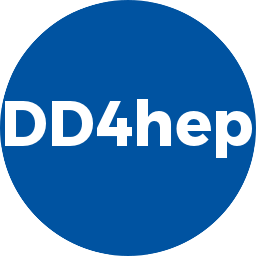 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
45 const std::string& path,
46 const std::vector<std::regex>& matches);
71 #include <TTimeStamp.h>
72 #include <TGeoManager.h>
74 #include <G4PVPlacement.hh>
75 #include <G4VSensitiveDetector.hh>
98 const std::string& path,
99 const std::vector<std::regex>& matches)
101 std::size_t count = 0;
103 if ( volumes.find(pv.
volume()) == volumes.end() ) {
104 if( !path.empty() ) {
105 for(
const auto& match : matches ) {
107 bool stat = std::regex_search(path, sm, match);
109 volumes.insert(pv.
volume());
116 for(
int i=0, num = pv->GetNdaughters(); i < num; ++i ) {
118 std::string daughter_path = path +
"/" + daughter.
name();
119 count += this->
collect_volumes(volumes, daughter, daughter_path, matches);
135 except(
"Failed to locate subdetector DetElement %s to manage Geant4 energy deposits.",
det);
139 except(
"Failed to locate sensitive detector %s to manage Geant4 energy deposits.",
det);
141 std::string nam = sd.
name();
142 auto iter = types.find(nam);
143 std::string typ = (iter != types.end()) ? (*iter).second : dflt;
146 std::set<Volume> volumes;
147 int flags = std::regex_constants::icase | std::regex_constants::ECMAScript;
148 std::vector<std::regex> expressions;
150 std::regex e(val, (std::regex_constants::syntax_option_type)flags);
151 expressions.emplace_back(e);
154 info(
"%s Starting to scan volume....",
det);
156 for(
const auto& vol : volumes ) {
157 G4LogicalVolume* g4vol = g4info->
g4Volumes[vol];
159 except(
"+++ Failed to access G4LogicalVolume for SD %s of type %s", nam.c_str(), typ.c_str());
161 debug(
"%s Assign sensitive detector [%s] to volume: %s.",
162 nam.c_str(), typ.c_str(), vol.name());
166 info(
"%s Handled %ld nodes with %ld sensitive volume type(s). Total of %7.3f seconds.",
167 det, num_nodes, volumes.size(), stop.AsDouble()-start.AsDouble() );
virtual ~Geant4RegexSensitivesConstruction()
Default destructor.
Handle class to hold the information of a sensitive detector.
Handle class holding a placed volume (also called physical volume)
Class to create Geant4 detector geometry from TGeo representation in memory.
PlacedVolume placement() const
Access to the physical volume of this detector element.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
bool isValid() const
Check the validity of the object held by the handle.
static void increment(T *)
Increment count according to type information.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
const char * name() const
Access the object name (or "" if not supported by the object)
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
const std::map< std::string, std::string > & sensitiveDetectorTypes() const
Property access: Names with specialized factories to create G4VSensitiveDetector instances.
Handle class describing a detector element.
Detector & description
Reference to geometry object.
std::size_t collect_volumes(std::set< Volume > &volumes, PlacedVolume pv, const std::string &path, const std::vector< std::regex > &matches)
Geant4GeometryInfo * ptr() const
Access to the data pointer.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
const std::string defaultSensitiveDetectorType() const
Property access: Name of the default factory to create G4VSensitiveDetector instances.
void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitives construction callback. Called at "ConstructSDandField()".
const std::string & placementPath() const
Access to the full path to the placed object.
std::string detector_name
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
void setSensitiveDetector(G4LogicalVolume *vol, G4VSensitiveDetector *sd)
Helper: Assign sensitive detector to logical volume.
Namespace for the AIDA detector description toolkit.
Volume volume() const
Logical volume of this placement.
void debug(const char *fmt,...) const
Support of debug messages.
Basic implementation of the Geant4 detector construction action.
std::vector< std::string > regex_values
Generic context to extend user, run and event information.
Geant4RegexSensitivesConstruction(Geant4Context *ctxt, const std::string &nam)
Initializing constructor for DDG4.
Geant4Context * context() const
Access the context.
Geant4GeometryMaps::VolumeMap g4Volumes