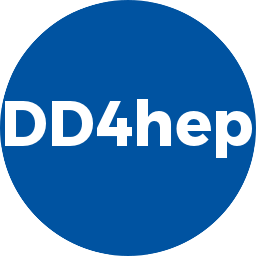 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
12 #ifndef DDSEGMENTATION_GRIDPHIETA_H
13 #define DDSEGMENTATION_GRIDPHIETA_H
27 namespace DDSegmentation {
159 #endif // DDSEGMENTATION_GRIDPHIETA_H
void setFieldNamePhi(const std::string &fieldName)
std::string m_phiID
the field name used for phi
Simple container for a physics vector.
double gridSizePhi() const
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
int m_phiBins
the number of bins in phi
double m_offsetEta
the coordinate offset in eta
void setOffsetPhi(double offset)
double m_offsetPhi
the coordinate offset in phi
virtual Vector3D position(const CellID &aCellID) const
GridPhiEta(const std::string &aCellEncoding)
default constructor using an arbitrary type
void setOffsetEta(double offset)
const std::string & fieldNamePhi() const
std::string m_etaID
the field name used for eta
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
const std::string & fieldNameEta() const
void setPhiBins(int bins)
double eta(const CellID &aCellID) const
double m_gridSizeEta
the grid size in eta
void setFieldNameEta(const std::string &fieldName)
double gridSizeEta() const
void setGridSizeEta(double aCellSize)
Namespace for the AIDA detector description toolkit.
Segmentation class describing segmentation in Phi-Eta.
double phi(const CellID &aCellID) const
virtual ~GridPhiEta()=default
destructor
Base class for all segmentations.
virtual CellID cellID(const Vector3D &aLocalPosition, const Vector3D &aGlobalPosition, const VolumeID &aVolumeID) const