing a cylindrical grid segmentation.
std::string _zId
the field name used for Z
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
double _offsetPhi
the coordinate offset in phi
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in the following order: R*dPhi,...
void setFieldNameZ(const std::string &fieldName)
set the field name used for Z
virtual ~CylindricalGridPhiZ()
destructor
bool _phiIsSigned
the isSigned attribute of the bitfield used for phi
double gridSizeZ() const
access the grid size in Z
void setFieldNamePhi(const std::string &fieldName)
set the field name used for phi
const std::string & fieldNameZ() const
access the field name used for Z
double offsetPhi() const
access the coordinate offset in phi
double offsetZ() const
access the coordinate offset in Z
double _gridSizePhi
the grid size in phi
double _offsetZ
the coordinate offset in Z
virtual Vector3D position(const CellID &cellID) const
determine the local based on the cell ID
double _gridSizeZ
the grid size in Z
void setOffsetPhi(double offset)
set the coordinate offset in phi
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
Segmentation base class describing cylindrical grid segmentation in the Phi-Z cylinder.
double gridSizePhi() const
access the grid size in phi
virtual void setDecoder(const BitFieldCoder *decoder)
Set the underlying decoder (setting, inter alia, whether phi isSigned)
void setOffsetZ(double offset)
set the coordinate offset in Z
void setGridSizeZ(double cellSize)
set the grid size in Z
std::string _phiId
the field name used for phi
const std::string & fieldNamePhi() const
access the field name used for phi
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
void setRadius(double radius)
set the radius
Namespace for the AIDA detector description toolkit.
CylindricalGridPhiZ(const std::string &cellEncoding)
default constructor using an arbitrary type
double radius() const
access the radius
void setGridSizePhi(double cellSize)
set the grid size in phi