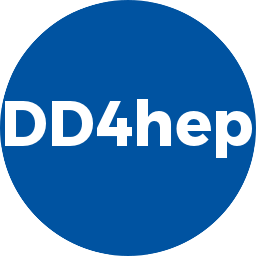 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
36 GenericEventHandler::~GenericEventHandler() {
45 throw std::runtime_error(
"Invalid event handler");
101 std::size_t idx = file_name.find(
"lcio");
102 std::size_t idr = file_name.find(
"root");
109 if ( file_type.find(
"FCC") != std::string::npos ) {
110 m_current = (
EventHandler*)PluginService::Create<void*>(
"DD4hep_DDEve_FCCEventHandler",(
const char*)0);
113 else if ( idx != std::string::npos ) {
114 m_current = (
EventHandler*)PluginService::Create<void*>(
"DD4hep_DDEve_LCIOEventHandler",(
const char*)0);
116 else if ( idr != std::string::npos ) {
117 m_current = (
EventHandler*)PluginService::Create<void*>(
"DD4hep_DDEve_DDG4EventHandler",(
const char*)0);
120 throw std::runtime_error(
"Attempt to open file:"+file_name+
" of unknown type:"+file_type);
128 err =
"+++ Failed to open the data file:"+file_name;
132 err =
"+++ Failed to create fikle reader for file '"+file_name+
"' of type '"+file_type+
"'";
136 err =
"\nAn exception occurred \n"
137 "while opening event data:\n" + std::string(e.what()) +
"\n\n";
139 std::string path = TString::Format(
"%s/stop_t.xpm", TROOT::GetIconPath().Data()).Data();
140 const TGPicture* pic = gClient->GetPicture(path.c_str());
141 new TGMsgBox(gClient->GetRoot(),0,
"Failed to open event data",err.c_str(),pic,
142 kMBDismiss,0,kVerticalFrame,kTextLeft|kTextCenterY);
157 throw std::runtime_error(
"+++ EventHandler::readEvent: No file open!");
160 std::string path = TString::Format(
"%s/stop_t.xpm", TROOT::GetIconPath().Data()).Data();
161 std::string err =
"\nAn exception occurred \n"
162 "while reading a new event:\n" + std::string(e.what()) +
"\n\n";
163 const TGPicture* pic = gClient->GetPicture(path.c_str());
164 new TGMsgBox(gClient->GetRoot(),0,
"Failed to read event", err.c_str(),pic,
165 kMBDismiss,0,kVerticalFrame,kTextLeft|kTextCenterY);
virtual CollectionType collectionType(const std::string &collection) const =0
Access to the collection type by name.
Event data actor base class for particles. Used to extract data from concrete classes.
Event handler base class. Interface to all DDEve I/O actions.
bool m_hasEvent
Flag to indicate that an event is loaded.
Event handler base class: Interface to all DDEve I/O actions.
void exception(const std::string &src, const std::string &msg)
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor) override
Loop over collection and extract data.
ClassImp(GenericEventHandler) GenericEventHandler
Standard constructor.
virtual void Subscribe(EventConsumer *display)
Subscribe to notification of new data present.
GenericEventHandler()
Standard constructor.
Event data actor base class for hits. Used to extract data from concrete classes.
virtual std::string datasourceName() const override
Access the data source name.
virtual bool Open(const std::string &type, const std::string &file_name)=0
Open a new event data file.
virtual std::string datasourceName() const =0
Access the data source name.
virtual bool hasEvent() const
Check if an event is present in memory.
virtual void NotifySubscribers(void(EventConsumer::*pmf)(EventHandler &))
Notfy all subscribers.
bool m_hasFile
Flag to indicate that a file is opened.
virtual bool NextEvent() override
Load the next event.
Event event consumer base class for DDEve: Interface class for event I/O.
virtual void OnNewEvent(EventHandler &)=0
Consumer event data callback.
virtual bool GotoEvent(long event_number) override
Goto a specified event in the file.
Subscriptions m_subscriptions
Data subscriptions (unordered)
virtual bool PreviousEvent() override
User overloadable function: Load the previous event.
virtual void OnFileOpen(EventHandler &)=0
Consumer file open callback.
EventHandler * current() const
virtual CollectionType collectionType(const std::string &collection) const override
Access to the collection type by name.
virtual bool Open(const std::string &type, const std::string &file_name) override
Open a new event data file.
virtual long numEvents() const override
Access the number of events on the current input data source (-1 if no data source connected)
Namespace for the AIDA detector description toolkit.
virtual size_t collectionLoop(const std::string &collection, DDEveHitActor &actor)=0
Loop over collection and extract hit data.
virtual long numEvents() const =0
Access the number of events on the current input data source (-1 if no data source connected)
virtual void Unsubscribe(EventConsumer *display)
Unsubscribe from notification of new data present.