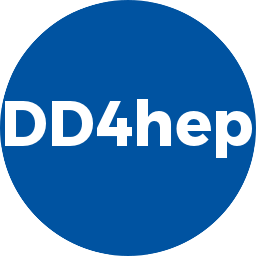 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDALIGN_GLOBALALIGNMENTCACHE_H
14 #define DDALIGN_GLOBALALIGNMENTCACHE_H
28 class GlobalAlignmentOperator;
29 class GlobalAlignmentCache;
30 class GlobalAlignmentStack;
49 typedef std::map<unsigned int, TGeoPhysicalNode*>
Cache;
80 void apply(
const std::vector<Entry*> &changes);
102 std::vector<GlobalAlignment>
matches(
const std::string& path_match,
bool exclude_exact=
false)
const;
107 #endif // DDALIGN_GLOBALALIGNMENTCACHE_H
static void uninstall(Detector &description)
Unregister and delete a tree instance.
Alignment Stack object definition.
Main handle class to hold a TGeo alignment object of type TGeoPhysicalNode.
Base class for alignment functors.
std::vector< GlobalAlignment > matches(const std::string &path_match, bool exclude_exact=false) const
Return all entries matching a given path. Careful: Expensive operaton!
int release()
Release object. If reference count goes to NULL, automatic deletion is triggered.
Cache m_cache
The subdetector specific map of alignments caches.
int addRef()
Add reference count.
size_t m_sdPathLen
The length of the branch name to optimize lookups....
SubdetectorAlignments m_detectors
Cache of subdetectors.
void apply(GlobalAlignmentStack &stack)
Population entry: Apply a complete stack of ordered alignments to the geometry structure.
GlobalAlignmentCache * subdetectorAlignments(const std::string &name)
Retrieve branch cache by name. If not present it will be created.
Implementation class for the object extension mechanism.
void commit(GlobalAlignmentStack &stack)
Close existing transaction stack and apply all alignments.
GlobalAlignment get(const std::string &path) const
Retrieve an alignment entry by its placement path.
GlobalAlignmentStack Stack
virtual ~GlobalAlignmentCache()
Default destructor.
bool m_top
Flag to indicate the top instance.
Class caching all known alignment operations for one Detector instance.
GlobalAlignmentCache(Detector &description, const std::string &sdPath, bool top)
Default constructor initializing variables.
int m_refCount
Reference count.
std::map< std::string, GlobalAlignmentCache * > SubdetectorAlignments
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
GlobalAlignmentCache * section(const std::string &path_name) const
Retrieve the cache section corresponding to the path of an entry.
const std::string & name() const
Access the section name.
std::map< unsigned int, TGeoPhysicalNode * > Cache
bool insert(GlobalAlignment alignment)
Add a new entry to the cache. The key is the placement path.
static GlobalAlignmentCache * install(Detector &description)
Create and install a new instance tree.