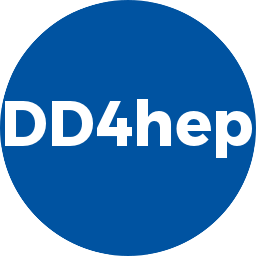 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_PROJECTIVECYLINDER_H
20 #define DDSEGMENTATION_PROJECTIVECYLINDER_H
25 namespace DDSegmentation {
112 #endif // DDSEGMENTATION_PROJECTIVECYLINDER_H
double _offsetPhi
the coordinate offset in phi
std::string _thetaID
the field name used for theta
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the position
Simple container for a physics vector.
void setFieldNamePhi(const std::string &fieldName)
set the field name used for phi
Segmentation base class describing a cylindrical grid segmentation.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
void setPhiBins(int bins)
set the number of bins in phi
virtual ~ProjectiveCylinder()
destructor
double offsetTheta() const
access the coordinate offset in theta
ProjectiveCylinder(const std::string &cellEncoding)
default constructor using an arbitrary type
void setOffsetPhi(double offset)
set the coordinate offset in phi
int thetaBins() const
access the number of bins in theta
std::string fieldNameTheta() const
access the field name used for theta
void setFieldNameTheta(const std::string &fieldName)
set the field name used for theta
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
A segmentation class to describe projective cylinders.
double _offsetTheta
the coordinate offset in theta
int phiBins() const
access the number of bins in theta
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
int _thetaBins
the number of bins in theta
double phi(const CellID &cellID) const
determine the azimuthal angle phi based on the cell ID
double offsetPhi() const
access the coordinate offset in phi
Namespace for the AIDA detector description toolkit.
double theta(const CellID &cellID) const
determine the polar angle theta based on the cell ID
int _phiBins
the number of bins in phi
void setThetaBins(int bins)
set the number of bins in theta
virtual Vector3D position(const CellID &cellID) const
determine the position based on the cell ID
void setOffsetTheta(double offset)
set the coordinate offset in theta
std::string _phiID
the field name used for phi
std::string fieldNamePhi() const
access the field name used for phi