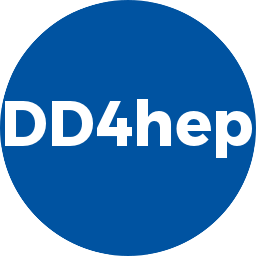 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #include <TEveBrowser.h>
27 #include <TEveManager.h>
28 #include <TEveElement.h>
29 #include <TEveWindow.h>
30 #include <TEveViewer.h>
31 #include <TGLViewer.h>
44 :
PopupMenu(display->client().GetRoot()), m_display(display), m_evtCtrl(0)
46 InstanceCount::increment(
this);
50 DD4hepMenu::~DD4hepMenu() {
62 pm.
menu().DisableEntry(
id);
64 pm.
menu().DisableEntry(
id);
66 pm.
menu().DisableEntry(
id);
68 pm.
menu().DisableEntry(
id);
70 menubar->AddPopup(
"&dd4hep",*
this,
new TGLayoutHints(hints, 0, 4, 0, 0));
75 TGPopupMenu& pm =
menu();
76 pm.DisableEntry(pm.GetEntry(
"Load XML")->GetEntryId());
77 pm.EnableEntry(pm.GetEntry(
"Show Event I/O")->GetEntryId());
78 pm.EnableEntry(pm.GetEntry(
"Open Event Data")->GetEntryId());
79 pm.DisableEntry(pm.GetEntry(
"Next Event")->GetEntryId());
80 pm.DisableEntry(pm.GetEntry(
"Previous Event")->GetEntryId());
86 if ( !fname.empty() ) {
95 if ( !fname.empty() ) {
104 browser->StartEmbedding(TRootBrowser::kLeft);
107 browser->SetTabTitle(
"Evt I/O", 0);
108 browser->StopEmbedding();
109 menu().DisableEntry(
menu().GetEntry(
"Show Event I/O")->GetEntryId());
119 TGPopupMenu& pm =
menu();
120 pm.EnableEntry(pm.GetEntry(
"Open Event Data")->GetEntryId());
121 pm.EnableEntry(pm.GetEntry(
"Next Event")->GetEntryId());
122 pm.EnableEntry(pm.GetEntry(
"Previous Event")->GetEntryId());
139 printout(INFO,
"DDEve",
"+++ The life of this display instance ended.... good bye!");
140 gSystem->Exit(0,kTRUE);
The main class of the DDEve display.
std::string OpenEventFileDialog(const std::string &default_dir) const
Popup ROOT file chooser. returns chosen file name; empty on cancel.
virtual bool Open()
Open a new event data file.
virtual bool NextEvent() override
Load the next event.
std::string OpenXmlFileDialog(const std::string &default_dir) const
Popup XML file chooser. returns chosen file name; empty on cancel.
virtual bool PreviousEvent() override
User overloadable function: Load the previous event.
static void decrement(T *)
Decrement count according to type information.
void LoadXML(const char *xmlFile)
Load geometry from compact xml file.
Event input control for DDEve: Interface class for event I/O.
virtual void Build()
Build the control.
void LoadGeometryRoot(const char *rootFile)
Load geometry from compact xml file.
GenericEventHandler & eventHandler() const
Access to the event reader.
Namespace for the AIDA detector description toolkit.
TEveManager & manager() const
Access to the EVE manager.