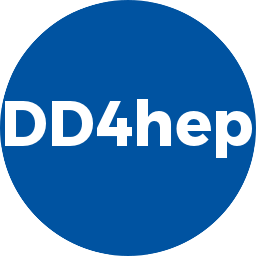 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #ifndef DDSEGMENTATION_CARTESIANGRIDUV_H
19 #define DDSEGMENTATION_CARTESIANGRIDUV_H
24 namespace DDSegmentation {
126 #endif // DDSEGMENTATION_CARTESIANGRIDUV_H
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
double gridSizeU() const
access the grid size in U
void setGridAngle(double angle)
set the rotation angle
void setGridSizeU(double cellSize)
set the grid size in U
void setGridSizeV(double cellSize)
set the grid size in V
Simple container for a physics vector.
void setOffsetV(double offset)
set the coordinate offset in V
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
const std::string & fieldNameU() const
access the field name used for U
double offsetV() const
access the coordinate offset in V
void setFieldNameU(const std::string &fieldName)
set the field name used for U
double _gridSizeU
the grid size in U
double offsetU() const
access the coordinate offset in U
std::string _uId
the field name used for U
void setFieldNameV(const std::string &fieldName)
set the field name used for V
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
double _offsetU
the coordinate offset in U
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
std::string _vId
the field name used for V
virtual ~CartesianGridUV()
destructor
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
Segmentation base class describing cartesian grid segmentation in along U,V rotated some angle from l...
double _gridAngle
the U grid angle
double _gridSizeV
the grid size in V
Namespace for the AIDA detector description toolkit.
double _offsetV
the coordinate offset in V
double gridAngle() const
access the rotation angle
CartesianGridUV(const std::string &cellEncoding="")
Default constructor passing the encoding string.
void setOffsetU(double offset)
set the coordinate offset in U
double gridSizeV() const
access the grid size in V
const std::string & fieldNameV() const
access the field name used for V
Segmentation base class describing cartesian grid segmentation.