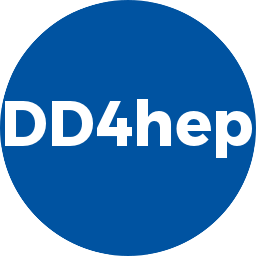 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4FASTSIMSHOWERMODEL_H
14 #define DDG4_GEANT4FASTSIMSHOWERMODEL_H
20 #include <G4FastSimulationPhysics.hh>
30 class G4ParticleDefinition;
31 class G4VFastSimulationModel;
40 class Geant4ShowerModelWrapper;
53 typedef std::map<const G4ParticleDefinition*, double>
ParticleCut;
79 G4VFastSimulationModel*
m_model {
nullptr };
92 G4Region*
getRegion(
const std::string& nam)
const;
96 void killParticle(G4FastStep& step,
double deposit,
double step_length = 0e0);
117 virtual void modelShower(
const G4FastTrack& track, G4FastStep& step);
129 template <
typename CONCRETE_MODEL>
164 virtual bool check_trigger(
const G4FastTrack& track)
override;
167 virtual void modelShower(
const G4FastTrack& track, G4FastStep& step)
override;
171 #endif // DDG4_GEANT4FASTSIMSHOWERMODEL_H
virtual void modelShower(const G4FastTrack &track, G4FastStep &step)
User callback to model the particle/energy shower.
ParticleConfig m_eMin
Property: Set minimum kinetic energy to trigger parametrisation.
std::vector< std::string > m_applicablePartNames
Property: Particle names for which this parametrization is applicable.
virtual void modelShower(const G4FastTrack &track, G4FastStep &step) override
User callback to model the particle/energy shower.
ParticleConfig m_eTriggerNames
Property: Set minimal kinetic energy for particles to trigger the model.
ParticleCut m_eTriggerCut
Particle cut to trigger model simulation indexed by G4ParticleDefinition.
double m_stepX0
Property: Defines step length.
CONCRETE_MODEL locals
Local parameter buffer to specialize caches, properties etc.
Wrapper m_wrapper
Reference to the shower model.
virtual void constructField(Geant4DetectorConstructionContext *ctxt) override
Electromagnetic field construction callback. Called at "ConstructSDandField()".
void initialize()
Declare optional properties from embedded structure.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
Geant4 detector construction context definition.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt) override
Geometry construction callback. Called at "Construct()".
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4FastSimShowerModel)
Define standard assignments and constructors.
Geant4FastSimShowerModel(Geant4Context *context, const std::string &nam)
Standard constructor.
Geant4 wrapper for the Geant4 fast simulation shower model.
std::set< const G4ParticleDefinition * > m_applicableParticles
Particle definitions for which this parametrization is applicable.
Geant4FSShowerModel(Geant4Context *context, const std::string &nam)
Standard constructor.
virtual bool check_trigger(const G4FastTrack &track) override
User callback to determine if the shower creation should be triggered.
std::string m_regionName
Property: Region name to which this parametrization should be applied.
virtual bool check_trigger(const G4FastTrack &track)
User callback to determine if the shower creation should be triggered.
virtual bool check_applicability(const G4ParticleDefinition &particle) override
User callback to determine if the model is applicable for the particle type.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
virtual ~Geant4FastSimShowerModel()
Default destructor.
virtual void constructField(Geant4DetectorConstructionContext *ctxt) override
Electromagnetic field construction callback. Called at "ConstructSDandField()".
virtual bool check_applicability(const G4ParticleDefinition &particle)
User callback to determine if the model is applicable for the particle type.
Geant4ShowerModelWrapper * Wrapper
const std::string & name() const
Access name of the action.
ParticleConfig m_eMax
Property: Set maximum kinetic energy to trigger parametrisation.
G4ParticleDefinition * getParticleDefinition(const std::string &name) const
Access particle definition from string.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt) override
Geometry construction callback. Called at "Construct()".
G4Region * getRegion(const std::string &nam) const
Access the region from the detector description by name.
Geant4 wrapper for the Geant4 fast simulation shower model.
void addShowerModel(G4Region *region)
Add shower model to region's fast simulation manager.
Namespace for the AIDA detector description toolkit.
G4Material * getMaterial(const std::string &name) const
Get parametrization material.
virtual ~Geant4FSShowerModel()
Default destructor.
G4VFastSimulationModel * m_model
Reference to the G4 fast simulation model.
std::map< const G4ParticleDefinition *, double > ParticleCut
Basic implementation of the Geant4 detector construction action.
void killParticle(G4FastStep &step, double deposit, double step_length=0e0)
Kill primary particle when creating the shower.
ParticleConfig m_eKill
Property: Set maximum kinetic energy for particles to be killed.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4 wrapper for the Geant4 fast simulation shower model.
bool m_enable
Property: Parametrisation control: Enable/disable fast simulation.
std::map< std::string, std::string > ParticleConfig