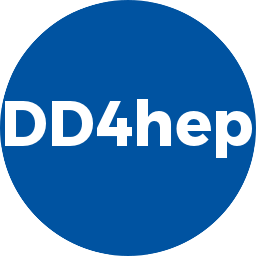 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #include <TGeoMatrix.h>
57 const std::vector<GlobalAlignment>& vol_alignments =
det.volumeAlignments();
58 for(
const auto& alignment : vol_alignments ) {
60 e.setAttr(
_ALU(path), alignment->GetName());
69 TGeoNode* n = a->GetNode();
70 TGeoHMatrix mat(a->GetOriginalMatrix()->Inverse());
71 mat.Multiply(n->GetMatrix());
72 const Double_t* t = mat.GetTranslation();
77 printout(INFO,
"GlobalAlignmentWriter",
"Write Delta constants for %s",a->GetName());
88 if ( mat.IsRotation() ) {
112 for (DetElement::Children::const_iterator i = c.begin(); i != c.end(); ++i) {
115 (elt ? (elt) : (elt=
createElement(doc,element))).append(daughter);
129 if ( (elt=
scan(doc,top)) ) elements.append(elt);
137 return docH.
output(doc, output);
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
Document create(const char *tag, const char *comment=0) const
Create new XML document by parsing empty xml buffer.
Document document() const
Access the hosting document handle of this DOM element.
GlobalDetectorAlignment. DetElement Handle supporting alignment operations.
void append(Handle_t handle) const
Append a new element to the existing tree.
Main handle class to hold a TGeo alignment object of type TGeoPhysicalNode.
GlobalAlignmentCache * m_cache
Reference to the alignment cache.
ROOT::Math::XYZVector XYZAngles
int release()
Release object. If reference count goes to NULL, automatic deletion is triggered.
long write(xml::Document doc, const std::string &output) const
Write the XML document structure to a file.
int addRef()
Add reference count.
bool isValid() const
Check the validity of the object held by the handle.
std::string _toString(bool value)
String conversions: boolean value to string.
Handle class describing a detector element.
xml::Document dump(DetElement element, bool enable_transactions=false) const
Dump one full DetElement subtree into a newly created document.
Namespace for the AIDA detector description toolkit supporting XML utilities.
const std::string & placementPath() const
Access to the full path to the placed object.
static std::string defaultComment()
Default comment string.
XYZAngles _xyzAngles(const double *matrix)
Convert a 3x3 rotation matrix to XYZAngles.
Class supporting the basic functionality of an XML document.
Class caching all known alignment operations for one Detector instance.
virtual int output(Document doc, const std::string &fname) const
Write xml document to output file (stdout if file name empty)
std::map< std::string, DetElement > Children
Class supporting to read and parse XML documents.
User abstraction class to manipulate XML elements within a document.
IFACE * extension(bool alert=true) const
Access extension element by the type.
virtual ~GlobalAlignmentWriter()
Standard destructor.
Attribute setAttr(const XmlChar *nam, const T &val) const
Set single attribute.
GlobalAlignmentWriter(Detector &description)
Initializing Constructor.
The main interface to the dd4hep detector description package.
xml::Element scan(xml::Document doc, DetElement element) const
Scan one DetElement structure and return an XML element containing the alignment in this subtree.
xml::Element createElement(xml::Document doc, DetElement element) const
Create the element corresponding to one single detector element without children.
void addNode(xml::Element elt, GlobalAlignment a) const
Add single alignment node to the XML document.
Namespace for implementation details of the AIDA detector description toolkit.
Handle_t root() const
Access the ROOT eleemnt of the DOM document.