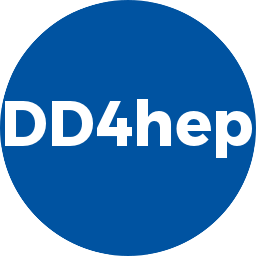 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDALIGN_GLOBALALIGNMENTSTACK_H
14 #define DDALIGN_GLOBALALIGNMENTSTACK_H
91 StackEntry& setResetChildren(
bool new_value=
true);
93 StackEntry& setOverlapCheck(
bool new_value=
true);
95 StackEntry& setOverlapPrecision(
double precision=0.001);
100 typedef std::map<std::string, StackEntry*>
Stack;
146 std::vector<const StackEntry*>
entries()
const;
151 #endif // DDALIGN_GLOBALALIGNMENTSTACK_H
static GlobalAlignmentStack & get()
Static client accessor.
std::map< std::string, StackEntry * > Stack
DetElement detector
Reference to the detector element.
static bool hasMatrix(const StackEntry &e)
Check if this alignment entry has a non unitary transformation matrix.
Alignment Stack object definition.
GlobalAlignmentStack()
Default constructor.
static void create()
Create an alignment stack instance. The creation of a second instance will be refused.
bool insert(const std::string &full_path, dd4hep_ptr< StackEntry > &new_entry)
Add a new entry to the cache. The key is the placement path.
size_t size() const
Access size of the alignment stack.
double overlap
Parameter for overlap checking.
Class describing an condition to re-adjust an alignment.
StackEntry()=delete
Default constructor.
Handle class describing a detector element.
std::vector< const StackEntry * > entries() const
Get all path entries to be aligned. Note: transient!
Delta delta
Delta transformation to be applied.
static bool overlapValue(const StackEntry &e)
Check if the overalp value is present.
static bool checkOverlap(const StackEntry &e)
Check if the overlap flag checking is enabled.
GlobalAlignmentStack::StackEntry StackEntry
StackEntry & operator=(const StackEntry &e)=default
Assignment operator.
static bool needsReset(const StackEntry &e)
Check flag if the node location should be reset.
virtual ~StackEntry()
Default destructor.
static bool overlapDefined(const StackEntry &e)
Check if the overlap flag checking is enabled.
Namespace for the AIDA detector description toolkit.
dd4hep_ptr< StackEntry > pop()
Retrieve an alignment entry of the current stack.
Stack m_stack
The subdetector specific map of alignments caches.
std::string path
Path to the misaligned volume.
bool add(dd4hep_ptr< StackEntry > &new_entry)
Add a new entry to the cache. The key is the placement path.
virtual ~GlobalAlignmentStack()
Default destructor. Careful with this one:
static bool exists()
Check existence of alignment stack.
void release()
Clear data content and remove the slignment stack.
bool checkFlag(unsigned int mask) const
Check a given flag.
static bool resetChildren(const StackEntry &e)
Check flag if the node location and all children should be reset.
Out version of the std auto_ptr implementation base either on auto_ptr or unique_ptr.