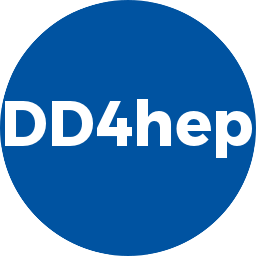 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
15 #include <DD4hep/detail/Handle.inl>
23 #include <TGeoVolume.h>
24 #include <TGeoMatrix.h>
25 #include <TGeoManager.h>
39 verbose(0), combineHits(0), ecut(0.0), readout(), region(), limits(), hitsCollection() {
40 printout(VERBOSE,
"SensitiveDetectorObject",
"+++ Created new anonymous SensitiveDetectorObject()");
47 verbose(0), combineHits(0), ecut(0.0), readout(), region(), limits(), hitsCollection() {
49 printout(VERBOSE,
"SensitiveDetectorObject",
"+++ Created new SensitiveDetectorObject('%s')",nam.c_str());
65 flag(0), id(0), combineHits(0), typeFlag(0), level(-1),
key(0), path(),
placementPath(),
66 idealPlace(), placement(), volumeID(0), parent(), children(),
69 printout(VERBOSE,
"DetElementObject",
"+++ Created new anonymous DetElementObject()");
76 flag(0), id(ident), combineHits(0), typeFlag(0), level(-1),
key(0), path(),
placementPath(),
77 idealPlace(), placement(), volumeID(0), parent(), children(),
81 printout(VERBOSE,
"DetElementObject",
"+++ Created new DetElementObject('%s', %d)",nam.c_str(),
id);
115 obj->ObjectExtensions::clear();
116 obj->ObjectExtensions::copyFrom(
extensions, obj);
130 except(
"DetElement",
"+++ DetElement::copy: Element %s is already "
131 "present [Double-Insert]", c.
name());
140 std::map<TGeoNode*,TGeoNode*> nodes;
143 printout(DEBUG,
"DetElement",
"reflect: Match %s %p ",de_det.
name(), de_det.
placement().
ptr());
144 if ( k == nodes.end() ) {
145 except(
"DetElement",
"reflect: Something went wrong when reflecting the source volume!");
148 const auto& childrens_det = de_det.
children();
149 const auto& childrens_ref = de_ref.
children();
150 for(
auto i=childrens_det.begin(), j=childrens_ref.begin(); i!=childrens_det.end(); ++i, ++j)
151 match((*i).second, (*j).second);
153 void map(TGeoNode* n1, TGeoNode* n2) {
154 if ( nodes.find(n1) == nodes.end() ) {
155 TGeoVolume* v1 = n1->GetVolume();
156 TGeoVolume* v2 = n2->GetVolume();
157 nodes.insert(std::make_pair(n1,n2));
158 printout(DEBUG,
"DetElement",
"reflect: Map %p --- %p ",n1,n2);
159 for(Int_t i=0; i<v1->GetNdaughters(); ++i)
160 map(v1->GetNode(i), v2->GetNode(i));
167 TGeoVolume* vol_det = vol.
ptr();
168 TGeoVolume* vol_ref = vol.
reflect(sd);
169 const auto& childrens_det =
det.children();
170 const auto& childrens_ref = det_ref.
children();
172 for(Int_t i=0; i<vol_det->GetNdaughters(); ++i)
173 mapper.map(vol_det->GetNode(i), vol_ref->GetNode(i));
174 for(
auto i=childrens_det.begin(), j=childrens_ref.begin(); i!=childrens_det.end(); ++i, ++j)
175 mapper.match((*i).second, (*j).second);
176 return std::make_pair(det_ref,vol_ref);
198 std::string place =
det.placementPath();
203 except(
"DetElement",
"The placement %s is not part of the hierarchy.",place.c_str());
206 "DetElement",
"+++ Invalidate chache of %s -> %s Placement:%p --> %p %s",
211 node->SetUserExtension(ext);
215 if ( node_vol.
ptr() != plac_vol.
ptr() && 0 == node_vol->GetUserExtension() ) {
216 auto ext = plac_vol->GetUserExtension();
217 node_vol->SetUserExtension(ext);
223 i.second->revalidate();
229 if ( (typ&((*i).second)) == typ && (*i).first.par == pointer ) {
239 const void* args[3] = { (
void*)((
unsigned long)tags),
this, param };
242 printout(INFO,
"DetElement",
243 "++ Need to update local and child caches of %s",
248 if ( (tags&i.second) )
249 i.first.execute(args);
Handle class to hold the information of the top DetElement object 'world'.
const Children & children() const
Access to the list of children.
virtual ~SensitiveDetectorObject()
Internal object destructor: release extension object(s)
DetElement::Children children
The array of children.
void destroyHandle(T &handle)
Helper to delete objects from heap and reset the handle.
Data class with properties of a detector element.
Handle class to hold the information of a sensitive detector.
Implementation of an object supporting arbitrary user extensions.
unsigned int typeFlag
Flag to encode detector types.
Main handle class to hold an alignment conditions object.
Handle class holding a placed volume (also called physical volume)
const char * GetName() const
Access name.
PlacedVolume placement() const
Access to the physical volume of this detector element.
PlacedVolume idealPlace
The subdetector placement corresponding to the ideal detector element's volume.
bool isValid() const
Check the validity of the object held by the handle.
static void increment(T *)
Increment count according to type information.
unsigned long long int magic_word()
Access to the magic word, which is protecting some objects against memory corruptions.
void removeAtUpdate(unsigned int type, void *pointer)
Remove callback from object.
const char * name() const
Access the object name (or "" if not supported by the object)
Data class with properties of a detector element.
void SetName(const char *nam)
Set name (used by Handle)
AlignmentCondition survey
Basic detector element alignment entry containing the survey data.
Handle< T > & clear()
Release the object held by the handle.
DD4HEP_INSTANTIATE_HANDLE_NAMED(DetElementObject)
DetElement parent
Reference to the parent element.
void revalidate()
Revalidate the caches.
World privateWorld
Reference to the parent element.
Handle class describing a detector element.
std::map< unsigned long long int, ExtensionEntry * > extensions
The extensions object.
Handle class holding a placed volume (also called physical volume)
World i_access_world()
Resolve the world object. Internal use ONLY.
unsigned int flag
Flag to remember internally calculated quatities.
Object & _data() const
Additional data accessor.
static void decrement(T *)
Decrement count according to type information.
void destroyHandles(M &arg)
Functional created of map destruction functors.
int level
Hierarchical level within the detector description.
WorldObject()
Not persistent in ROOT.
virtual ~WorldObject()
Internal object destructor: release extension object(s)
PlacedVolume placement
The subdetector placement corresponding to the actual detector element's volume.
std::size_t verbose(const std::string &src, const std::string &msg)
detail::tools::ElementPath ElementPath
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
const char * GetTitle() const
Get name (used by Handle)
int id
Unique integer identifier of the detector instance.
std::pair< DetElement, Volume > reflect(const std::string &new_name, int new_id, SensitiveDetector sd)
Reflect all volumes in a DetElement sub-tree and re-attach the placements.
UpdateCallbacks updateCalls
Placeholder for structure with update callbacks.
World world()
Access to the world object. Only possible once the geometry is closed.
virtual ~DetElementObject()
Internal object destructor: release extension object(s)
T * ptr() const
Access to the held object.
void clear(bool destroy=true)
Clear all extensions.
Data class with properties of sensitive detectors.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
std::string placementPath
The path to the placement of the detector element (if placed)
Volume volume() const
Logical volume of this placement.
int combineHits
Flag to process hits.
The main interface to the dd4hep detector description package.
Volume reflect() const
Create a reflected volume tree. The reflected volume has left-handed coordinates.
DetElementObject()
Default constructor.
SensitiveDetectorObject()
Default constructor.
AlignmentCondition nominal
Not ROOT persistent.
virtual DetElementObject * clone(int new_id, int flag) const
Deep object copy to replicate DetElement trees e.g. for reflection.
detail::tools::PlacementPath PlacementPath
void update(unsigned int tags, void *param)
Trigger update callbacks.
unsigned int key
Access hash key of this detector element (Only valid once geometry is closed!)