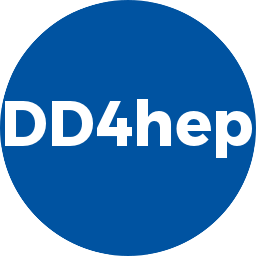 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDEVE_UTILITIES_H
14 #define DDEVE_UTILITIES_H
20 #include "TGeoMatrix.h"
21 #include "TEveElement.h"
28 int findNodeWithMatrix(TGeoNode* p, TGeoNode* n, TGeoHMatrix* mat, std::string* sub_path=0);
30 std::pair<bool,TEveElement*>
31 createEveShape(
int level,
int max_level, TEveElement* p, TGeoNode* n,
const
32 TGeoHMatrix& mat,
const std::string& node_name);
34 std::pair<bool,TEveElement*>
45 template <
typename T>
inline const char*
GetName(T* p) {
47 return n ? n->GetName() :
"???";
51 #endif // DDEVE_UTILITIES_H
int findNodeWithMatrix(TGeoNode *p, TGeoNode *n, TGeoHMatrix *mat, std::string *sub_path=0)
void SetRnrChildren(TEveElementList *l, bool b)
Set the rendering flags for the object and the next level children.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::pair< bool, TEveElement * > createEveShape(int level, int max_level, TEveElement *p, TGeoNode *n, const TGeoHMatrix &mat, const std::string &node_name)
void SetRnrAll(TEveElementList *l, bool b)
Recursively set the rendering flags for the object ans its children.
Handle class describing a detector element.
const char * GetName(T *p)
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::pair< bool, TEveElement * > LoadDetElement(DetElement element, int levels, TEveElement *parent)
Namespace for the AIDA detector description toolkit.
void MakeNodesVisible(TEveElement *e, bool visible, int level)
Make a set of nodes starting from a top element (in-)visible with a given depth.