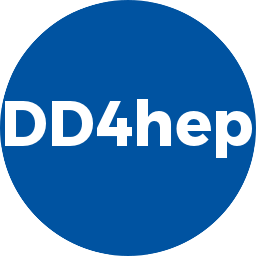 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_POLARGRIDRPHI2_H
20 #define DDSEGMENTATION_POLARGRIDRPHI2_H
27 namespace DDSegmentation {
160 #endif // DDSEGMENTATION_POLARGRIDRPHI2_H
void setOffsetPhi(double offset)
set the coordinate offset in Phi
A segmentation for arbitrary sizes in R and R-dependent sizes in Phi.
void setOffsetR(double offset)
set the coordinate offset in R
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
const std::string & fieldNameR() const
access the field name used for R
void setGridRValues(std::vector< double > const &rValues)
double offsetR() const
access the coordinate offset in R
std::string _rId
the field name used for R
std::vector< double > gridRValues() const
access the grid size in R
double _offsetR
the coordinate offset in R
virtual ~PolarGridRPhi2()
destructor
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
Segmentation base class for polar grids.
double offsetPhi() const
access the coordinate offset in Phi
void setFieldNameR(const std::string &fieldName)
set the field name used for X
void setFieldNamePhi(const std::string &fieldName)
set the field name used for Y
PolarGridRPhi2(const std::string &cellEncoding="")
Default constructor passing the encoding string.
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
double _offsetPhi
the coordinate offset in Phi
const std::string & fieldNamePhi() const
access the field name used for Phi
Namespace for the AIDA detector description toolkit.
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
std::vector< double > gridPhiValues() const
access the grid size in Phi
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions: d...
void setGridRValues(double cellSize, int rID)
set the grid Boundaries in R
std::string _phiId
the field name used for Phi
std::vector< double > _gridPhiValues
the grid sizes in Phi
void setGridSizePhi(double cellSize, int phiID)
set the grid size in Phi
std::vector< double > _gridRValues
the grid boundaries in R
void setGridPhiValues(std::vector< double > const &phiValues)