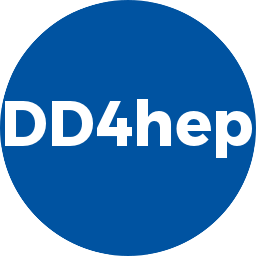 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
58 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
59 except(
"ConditionUpdateCall:",
"Failed to access non-existing condition:"+key_value.name);
62 except(
"ConditionUpdateCall:",
"Failed to access non-existing condition with key [%08X %08X]:",
76 throw std::runtime_error(
"ConditionUpdateCall: Failed to access non-existing condition.");
81 bool throw_if_not)
const {
88 else if ( throw_if_not ) {
89 throw std::runtime_error(
"ConditionUpdateCall: Failed to access non-existing condition.");
120 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
121 except(
"ConditionUpdateCall",
122 "%s [%016llX]: FAILED to access non-existing item:%s [%016llX]",
124 key_value.name.c_str(), key_value.
hash);
128 except(
"ConditionUpdateCall",
129 "Derived condition [%08X %08X]: FAILED to access non-existing item:%s [%08X %08X]",
137 std::shared_ptr<ConditionUpdateCall> call)
138 : m_refCount(0), detector(de), target(de, item_key), callback(
std::move(call))
145 const std::string& item,
146 std::shared_ptr<ConditionUpdateCall> call)
148 detector(de), target(de, item), callback(
std::move(call))
167 unsigned int item_key,
168 std::shared_ptr<ConditionUpdateCall> call)
175 const std::string& item,
176 std::shared_ptr<ConditionUpdateCall> call)
191 except(
"Dependency",
"++ Invalid object. No further source may be added!");
199 except(
"Dependency",
"++ Invalid object. Cannot access built objects!");
void iov_intersection(const IOV &comparator)
Set the intersection of this IOV with the argument IOV.
virtual ~ConditionDependency()
Default destructor.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
AlignmentCondition::Object * cond
virtual ~ConditionResolver()
Standard destructor.
virtual Condition get(const ConditionKey &key)=0
Interface to access conditions by conditions key.
ConditionResolver * resolver
Internal reference to the resolver to access other conditions (Be careful)
Condition::key_type hash
Hashed key representation.
void accessFailure(const ConditionKey &key_value) const
Throw exception on conditions access failure.
virtual std::vector< Condition > getByItem(Condition::itemkey_type key)=0
Interface to access conditions by hash value of the item (only valid at resolve!)
ConditionKey target
Key to the condition to be updated.
DetElement world() const
Access to the top level detector element.
bool isValid() const
Check the validity of the object held by the handle.
ConditionDependency * release()
Release the created dependency and take ownership.
static void increment(T *)
Increment count according to type information.
ConditionUpdateCall()
Standard destructor.
IOV * iov
The reference to the combined IOV resulting from the cumputation.
std::unique_ptr< ConditionDependency > m_dependency
The created dependency.
virtual bool registerOne(const IOV &iov, Condition cond)=0
Interface to handle multi-condition inserts by callbacks: One single insert.
Key definition to optimize ans simplyfy the access to conditions entities.
const ConditionKey & key(size_t which) const
Access to dependency keys.
Helper union to interprete conditions keys.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
Namespace for implementation details of the AIDA detector description toolkit.
Condition dependency definition.
Class describing the interval of validty.
size_t registerMany(const IOV &iov, const std::vector< Condition > &values)
Handle multi-condition inserts by callbacks: block insertions of conditions with identical IOV.
Condition condition(const ConditionKey &key_value) const
Access to condition object by dependency key.
Handle class describing a detector element.
virtual size_t registerMany(const IOV &iov, const std::vector< Condition > &values)=0
Handle multi-condition inserts by callbacks: block insertions of conditions with identical IOV.
static void decrement(T *)
Decrement count according to type information.
virtual ~ConditionUpdateUserContext()
Default destructor.
bool registerOne(const IOV &iov, Condition cond)
Interface to handle multi-condition inserts by callbacks: One single insert.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
virtual ~DependencyBuilder()
Default destructor.
unsigned long long int key_type
Forward definition of the key type.
virtual ~ConditionUpdateCall()
Standard destructor.
void add(const ConditionKey &source_key)
Add a new dependency.
Condition::detkey_type det_key
Namespace for the AIDA detector description toolkit.
virtual Detector & detectorDescription() const =0
Access to the detector description instance.
Condition::itemkey_type item_key() const
Access the detector element part of the key.
DependencyBuilder(DetElement de, Condition::itemkey_type item_key, std::shared_ptr< ConditionUpdateCall > call)
Initializing constructor.
const ConditionDependency * dependency
The dependency to be handled within this context.
struct dd4hep::ConditionKey::KeyMaker::@2 values
std::vector< Condition > getByItem(Condition::itemkey_type key) const
Access conditions by the condition item key.
const IOV & iov() const
Access the IOV block.
ConditionDependency()
Default constructor.
Condition::itemkey_type item_key
std::vector< Condition > conditions(DetElement det) const
Access to all conditions of a detector element.