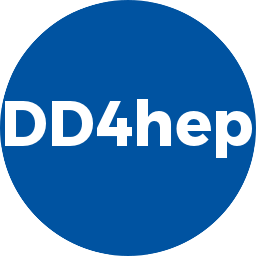 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETAIL_SEGMENTATIONSINTERNA_H
14 #define DD4HEP_DETAIL_SEGMENTATIONSINTERNA_H
27 class DetElementObject;
28 class SegmentationObject;
29 class SensitiveDetectorObject;
31 namespace DDSegmentation {
33 class SegmentationParameter;
55 const std::string&
name()
const;
57 void setName(
const std::string& value);
60 const std::string&
type()
const;
64 const BitFieldCoder*
decoder()
const;
110 #if defined(G__ROOT) || defined(__CLANG__) || defined(__ROOTCLING__)
121 template <
typename IMP>
inline
139 #endif // DD4HEP_DETAIL_SEGMENTATIONSINTERNA_H
VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
DDSegmentation::Parameters parameters() const
Access to all parameters.
SegmentationObject(DDSegmentation::Segmentation *seg=0)
Standard constructor.
SegmentationParameter * Parameter
DDSegmentation::Segmentation * segmentation
Reference to base segmentation.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
void neighbours(const CellID &cellID, std::set< CellID > &neighbours) const
Calculates the neighbours of the given cell ID and adds them to the list of neighbours.
virtual ~SegmentationObject()
Default destructor.
Handle< SensitiveDetectorObject > sensitive
Reference to hosting top level sensitve detector structure.
Implementation class supporting generic Segmentation of sensitive detectors.
Class to hold a segmentation parameter with its description.
virtual ~SegmentationWrapper()
Default destructor.
unsigned char useForHitPosition
Flag to use segmentation for hit positioning.
Concrete wrapper class for segmentation implementation based on DDSegmentation objects.
std::vector< Parameter > Parameters
const std::string & description() const
Access the description of the segmentation.
IMP * implementation
DDSegmentation aggregate.
SegmentationWrapper(const BitFieldCoder *decoder)
Standard constructor.
Handle< DetElementObject > detector
Reference to hosting top level DetElement structure.
const std::string & type() const
Access the segmentation type.
const std::string & name() const
Access the segmentation name.
ROOT::Math::XYZVector Position
unsigned long magic
Magic word to check object integrity.
dd4hep::DDSegmentation::VolumeID VolumeID
void setParameters(const DDSegmentation::Parameters ¶meters)
Set all parameters from an existing set of parameters.
CellID cellID(const Position &localPosition, const Position &globalPosition, const VolumeID &volumeID) const
Determine the cell ID based on the position.
Namespace for the AIDA detector description toolkit.
Position position(const CellID &cellID) const
Determine the local position based on the cell ID.
const BitFieldCoder * decoder() const
Access the underlying decoder.
std::string fieldDescription() const
Access the encoding string.
Base class for all segmentations.
DDSegmentation::Parameter parameter(const std::string ¶meterName) const
Access to parameter by name.
void setDecoder(const BitFieldCoder *decoder) const
Set the underlying decoder.
void setName(const std::string &value)
Set the segmentation name.
dd4hep::DDSegmentation::CellID CellID