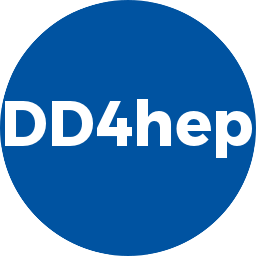 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
22 #include <TGeoManager.h>
36 dd4hep::except(
"GlobalAlignmentCache",
"DetElement cannot determine detector parent [Invalid handle]");
42 : m_detDesc(description), m_sdPath(sdPath), m_sdPathLen(sdPath.length()), m_refCount(1), m_top(top)
48 int nentries = (int)
m_cache.size();
52 printout(INFO,
"GlobalAlignmentCache",
53 "Destroy cache for subdetector %s [%d section(s), %d entrie(s)]",
91 TGeoPhysicalNode* pn = alignment.
ptr();
92 unsigned int index = detail::hash32(pn->GetName()+
m_sdPathLen);
93 Cache::const_iterator i =
m_cache.find(index);
94 printout(ALWAYS,
"GlobalAlignmentCache",
"Section: %s adding entry: %s",
95 name().c_str(),alignment->GetName());
105 std::size_t idx, idq;
106 if ( path_name[0] !=
'/' ) {
109 else if ( (idx=path_name.find(
'/',1)) == std::string::npos ) {
115 if ( (idq=path_name.find(
'/',idx+1)) != std::string::npos ) --idq;
116 std::string path = path_name.substr(idx+1,idq-idx);
117 SubdetectorAlignments::const_iterator j =
m_detectors.find(path);
123 std::size_t idx, idq;
124 unsigned int index = detail::hash32(path_name.c_str()+
m_sdPathLen);
125 Cache::const_iterator i =
m_cache.find(index);
132 else if ( path_name[0] !=
'/' ) {
135 else if ( (idx=path_name.find(
'/',1)) == std::string::npos ) {
139 if ( (idq=path_name.find(
'/',idx+1)) != std::string::npos ) --idq;
140 std::string path = path_name.substr(idx+1, idq-idx);
141 SubdetectorAlignments::const_iterator j =
m_detectors.find(path);
142 if ( j !=
m_detectors.end() )
return (*j).second->get(path_name);
147 std::vector<GlobalAlignment>
149 std::vector<GlobalAlignment> result;
152 std::size_t len = match.length();
153 result.reserve(c->
m_cache.size());
154 for(Cache::const_iterator i=c->
m_cache.begin(); i!=c->
m_cache.end();++i) {
155 const Cache::value_type&
v = *i;
156 const char* n =
v.second->GetName();
157 if ( 0 == ::strncmp(n,match.c_str(),len) ) {
158 if ( exclude_exact && len == ::strlen(n) )
continue;
169 mgr.UnlockGeometry();
176 SubdetectorAlignments::const_iterator i =
m_detectors.find(nam);
187 typedef std::map<std::string,DetElement> DetElementUpdates;
188 typedef std::map<DetElement,std::vector<Entry*> > sd_entries_t;
190 DetElementUpdates detelt_updates;
193 while(stack.
size() > 0) {
196 all[
det].emplace_back(e);
201 for(sd_entries_t::iterator i=all.begin(); i!=all.end(); ++i) {
204 sd_cache->
apply( (*i).second );
208 printout(INFO,
"GlobalAlignmentCache",
"Alignments were applied. Refreshing physical nodes....");
209 mgr.GetCurrentNavigator()->ResetAll();
210 mgr.GetCurrentNavigator()->BuildCache();
211 mgr.RefreshPhysicalNodes();
214 for(DetElementUpdates::iterator i=detelt_updates.begin(); i!=detelt_updates.end(); ++i) {
216 printout(DEBUG,
"GlobalAlignmentCache",
"+++ Trigger placement update for %s [2]",elt.
path().c_str());
220 std::string last_path =
"?????";
221 for(DetElementUpdates::iterator i=detelt_updates.begin(); i!=detelt_updates.end(); ++i) {
222 const std::string& path = (*i).first;
223 if ( path.find(last_path) == std::string::npos ) {
225 printout(DEBUG,
"GlobalAlignmentCache",
"+++ Trigger placement update for %s [1]",elt.
path().c_str());
227 last_path = (*i).first;
231 for(sd_entries_t::iterator i=all.begin(); i!=all.end(); ++i) {
233 printout(DEBUG,
"GlobalAlignmentCache",
"+++ Trigger placement update for %s [0]",elt.
path().c_str());
240 std::map<std::string,std::pair<TGeoPhysicalNode*,Entry*> > nodes;
248 for_each(changes.begin(),changes.end(),selector.
reset());
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
static void uninstall(Detector &description)
Unregister and delete a tree instance.
Definition of the extension entry interface class.
DetElement detector
Reference to the detector element.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
static bool hasMatrix(const StackEntry &e)
Check if this alignment entry has a non unitary transformation matrix.
Alignment Stack object definition.
Main handle class to hold a TGeo alignment object of type TGeoPhysicalNode.
DetElement parent() const
Access to the detector elements's parent.
Act on selected alignment entries.
std::vector< GlobalAlignment > matches(const std::string &path_match, bool exclude_exact=false) const
Return all entries matching a given path. Careful: Expensive operaton!
int release()
Release object. If reference count goes to NULL, automatic deletion is triggered.
Cache m_cache
The subdetector specific map of alignments caches.
int addRef()
Add reference count.
bool isValid() const
Check the validity of the object held by the handle.
size_t size() const
Access size of the alignment stack.
virtual void * addUserExtension(unsigned long long int key, ExtensionEntry *entry)=0
Add an extension object to the detector element (low level member function)
size_t m_sdPathLen
The length of the branch name to optimize lookups....
SubdetectorAlignments m_detectors
Cache of subdetectors.
void apply(GlobalAlignmentStack &stack)
Population entry: Apply a complete stack of ordered alignments to the geometry structure.
GlobalAlignmentCache * subdetectorAlignments(const std::string &name)
Retrieve branch cache by name. If not present it will be created.
Handle class describing a detector element.
Implementation class for the object extension mechanism.
Helper namespace to specialize functionality.
void commit(GlobalAlignmentStack &stack)
Close existing transaction stack and apply all alignments.
GlobalAlignment get(const std::string &path) const
Retrieve an alignment entry by its placement path.
const std::string & placementPath() const
Access to the full path to the placed object.
virtual ~GlobalAlignmentCache()
Default destructor.
dd4hep::DetElement _detector(dd4hep::DetElement child)
GlobalAlignmentStack::StackEntry StackEntry
Class caching all known alignment operations for one Detector instance.
static bool needsReset(const StackEntry &e)
Check flag if the node location should be reset.
const GlobalAlignmentSelector & reset() const
GlobalAlignmentCache(Detector &description, const std::string &sdPath, bool top)
Default constructor initializing variables.
T * ptr() const
Access to the held object.
int m_refCount
Reference count.
IFACE * extension(bool alert=true) const
Access extension element by the type.
dd4hep_ptr< StackEntry > pop()
Retrieve an alignment entry of the current stack.
The main interface to the dd4hep detector description package.
GlobalAlignmentCache * section(const std::string &path_name) const
Retrieve the cache section corresponding to the path of an entry.
const std::string & name() const
Access the section name.
Select alignment operations according to certain criteria.
bool insert(GlobalAlignment alignment)
Add a new entry to the cache. The key is the placement path.
Namespace for implementation details of the AIDA detector description toolkit.
static bool resetChildren(const StackEntry &e)
Check flag if the node location and all children should be reset.
IFACE * removeExtension(bool destroy=true)
Remove an existing extension object from the Detector instance. If not destroyed, the instance is ret...
static GlobalAlignmentCache * install(Detector &description)
Create and install a new instance tree.