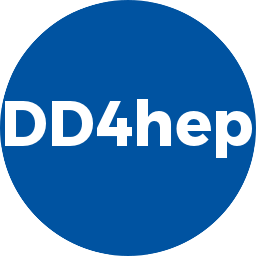 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 #ifndef DDSEGMENTATION_SEGMENTATIONPARAMETER_H
21 #define DDSEGMENTATION_SEGMENTATIONPARAMETER_H
29 namespace DDSegmentation {
33 inline std::vector<std::string>
splitString(
const std::string& s,
char delimiter =
' ') {
34 std::vector<std::string> elements;
35 std::stringstream ss(s);
37 while (std::getline(ss, item, delimiter)) {
38 elements.emplace_back(item);
45 static const char*
name() {
46 return typeid(TYPE).
name();
52 static const char*
name() {
59 static const char*
name() {
66 static const char*
name() {
73 static const char*
name() {
80 static const char*
name() {
87 static const char*
name() {
94 static const char*
name() {
117 const std::string&
name()
const {
129 virtual std::string
type()
const = 0;
131 virtual std::string
value()
const = 0;
152 bool isOpt =
false) :
168 #if defined(G__ROOT) || defined(__CLANG__) || defined(__ROOTCLING__)
176 bool isOpt =
false) :
232 #if defined(G__ROOT) || defined(__CLANG__) || defined(__ROOTCLING__)
265 s << TypeName<TYPE>::name() <<
"vec";
272 for (
const auto& it : *
_value ) {
281 std::vector<std::string> elements =
splitString(val);
283 for (std::vector<std::string>::const_iterator it = elements.begin(); it != elements.end(); ++it) {
284 if (not it->empty()) {
289 _value->emplace_back(entry);
297 typename std::vector<TYPE>::const_iterator it =
_defaultValue.begin();
313 #endif // DDSEGMENTATION_SEGMENTATIONPARAMETER_H
const TYPE & typedValue() const
Access to the parameter value.
UnitType _unitType
The unit type.
const std::string & description() const
Access to the parameter description.
static const char * name()
std::string defaultValue() const
Access to the parameter default value in string representation.
const TYPE & typedDefaultValue() const
Access to the parameter default value.
TypedSegmentationParameter(const std::string &nam, const std::string &desc, TYPE &val, const TYPE &default_Value, SegmentationParameter::UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Default constructor.
void setValue(const std::string &val)
Set the parameter value in string representation.
std::string value() const
Access to the parameter value in string representation.
std::string _name
The parameter name.
static const char * name()
static const char * name()
Class to hold a segmentation parameter with its description.
virtual std::string type() const =0
Access to the parameter type.
static const char * name()
bool _isOptional
Store if parameter is optional.
std::string _description
The parameter description.
std::string type() const
Access to the parameter type.
static const char * name()
const std::string & name() const
Access to the parameter name.
std::string toString() const
Printable version.
virtual ~SegmentationParameter()
Default destructor.
const std::vector< TYPE > & typedValue() const
Access to the parameter value.
std::vector< TYPE > _defaultValue
void setValue(const std::string &val)
Set the parameter value in string representation.
void setTypedValue(const TYPE &val)
Set the parameter value.
SegmentationParameter(const std::string &nam, const std::string &desc, UnitType unitTyp=NoUnit, bool isOpt=false)
Default constructor used by derived classes.
bool isOptional() const
Check if this parameter is optional.
std::string value() const
Access to the parameter value in string representation.
virtual void setValue(const std::string &value)=0
Set the parameter value in string representation.
std::vector< std::string > splitString(const std::string &s, char delimiter=' ')
Helper method to split string into tokens.
virtual std::string defaultValue() const =0
Access to the parameter default value in string representation.
std::string type() const
Access to the parameter type.
virtual ~TypedSegmentationParameter()
Default destructor.
static const char * name()
static const char * name()
const std::vector< TYPE > & typedDefaultValue() const
Access to the parameter default value.
Namespace for the AIDA detector description toolkit.
Concrete class to hold a segmentation parameter of a given type with its description.
void setTypedValue(const std::vector< TYPE > &val)
Set the parameter value.
virtual ~TypedSegmentationParameter()
Default destructor.
static const char * name()
Helper class to extract type names.
std::string defaultValue() const
Access to the parameter default value in string representation.
UnitType
Defines the parameter unit type (useful to convert to default set of units)
static const char * name()
virtual std::string value() const =0
Access to the parameter value in string representation.
UnitType unitType() const
Access to the unit type.