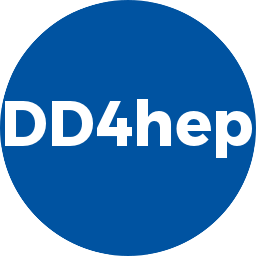 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
21 #include <TEveScene.h>
22 #include <TEveViewer.h>
47 typedef std::map<std::string, TEveElementList*>
Topics;
116 virtual std::pair<bool,TEveElement*>
117 CreateGeometry(DetElement de,
const DisplayConfiguration::Config& cfg);
119 virtual std::pair<bool,TEveElement*>
124 virtual void ImportGeo(
const std::string& topic, TEveElement* element);
128 virtual void ImportGeo(TEveElement* element);
146 virtual void ConfigureEvent(
const DisplayConfiguration::ViewConfig& config);
156 #endif // DDEVE_VIEW_H
virtual void ConfigureGeometry(const DisplayConfiguration::ViewConfig &config)
Configure a single geometry view.
The main class of the DDEve display.
const char * c_name() const
TEveScene * geoScene() const
Access to the Eve geometry scene.
ClassDef(View, 0)
Root implementation macro.
View(Display *eve, const std::string &name)
Initializing constructor.
virtual std::pair< bool, TEveElement * > GetGlobalGeometry(DetElement de, const DisplayConfiguration::Config &cfg)
Access the global instance of the subdetector geometry.
virtual void ImportEvent(TEveElement *element)
Call to import event elements into the main event scene.
virtual void ConfigureGeometryFromGlobal()
Configure a single geometry view by default from the global geometry scene with all subdetectors.
virtual View & Map(TEveWindow *slot)
Map the view view to the slot.
virtual std::pair< bool, TEveElement * > CreateGeometry(DetElement de, const DisplayConfiguration::Config &cfg)
Create a new instance of the geometry of a sub-detector.
std::map< std::string, TEveElementList * > Topics
virtual ~View()
Default destructor.
virtual View & Build(TEveWindow *slot)
Build the view view and map it to the given slot.
virtual void ConfigureGeometryFromInfo()
Configure a view with geo info. Used configuration if present.
void setShowGlobal(bool value)
Set show globals.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
TEveScene * eveScene() const
Access to the Eve event scene.
const DisplayConfiguration::ViewConfig * m_config
virtual TEveElementList & GetGeoTopic(const std::string &name)
Access/Create an geometry topic by name.
virtual TEveElement * ImportEventElement(TEveElement *element, TEveElementList *list)
Call an element to a event element list.
const std::string & name() const
Access to the view name/title.
virtual TEveElementList * AddToGlobalItems(const std::string &nam)
Add the view to the global list of eve objects.
View TEveWindowSlot * slot
virtual TEveElement * ImportGeoElement(TEveElement *element, TEveElementList *list)
Call an element to a geometry element list.
virtual View & CreateGeoScene()
Create the geometry scene.
virtual View & CreateEventScene()
Create the event scene.
virtual TEveElement * ImportGeoTopic(TEveElement *element, TEveElementList *list)
Call an element to a geometry element list.
virtual void ImportEventTopics()
Import event typics after creation.
virtual void ConfigureEventFromInfo()
Configure a view with event info. Used configuration if present.
virtual void ConfigureEventFromGlobal()
Configure an event view by default from the global event scene.
TEveScene * m_geoScene
Reference to the geometry scene.
TEveViewer * viewer() const
Access to the Eve viewer.
virtual void ImportGeoTopics(const std::string &title)
Call to import geometry topics. If title is empty, do not add to global item list.
virtual View & CreateScenes()
Create the geometry and the event scene.
std::string m_name
The name of the view.
TEveElementList * m_global
Reference to the global item (if added.
TEveViewer * m_view
Reference to the view.
Namespace for the AIDA detector description toolkit.
virtual void Initialize()
Initialize the view port.
bool showGlobal() const
Show global directory.
class View View.h DDEve/View.h
TEveScene * m_eveScene
Reference to the event scene.
virtual void ImportGeo(const std::string &topic, TEveElement *element)
Call to import geometry elements into topics.
virtual void ConfigureEvent(const DisplayConfiguration::ViewConfig &config)
Configure a single event scene view.