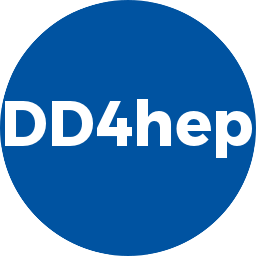 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
40 #include <TGFileDialog.h>
41 #include <TEveScene.h>
42 #include <TEveBrowser.h>
43 #include <TEveManager.h>
44 #include <TEveCaloData.h>
46 #include <TEveViewer.h>
47 #include <TEveCompound.h>
48 #include <TEveBoxSet.h>
49 #include <TEvePointSet.h>
50 #include <TEveGeoShape.h>
51 #include <TEveTrackPropagator.h>
52 #include <TGeoManager.h>
64 void EveDisplay(
const char* xmlConfig = 0,
const char* eventFileName = 0) {
66 if ( xmlConfig != 0 ) {
68 ::snprintf(text,
sizeof(text),
"%s%s",strncmp(xmlConfig,
"file:",5)==0 ?
"file:" :
"",xmlConfig);
72 display->
MessageBox(INFO,
"No DDEve setup given.\nYou need to choose now.....\n"
73 "If you need an example, open\n\n"
74 "examples/CLIDSid/eve/DDEve.xml\n"
75 "and the corresponding event data\n"
76 "examples/CLIDSid/eve/CLICSiD_Events.root\n\n\n",
77 "Need to choos setup file");
81 if (eventFileName != 0) {
89 : m_eve(eve), m_detDesc(0), m_evtHandler(0), m_geoGlobal(0), m_eveGlobal(0),
90 m_viewMenu(0), m_dd4Menu(0), m_visLevel(7), m_loadLevel(1)
92 TEveBrowser* br =
m_eve->GetBrowser();
93 TGMenuBar* menu = br->GetMenuBar();
98 TEveGeoShape::GetGeoMangeur()->AddNavigator();
99 TEveGeoShape::GetGeoMangeur()->SetCurrentNavigator(0);
103 br->ShowCloseTab(kFALSE);
104 m_eve->GetViewers()->SwitchColorSet();
105 TFile::SetCacheFileDir(
".");
107 br->SetTabTitle(
"Global Scene",TRootBrowser::kRight,0);
112 TRootBrowser* br =
m_eve->GetBrowser();
143 m_eve->FullRedraw3D(kTRUE);
149 throw std::runtime_error(
"This call is not implemented !");
172 throw std::runtime_error(
"Invalid event handler");
178 menu->
Build(menubar, hints);
179 m_eve->FullRedraw3D(kTRUE);
184 DisplayConfiguration::ViewConfigurations::const_iterator i;
185 for(i=config.
views.begin(); i!=config.
views.end(); ++i)
188 DisplayConfiguration::Configurations::const_iterator j;
206 const char* n = nam.c_str();
209 h->SetTitle(hits.c_str());
210 ctx.
eveHist =
new TEveCaloDataHist();
214 ctx.
eveHist->GetEtaBins()->SetTitleFont(120);
215 ctx.
eveHist->GetEtaBins()->SetTitle(
"h");
216 ctx.
eveHist->GetPhiBins()->SetTitleFont(120);
217 ctx.
eveHist->GetPhiBins()->SetTitle(
"f");
224 ctx.
calo3D->SetAutoRange(kTRUE);
241 throw std::runtime_error(
"Cannot access calodata configuration " + nam);
248 ViewConfigurations::const_iterator i =
m_viewConfigs.find(nam);
273 std::string path = TString::Format(
"%s/", TROOT::GetIconPath().Data()).Data();
274 const TGPicture* pic = 0;
275 if ( level == VERBOSE )
276 pic =
client().GetPicture((path+
"mb_asterisk_s.xpm").c_str());
277 else if ( level == DEBUG )
278 pic =
client().GetPicture((path+
"mb_asterisk_s.xpm").c_str());
279 else if ( level == INFO )
280 pic =
client().GetPicture((path+
"mb_asterisk_s.xpm").c_str());
281 else if ( level == WARNING )
282 pic =
client().GetPicture((path+
"mb_excalamation_s.xpm").c_str());
283 else if ( level == ERROR )
284 pic =
client().GetPicture((path+
"mb_stop.xpm").c_str());
285 else if ( level == FATAL )
286 pic =
client().GetPicture((path+
"interrupt.xpm").c_str());
287 new TGMsgBox(gClient->GetRoot(),0,title.c_str(),text.c_str(),pic,
288 kMBDismiss,0,kVerticalFrame,kTextLeft|kTextCenterY);
293 static const char *evtFiletypes[] = {
294 "xml files",
"*.xml",
295 "XML files",
"*.XML",
300 fi.fFileTypes = evtFiletypes;
301 fi.fIniDir = StrDup(default_dir.c_str());
303 new TGFileDialog(
client().GetRoot(), 0, kFDOpen, &fi);
304 if ( fi.fFilename ) {
305 std::string ret = fi.fFilename;
306 if ( ret.find(
"file:") != 0 )
return "file:"+ret;
314 static const char *evtFiletypes[] = {
315 "ROOT files",
"*.root",
316 "SLCIO files",
"*.slcio",
317 "LCIO files",
"*.lcio",
322 fi.fFileTypes = evtFiletypes;
323 fi.fIniDir = StrDup(default_dir.c_str());
325 new TGFileDialog(
client().GetRoot(), 0, kFDOpen, &fi);
326 if ( fi.fFilename ) {
334 if ( 0 == menubar ) {
335 menubar =
m_eve->GetBrowser()->GetMenuBar();
349 TFile* f = TFile::Open(name);
350 if ( f && !f->IsZombie() )
return f;
351 throw std::runtime_error(
"+++ Failed to open ROOT file:"+std::string(name));
361 typedef std::vector<EventHandler::Collection> Collections;
362 const Types& types = handler.
data();
363 TEveElement* particles = 0;
365 printout(ERROR,
"EventHandler",
"+++ Display new event.....");
366 manager().GetEventScene()->DestroyElements();
367 for(Types::const_iterator ityp=types.begin(); ityp!=types.end(); ++ityp) {
368 const Collections& colls = (*ityp).second;
369 for(Collections::const_iterator j=colls.begin(); j!=colls.end(); ++j) {
370 std::size_t len = (*j).second;
372 const char* nam = (*j).first;
378 if ( icfg != cfgend ) {
380 if ( ::toupper(cfg.
use[0]) ==
'T' || ::toupper(cfg.
use[0]) ==
'Y' ) {
381 if ( cfg.
hits ==
"PointSet" ) {
386 else if ( cfg.
hits ==
"BoxSet" ) {
391 else if ( cfg.
hits ==
"TowerSet" ) {
418 printout(INFO,
"Display",
"+++ StartVertexPoints: Filled %d start vertex points.....",cr.
count);
422 MCParticleCreator cr(
new TEveTrackPropagator(
"",
"",
new TEveMagFieldDuo(350, -3.5, 2.0)),
423 new TEveCompound(
"MC_Particles",
"MC_Particles"),
424 icfg == cfgend ? 0 : &((*icfg).second));
426 printout(INFO,
"Display",
"+++ StartVertexPoints: Filled %d patricle tracks.....",cr.
count);
435 (*i).second.eveHist->GetHist(0)->Reset();
438 TH2F* h = ctx.
eveHist->GetHist(0);
442 printout(INFO,
"FillEtaPhiHistogram",
"+++ %s: Filled %ld hits from %s....",
451 (*i)->ConfigureEventFromInfo();
470 GetGeo().AddElement(topic);
473 return *((*i).second);
480 throw std::runtime_error(
"Display: Attempt to access non-existing geometry topic:"+name);
482 return *((*i).second);
491 manager().GetEventScene()->AddElement(topic);
494 return *((*i).second);
501 throw std::runtime_error(
"Display: Attempt to access non-existing event topic:"+name);
503 return *((*i).second);
523 manager().GetEventScene()->AddElement(el);
530 if ( world.
children().size() == 0 ) {
531 MessageBox(INFO,
"It looks like there is no\nGeometry loaded.\nNo event display availible.\n");
533 else if ( levels > 0 ) {
539 printout(INFO,
"Display",
"+++ Load children of %s to %d levels",
541 for (DetElement::Children::const_iterator i = c.begin(); i != c.end(); ++i) {
546 if ( e.second && e.first ) {
547 parent.AddElement(e.second);
552 TGeoNode* n = (TGeoNode*)start->GetUserData();
553 printout(INFO,
"Display",
"+++ Load children of %s to %d levels",
Utilities::GetName(start),levels);
556 const char* node_name = n->GetName();
559 std::pair<bool,TEveElement*> e(
false,0);
561 for (DetElement::Children::const_iterator i = c.begin(); i != c.end(); ++i) {
568 if ( !e.first && !e.second ) {
572 start->AddElement(e.second);
574 printout(INFO,
"Display",
"+++ Import geometry node %s with %d levels.",node_name, levels);
577 printout(INFO,
"Display",
"+++ FAILED to import geometry node %s with %d levels.",node_name, levels);
592 printout(INFO,
"Display",
"+++ %s element %s with a depth of %d.",
virtual CollectionType collectionType(const std::string &collection) const =0
Access to the collection type by name.
const DataConfig * GetCalodataConfiguration(const std::string &name) const
Access a data filter by name. Data filters are used to customize views.
const Children & children() const
Access to the list of children.
Fill a 3D tower set from a hit collection.
void MakeNodesVisible(TEveElement *e, bool visible, int level)
Make a set of nodes starting from a top element (in-)visible with a given depth.
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
The main class of the DDEve display.
IFACE * addExtension(CONCRETE *c)
Extend the sensitive detector element with an arbitrary structure accessible by the type.
void MessageBox(PrintLevel level, const std::string &text, const std::string &title="") const
Open standard message box.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
Event handler base class. Interface to all DDEve I/O actions.
std::string getEventHandlerName()
Get Event Handler Plugin name.
Topics m_eveTopics
Event data topics.
ViewConfigurations m_viewConfigs
Container with view configurations.
Handle class to hold the information of a sensitive detector.
Menus m_menus
Container with top level menues.
int findNodeWithMatrix(TGeoNode *p, TGeoNode *n, TGeoHMatrix *mat, std::string *sub_path=0)
Event handler base class: Interface to all DDEve I/O actions.
void ImportGeo(TEveElement *el)
Call to import geometry elements.
ViewMenu * m_viewMenu
Reference to the view menu.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor) override
Loop over collection and extract data.
PlacedVolume placement() const
Access to the physical volume of this detector element.
virtual TEveElementList & GetGeoTopic(const std::string &name)
Access/Create an geometry topic by name.
TEveElement * element() const
Return eve element.
std::string OpenEventFileDialog(const std::string &default_dir) const
Popup ROOT file chooser. returns chosen file name; empty on cancel.
virtual void Subscribe(EventConsumer *display)
Subscribe to notification of new data present.
void LoadGeoChildren(TEveElement *start, int levels, bool redraw)
Load 'levels' Children into the geometry scene.
bool isValid() const
Check the validity of the object held by the handle.
int m_visLevel
TGeoManager visualisation level.
Local implementation with overrides of the TEveElementList.
const char * name() const
Access the object name (or "" if not supported by the object)
DataConfigurations m_calodataConfigs
Container with calorimeter data display configurations.
const ViewConfig * GetViewConfiguration(const std::string &name) const
Access a data filter by name. Data filters are used to customize views.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::pair< bool, TEveElement * > createEveShape(int level, int max_level, TEveElement *p, TGeoNode *n, const TGeoHMatrix &mat, const std::string &node_name)
ClassImp(Display) namespace dd4hep
Views m_eveViews
Container with configured event views.
std::string OpenXmlFileDialog(const std::string &default_dir) const
Popup XML file chooser. returns chosen file name; empty on cancel.
Fill a 3D pointset from a hit collection.
static Detector & getInstance(const std::string &name="default")
—Factory method----—
Handle class describing a detector element.
const char * GetName(T *p)
CalodataContext & GetCaloHistogram(const std::string &name)
Access to calo data histograms by name as defined in the configuration.
TEveCaloDataHist * eveHist
DD4hep internal namespace.
void EveDisplay(const char *xmlFile, const char *eventFileName)
ViewConfigurations views
Container with view configurations.
Fill EtaPhi histograms from a hit collection.
TEveElement * element() const
Return eve element.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
void LoadXML(const char *xmlFile)
Load geometry from compact xml file.
TEveElementList * m_eveGlobal
Event item element list.
Detector & detectorDescription() const
Access to geometry hub.
TGClient & client() const
Access to X-client.
void destroyData(bool destroy_mgr=true)
Clear data content: releases all allocated resources.
DisplayConfiguration::Config config
int m_loadLevel
Load level for the eve geometry.
virtual ~Display()
Default destructor.
Detector * m_detDesc
Reference to geometry hub.
GenericEventHandler * m_evtHandler
Reference to the event reader object.
Container with full display configuration.
TEveElementList & GetGeo()
Access / Create global geometry element.
std::pair< bool, TEveElement * > LoadDetElement(DetElement element, int levels, TEveElement *parent)
Calodata m_calodata
Container with calorimeter data (projections)
Display(TEveManager *eve)
Standard constructor.
virtual bool Open(const std::string &type, const std::string &file_name) override
Open a new event data file.
Fill a 3D pointset with particle start vertices.
void LoadGeometryRoot(const char *rootFile)
Load geometry from compact xml file.
std::map< std::string, DetElement > Children
void close()
Close compounds.
virtual TEveElementList & GetEveTopic(const std::string &name)
Access/Create an event topic by name.
void ChooseGeometry()
Load geometry with panel.
T * ptr() const
Access to the held object.
GenericEventHandler & eventHandler() const
Access to the event reader.
Configuration class for 3D calorimeter data display.
Topics m_geoTopics
Geometry data topics.
Generic display configuration structure for DDEve.
virtual void AddMenu(TGMenuBar *menuBar, PopupMenu *menu, int hints=kLHintsNormal)
Add new menu to the main menu bar.
Namespace for the AIDA detector description toolkit.
void ImportEvent(TEveElement *el)
Call to import top level event elements.
virtual void OnFileOpen(EventHandler &handler) override
Consumer overload: open file.
Data implementation class of the Detector interface.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor)=0
Loop over collection and extract hit data.
TFile * Open(const char *rootFile) const
Open ROOT file.
virtual void fromXML(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT)=0
Read any geometry description or alignment file.
The main interface to the dd4hep detector description package.
virtual void BuildMenus(TGMenuBar *menuBar=0)
Build the DDEve specific menues. Default bar is the ROOT browser's bar.
class View View.h DDEve/View.h
Calorimeter data context for the DDEve event display.
void MakeNodesVisible(TEveElement *e, bool visible, int level)
Make a set of nodes starting from a top element (in-)visible with a given depth.
virtual void UnregisterEvents(View *view)
Unregister from the main event scene.
Configurations calodata
Container with calorimeter data configurations.
std::map< std::string, std::vector< Collection > > TypedEventCollections
Types collection: collections are grouped by type (class name)
virtual void RegisterEvents(View *view)
Register to the main event scene on new events.
void ImportConfiguration(const DisplayConfiguration &config)
Import configuration parameters.
Fill eve particles from a MC particle collection.
DD4hepMenu * m_dd4Menu
Reference to the specialized ddeve menu.
TEveElement * element() const
Return eve element.
virtual void OnNewEvent(EventHandler &handler) override
EventConsumer overload: Consumer event data.
TEveManager & manager() const
Access to the EVE manager.
virtual const TypedEventCollections & data() const =0
Access the map of simulation data collections.
TEveElementList * m_geoGlobal
Geometry item element list.
Fill a 3D box set from a hit collection.
IFACE * removeExtension(bool destroy=true)
Remove an existing extension object from the Detector instance. If not destroyed, the instance is ret...
Configurations collections
Container for data collection configurations.
TEveManager * m_eve
Reference to TEve manager.
DataConfigurations m_collectionsConfigs
Container with non-calorimeter data display configurations.