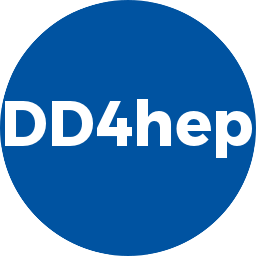 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDEVE_EVENTHANDLER_H
14 #define DDEVE_EVENTHANDLER_H
27 #define MEV_2_GEV 0.001
109 virtual bool Open(
const std::string& type,
const std::string& file_name) = 0;
141 #endif // DDEVE_EVENTHANDLER_H
virtual CollectionType collectionType(const std::string &collection) const =0
Access to the collection type by name.
Event data actor base class for particles. Used to extract data from concrete classes.
DDEve event classes: Basic hit.
virtual bool PreviousEvent()=0
User overloadable function: Load the previous event.
bool m_hasEvent
Flag to indicate that an event is loaded.
Event handler base class: Interface to all DDEve I/O actions.
virtual ~DDEveParticleActor()
ClassDef(EventConsumer, 0)
virtual void operator()(const DDEveParticle &)=0
Event data actor base class for hits. Used to extract data from concrete classes.
virtual bool Open(const std::string &type, const std::string &file_name)=0
Open a new event data file.
virtual std::string datasourceName() const =0
Access the data source name.
virtual bool hasEvent() const
Check if an event is present in memory.
bool m_hasFile
Flag to indicate that a file is opened.
Event event consumer base class for DDEve: Interface class for event I/O.
EventHandler()=default
Standard constructor.
virtual void OnNewEvent(EventHandler &)=0
Consumer event data callback.
virtual bool hasFile() const
Check if a data file is connected to the handler.
virtual void operator()(const DDEveHit &)=0
virtual bool NextEvent()=0
Load the next event.
virtual ~EventConsumer()
Default destructor.
virtual void OnFileOpen(EventHandler &)=0
Consumer file open callback.
ClassDef(EventHandler, 0)
virtual void setSize(size_t)
std::pair< const char *, size_t > Collection
Collection definition: name, size.
Namespace for the AIDA detector description toolkit.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor)=0
Loop over collection and extract hit data.
virtual bool GotoEvent(long event_number)=0
Goto a specified event in the file.
virtual size_t collectionLoop(const std::string &collection, DDEveParticleActor &actor)=0
Loop over collection and extract particle data.
virtual long numEvents() const =0
Access the number of events on the current input data source (-1 if no data source connected)
virtual ~EventHandler()
Default destructor.
Data structure to store the MC particle information.
std::map< std::string, std::vector< Collection > > TypedEventCollections
Types collection: collections are grouped by type (class name)
EventConsumer()=default
Standard constructor.
virtual const TypedEventCollections & data() const =0
Access the map of simulation data collections.
virtual void setSize(size_t)