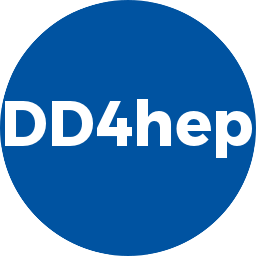 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
20 #include <G4FastSimulationManager.hh>
21 #include <G4VFastSimulationModel.hh>
22 #include <G4TouchableHandle.hh>
23 #include <G4ParticleTable.hh>
24 #include <G4FastStep.hh>
58 virtual G4bool IsApplicable(
const G4ParticleDefinition& particle)
override;
60 virtual G4bool ModelTrigger(
const G4FastTrack& track)
override;
62 virtual void DoIt(
const G4FastTrack& track, G4FastStep& step)
override;
70 : G4VFastSimulationModel(model->name()), m_model(model)
112 G4ParticleTable* pt = G4ParticleTable::GetParticleTable();
113 G4ParticleDefinition* def = pt->FindParticle(particle);
114 if ( def )
return def;
115 except(
"Failed to access Geant4 particle definition: %s", particle.c_str());
122 Material material = kernel.detectorDescription().material(mat_name);
124 auto mat_iter =
mapping.g4Materials.find(material);
125 if ( mat_iter !=
mapping.g4Materials.end() ) {
126 return (*mat_iter).second;
129 except(
"Failed to access shower parametrization material: %s", mat_name.c_str());
137 Region rg = kernel.detectorDescription().region(nam);
139 except(
"Failed to access the region %s from the detector description.", nam.c_str());
141 auto region_iter =
mapping.g4Regions.find(rg);
142 if ( region_iter ==
mapping.g4Regions.end() ) {
143 except(
"Failed to locate G4Region: %s from the Geant4 mapping.", nam.c_str());
145 G4Region* region = (*region_iter).second;
154 except(
"Geant4FastSimShowerModel::addShowerModel: Invalid G4Region reference!");
157 G4FastSimulationManager* fastsimManager = region->GetFastSimulationManager();
158 if ( !fastsimManager ) {
159 fastsimManager =
new G4FastSimulationManager(region,
true);
163 fastsimManager->AddFastSimulationModel(
m_model);
165 fastsimManager->AddFastSimulationModel(
m_wrapper);
167 except(
"Geant4FastSimShowerModel::addShowerModel: Invalid shower model reference!");
190 this->
info(
"Set Energy(ModelTrigger) [%-16s] = %8.4f GeV", prop.first.c_str(), val);
198 step.KillPrimaryTrack();
199 step.ProposePrimaryTrackPathLength(step_length);
200 step.ProposeTotalEnergyDeposited(deposit);
212 auto* prim = track.GetPrimaryTrack();
213 auto* def = prim->GetParticleDefinition();
216 return (*iter).second < prim->GetKineticEnergy();
220 return (*iter).second < prim->GetKineticEnergy();
227 except(
"Method %s::modelShower(const G4FastTrack& track, G4FastStep& step) "
228 "is not implemented. User implementation mandatory.", this->
name().c_str());
virtual void modelShower(const G4FastTrack &track, G4FastStep &step)
User callback to model the particle/energy shower.
ParticleConfig m_eMin
Property: Set minimum kinetic energy to trigger parametrisation.
std::vector< std::string > m_applicablePartNames
Property: Particle names for which this parametrization is applicable.
ParticleConfig m_eTriggerNames
Property: Set minimal kinetic energy for particles to trigger the model.
virtual G4bool ModelTrigger(const G4FastTrack &track) override
User callback to determine if the shower creation should be triggered.
ParticleCut m_eTriggerCut
Particle cut to trigger model simulation indexed by G4ParticleDefinition.
Geant4GeometryInfo & data() const
Access to the data pointer.
double m_stepX0
Property: Defines step length.
Wrapper m_wrapper
Reference to the shower model.
bool isValid() const
Check the validity of the object held by the handle.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
virtual ~Geant4ShowerModelWrapper()=default
Default destructor.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt) override
Sensitive detector construction callback. Called at "ConstructSDandField()".
void info(const char *fmt,...) const
Support of info messages.
Handle class describing a material.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
Geant4 detector construction context definition.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt) override
Geometry construction callback. Called at "Construct()".
Geant4FastSimShowerModel(Geant4Context *context, const std::string &nam)
Standard constructor.
std::set< const G4ParticleDefinition * > m_applicableParticles
Particle definitions for which this parametrization is applicable.
virtual void DoIt(const G4FastTrack &track, G4FastStep &step) override
User callback to model the particle/energy shower.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
std::string m_regionName
Property: Region name to which this parametrization should be applied.
virtual bool check_trigger(const G4FastTrack &track)
User callback to determine if the shower creation should be triggered.
virtual ~Geant4FastSimShowerModel()
Default destructor.
Handle class describing a region as used in simulation.
virtual void constructField(Geant4DetectorConstructionContext *ctxt) override
Electromagnetic field construction callback. Called at "ConstructSDandField()".
virtual bool check_applicability(const G4ParticleDefinition &particle)
User callback to determine if the model is applicable for the particle type.
const std::string & name() const
Access name of the action.
ParticleConfig m_eMax
Property: Set maximum kinetic energy to trigger parametrisation.
G4ParticleDefinition * getParticleDefinition(const std::string &name) const
Access particle definition from string.
virtual G4bool IsApplicable(const G4ParticleDefinition &particle) override
User callback to determine if the model is applicable for the particle type.
G4Region * getRegion(const std::string &nam) const
Access the region from the detector description by name.
double _toDouble(const std::string &value)
String conversions: string to double value.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
Geant4ShowerModelWrapper(Geant4FastSimShowerModel *model)
Initializing constructor.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
Geant4 wrapper for the Geant4 fast simulation shower model.
void addShowerModel(G4Region *region)
Add shower model to region's fast simulation manager.
Namespace for the AIDA detector description toolkit.
G4Material * getMaterial(const std::string &name) const
Get parametrization material.
G4VFastSimulationModel * m_model
Reference to the G4 fast simulation model.
Basic implementation of the Geant4 detector construction action.
Geant4FastSimShowerModel * m_model
Reference to the model wrapper.
void killParticle(G4FastStep &step, double deposit, double step_length=0e0)
Kill primary particle when creating the shower.
ParticleConfig m_eKill
Property: Set maximum kinetic energy for particles to be killed.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4 wrapper for the Geant4 fast simulation shower model.
bool m_enable
Property: Parametrisation control: Enable/disable fast simulation.