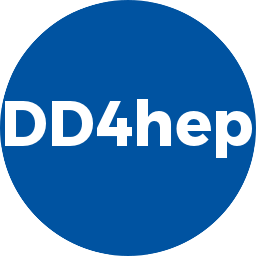 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4GFLASHSHOWERMODEL_H
14 #define DDG4_GEANT4GFLASHSHOWERMODEL_H
20 class GVFlashShowerParameterisation;
21 class G4ParticleDefinition;
22 class GFlashParticleBounds;
23 class GFlashShowerModel;
93 #endif // DDG4_GEANT4GFLASHSHOWERMODEL_H
116 #include <GVFlashShowerParameterisation.hh>
117 #include <GFlashHomoShowerParameterisation.hh>
118 #include <GFlashSamplingShowerParameterisation.hh>
119 #include <GFlashParticleBounds.hh>
120 #include <GFlashShowerModel.hh>
121 #include <GFlashHitMaker.hh>
147 if ( a ) detail::releasePtr(a);
161 detail::releasePtr(a);
169 except(
"The supplied parametrization %s was found as Geant4Action, but is no "
170 "GVFlashShowerParameterisation!", this->
m_paramName.c_str());
180 except(
"Failed to access the region %s from the detector description.", this->
m_regionName.c_str());
182 auto region_iter =
mapping.g4Regions.find(rg);
183 if ( region_iter ==
mapping.g4Regions.end() ) {
184 except(
"Failed to locate G4Region: %s from the Geant4 mapping.", this->
m_regionName.c_str());
186 G4Region* region = (*region_iter).second;
189 auto* shower_model =
new GFlashShowerModel(this->
name(), region);
191 logger <<
"Geant4 shower model initialized with parametrization: ";
194 except(
"No proper parametrization name supplied in the properties: %s",this->
m_paramName.c_str());
196 if ( this->
m_paramName ==
"GFlashHomoShowerParameterisation" ) {
199 logger <<
"GFlashHomoShowerParameterisation Material: " << mat->GetName();
201 else if ( this->
m_paramName ==
"GFlashSamplingShowerParameterisation" ) {
206 logger <<
"GFlashSamplingShowerParameterisation Materials: " << mat1->GetName()
210 auto* action = kernel.globalAction(this->
m_paramName,
false);
212 if ( action )
logger << typeName(
typeid(*action));
219 except(
"No proper parametrization supplied. Failed to construct shower model.");
228 shower_model->SetStepInX0(this->
m_stepX0);
229 shower_model->SetFlagParamType(this->
m_enable ? 1 : 0);
232 for(
const auto& prop : this->
m_eMin) {
236 this->
info(
"SetMinEneToParametrise [%-16s] = %8.4f GeV", prop.first.c_str(), val);
238 for(
const auto& prop : this->
m_eMax) {
242 this->
info(
"SetMaxEneToParametrise [%-16s] = %8.4f GeV", prop.first.c_str(), val);
244 for(
const auto& prop : this->
m_eKill) {
248 this->
info(
"SetEneToKill [%-16s] = %8.4f GeV", prop.first.c_str(), val);
252 this->
info(logger.str().c_str());
ParticleConfig m_eMin
Property: Set minimum kinetic energy to trigger parametrisation.
std::vector< std::string > m_applicablePartNames
Property: Particle names for which this parametrization is applicable.
GVFlashShowerParameterisation * m_parametrization
Reference to the parametrization.
Geant4GeometryInfo & data() const
Access to the data pointer.
int m_particleContainment
Property: Defines if particle containment is checked.
GAUDIPS_API Logger & logger()
Return the current logger instance.
bool isValid() const
Check the validity of the object held by the handle.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
std::string m_material
Property: Material name for GFlashHomoShowerParameterisation.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
GFlashParticleBounds * m_particleBounds
Reference to the particle bounds object.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4GFlashShowerModel)
Define standard assignments and constructors.
void adoptShowerParametrization(Geant4Action *param)
Adopt shower parametrization object.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
Geant4GFlashShowerModel(Geant4Context *context, const std::string &nam)
Standard constructor.
std::string m_regionName
Property: Region name to which this parametrization should be applied.
Handle class describing a region as used in simulation.
Default base class for all Geant 4 actions and derivates thereof.
double m_parameter_1
Property: Parameter 1 name for GFlashSamplingShowerParameterisation.
const std::string & name() const
Access name of the action.
ParticleConfig m_eMax
Property: Set maximum kinetic energy to trigger parametrisation.
G4ParticleDefinition * getParticleDefinition(const std::string &name) const
Access particle definition from string.
double m_parameter_2
Property: Parameter 2 name for GFlashSamplingShowerParameterisation.
double m_stepX0
Property: Defines step lenght.
double _toDouble(const std::string &value)
String conversions: string to double value.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
std::string m_material_2
Property: Material 2 name for GFlashSamplingShowerParameterisation.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
GFlashHitMaker * m_hitMaker
Reference to the hit maker.
void addShowerModel(G4Region *region)
Add shower model to region's fast simulation manager.
Namespace for the AIDA detector description toolkit.
G4Material * getMaterial(const std::string &name) const
Get parametrization material.
G4VFastSimulationModel * m_model
Reference to the G4 fast simulation model.
virtual ~Geant4GFlashShowerModel()
Default destructor.
Geant4 wrapper for the GFlash shower model.
ParticleConfig m_eKill
Property: Set maximum kinetic energy for particles to be killed.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
std::string m_paramName
Property: Name of the Geant4Action implementing the parametrization.
Geant4 wrapper for the Geant4 fast simulation shower model.
bool m_enable
Property: Parametrisation control: Enable/disable fast simulation.