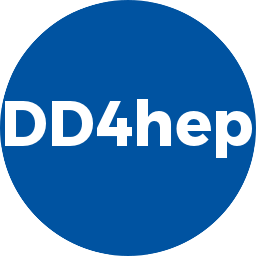 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_POLARGRIDRPHI_H
20 #define DDSEGMENTATION_POLARGRIDRPHI_H
26 namespace DDSegmentation {
118 #endif // DDSEGMENTATION_POLARGRIDRPHI_H
double gridSizeR() const
access the grid size in R
void setFieldNameR(const std::string &fieldName)
set the field name used for X
Simple container for a physics vector.
void setOffsetR(double offset)
set the coordinate offset in R
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
const std::string & fieldNamePhi() const
access the field name used for Phi
void setFieldNamePhi(const std::string &fieldName)
set the field name used for Y
PolarGridRPhi(const std::string &cellEncoding="")
Default constructor passing the encoding string.
void setGridSizeR(double cellSize)
set the grid size in R
double _offsetR
the coordinate offset in R
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
void setGridSizePhi(double cellSize)
set the grid size in Phi
double offsetR() const
access the coordinate offset in R
const std::string & fieldNameR() const
access the field name used for R
double _gridSizePhi
the grid size in Phi
A segmentation for arbitrary sizes in R and R-dependent sizes in Phi.
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
double _gridSizeR
the grid size in R
Segmentation base class for polar grids.
virtual std::vector< double > cellDimensions(const CellID &cID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions: d...
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
std::string _phiId
the field name used for Phi
double offsetPhi() const
access the coordinate offset in Phi
Namespace for the AIDA detector description toolkit.
double _offsetPhi
the coordinate offset in Phi
std::string _rId
the field name used for R
void setOffsetPhi(double offset)
set the coordinate offset in Phi
double gridSizePhi() const
access the grid size in Phi
virtual ~PolarGridRPhi()
destructor