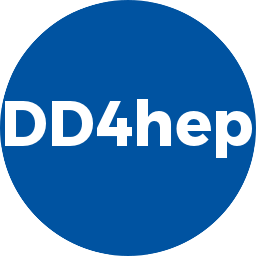 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
29 #define BOOST_BIND_GLOBAL_PLACEHOLDERS
42 #include <boost/property_tree/json_parser.hpp>
51 static void setChildTitles(
const std::pair<std::string, DetElement>& e) {
54 if (::strlen(e.second->GetTitle()) == 0) {
55 e.second->SetTitle(parent.
isValid() ? parent.
type().c_str() : e.first.c_str());
57 for_each(children.begin(), children.end(), setChildTitles);
61 template <>
void Converter<detector>::operator()(
json_h element)
const;
65 template <>
void Converter<detector>::operator()(
json_h element)
const {
66 std::string type = element.
attr<std::string>(
_U(type));
67 std::string name = element.
attr<std::string>(
_U(name));
68 std::string name_match =
":" + name +
":";
69 std::string type_match =
":" + type +
":";
77 std::string par_name = element.
attr<std::string>(attr_par);
78 DetElement parent_detector = description.detector(par_name);
79 if ( !parent_detector.isValid() ) {
80 except(
"Compact",
"Failed to access valid parent detector of %s",name.c_str());
82 description.declareParent(name, parent_detector);
88 Readout ro = description.readout(element.
attr<std::string>(attr_ro));
90 throw std::runtime_error(
"No Readout structure present for detector:" + name);
96 description.addSensitiveDetector(sd);
99 DetElement det(PluginService::Create<NamedObject*>(type, &description, &element, &sens));
101 setChildTitles(std::make_pair(name,
det));
110 printout(
det.isValid() ? INFO : ERROR,
"Compact",
"%s subdetector:%s of type %s %s",
111 (
det.isValid() ?
"++ Converted" :
"FAILED "), name.c_str(), type.c_str(),
112 (sd.
isValid() ? (
"[" + sd.
type() +
"]").c_str() :
""));
114 if (!
det.isValid()) {
116 PluginService::Create<NamedObject*>(type, &description, &element, &sens);
117 throw std::runtime_error(
"Failed to execute subdetector creation plugin. " + dbg.
missingFactory(type));
119 description.addDetector(
det);
123 printout(ERROR,
"Compact",
"++ FAILED to convert subdetector: %s: %s", name.c_str(), e.what());
127 printout(ERROR,
"Compact",
"++ FAILED to convert subdetector: %s: %s", name.c_str(),
"UNKNONW Exception");
132 static long handle_json(
Detector& description,
int argc,
char** argv) {
133 if ( argc < 1 || (argc<2 && argv[0][0] !=
'/') ) {
134 ::printf(
"DD4hep_JsonProcessor <file> <directory> \n"
135 " If file is an absolute path (does NOT start with '/')\n"
136 " the directory path is mandatory. \n"
137 " The file name is then assumed to be relative. \n"
141 std::string file = argv[0];
142 if ( file[0] !=
'/' ) file = std::string(argv[1]) +
"/" + file;
144 printout(INFO,
"JsonProcessor",
"++ Processing JSON input: %s",file.c_str());
149 printout(INFO,
"JsonProcessor",
"++ ... Successfully processed JSON input: %s",file.c_str());
const Children & children() const
Access to the list of children.
Handle class to hold the information of a sensitive detector.
DetElement parent() const
Access to the detector elements's parent.
void exception(const std::string &src, const std::string &msg)
std::string type() const
Access detector type (structure, tracker, calorimeter, etc.).
T attr(const Attribute a) const
Access typed attribute value by the JsonAttr.
SensitiveDetector & setHitsCollection(const std::string &spec)
Assign the name of the hits collection.
Handle< SensitiveDetectorObject > sensitive() const
Access the sensitive detector using this segmentation object.
#define DECLARE_APPLY(name, func)
bool isValid() const
Check the validity of the object held by the handle.
Handle< DetElementObject > detector() const
Access the main detector element using this segmentation object.
Class supporting to read and parse XML documents.
const char * name() const
Access the object name (or "" if not supported by the object)
Attribute attr_nothrow(const char *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)
dd4hep::json::Collection_t json_coll_t
Handle class describing a detector element.
unsigned int flag
Flag to remember internally calculated quatities.
Helper to debug plugin manager calls.
void for_each(T oper) const
Loop processor using function object.
SensitiveDetector & setReadout(Readout readout)
Assign the IDDescriptor reference.
Segmentation segmentation() const
Access segmentation structure.
std::string missingFactory(const std::string &name) const
Helper to check factory existence.
std::string type() const
Access the type of the sensitive detector.
Class supporting the basic functionality of an JSON document including ownership.
std::map< std::string, DetElement > Children
Namespace for the AIDA detector description toolkit.
Class to easily access the properties of single JsonElements.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
Handle class supporting generic Segmentations of sensitive detectors.
dd4hep::json::Attribute json_attr_t
@ HAVE_SENSITIVE_DETECTOR
User abstraction class to manipulate JSON elements within a document.