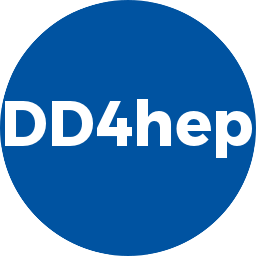 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
11 #ifndef DDSEGMENTATION_CARTESIANGRIDYZ_H
12 #define DDSEGMENTATION_CARTESIANGRIDYZ_H
17 namespace DDSegmentation {
118 #endif // DDSEGMENTATION_CARTESIANGRIDYZ_H
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
void setFieldNameY(const std::string &fieldName)
set the field name used for Y
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
double _offsetY
the coordinate offset in Y
void setOffsetY(double offset)
set the coordinate offset in Y
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
virtual ~CartesianGridYZ()
destructor
CartesianGridYZ(const std::string &cellEncoding="")
Default constructor passing the encoding string.
void setGridSizeY(double cellSize)
set the grid size in Y
const std::string & fieldNameZ() const
access the field name used for Z
double offsetY() const
access the coordinate offset in Y
void setOffsetZ(double offset)
set the coordinate offset in Z
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
std::string _zId
the field name used for Z
const std::string & fieldNameY() const
access the field name used for Y
void setFieldNameZ(const std::string &fieldName)
set the field name used for Z
double offsetZ() const
access the coordinate offset in Z
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
double gridSizeZ() const
access the grid size in Z
Segmentation base class describing cartesian grid segmentation in the Y-Z plane.
void setGridSizeZ(double cellSize)
set the grid size in Z
Namespace for the AIDA detector description toolkit.
double _offsetZ
the coordinate offset in Z
std::string _yId
the field name used for Y
double _gridSizeZ
the grid size in Z
double _gridSizeY
the grid size in Y
Segmentation base class describing cartesian grid segmentation.
double gridSizeY() const
access the grid size in Y