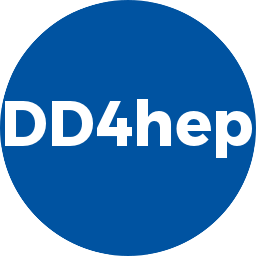 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_PLUGINS_H
14 #define DD4HEP_PLUGINS_H
24 #if __cplusplus >= 201703
26 inline bool any_has_value(std::any a){
return a.has_value(); }
28 # include <boost/any.hpp>
31 using boost::any_cast;
32 using boost::bad_any_cast;
37 #ifndef DD4HEP_PARSERS_NO_ROOT
46 template <
typename T>
class Handle;
52 template <
typename T>
static T*
ptr(
const T*
_p) {
return (T*)
_p; }
53 template <
typename T>
static T&
ref(
const T*
_p) {
return *(T*)
_p; }
54 template <
typename T>
static T
val(
const T*
_p) {
return T(*
_p); }
55 template <
typename T>
static T
value(
const void*
_p) {
return (T)
_p; }
56 static const char*
value(
const void*
_p) {
return (
const char*)(
_p); }
58 template <>
inline int PluginFactoryBase::value<int>(
const void*
_p) {
return *(
int*)(
_p); }
59 template <>
inline long PluginFactoryBase::value<long>(
const void*
_p) {
return *(
long*)(
_p); }
60 template <>
inline std::string PluginFactoryBase::value<std::string>(
const void*
_p) {
return *(std::string*)(
_p); }
61 template <>
inline const std::string& PluginFactoryBase::value<const std::string&>(
const void*
_p) {
return *(std::string*)(
_p); }
88 template <
typename R,
typename... Args>
static R
Create(
const std::string&
id, Args... args) {
89 typedef R(*func)(Args...);
92 #if DD4HEP_PLUGINSVC_VERSION==1
93 union {
void* ptr; func fcn; } fptr;
95 fptr.ptr = std::any_cast<void*>(f);
97 return (*fptr.fcn)(std::forward<Args>(args)...);
98 #elif DD4HEP_PLUGINSVC_VERSION==2
100 if ( std::any_cast<func>(f) )
101 return std::any_cast<func>(f)(std::forward<Args>(args)...);
104 catch(
const std::bad_any_cast& e) {
105 print_bad_cast(
id, f,
typeid(R(Args...)), e.what());
109 template <
typename FUNCTION>
static std::any
function(FUNCTION func) {
110 #if DD4HEP_PLUGINSVC_VERSION==1
111 union {
void* ptr; FUNCTION fcn; } fptr;
113 return std::any(fptr.ptr);
114 #elif DD4HEP_PLUGINSVC_VERSION==2
115 return std::any(func);
119 static bool setDebug(
bool new_value);
120 static stub_t
getCreator(
const std::string&
id,
const std::type_info&
info);
121 static void addFactory(
const std::string&
id,
123 const std::type_info& signature_type,
124 const std::type_info& return_type);
125 static void print_bad_cast(
const std::string&
id,
const stub_t& stub,
const std::type_info& signature,
const char* msg);
133 template <
typename R,
typename... Args>
static void add(
const std::string&
id, R(*func)(Args...)) {
134 svc_t::addFactory(
id,svc_t::function(func),
typeid(R(Args...)),
typeid(R));
141 template <
typename P,
typename S>
class Factory {};
144 #define DD4HEP_FACTORY_CALL(type,name,signature) dd4hep::PluginRegistry<signature>::add(name,Factory<type,signature>::call)
145 #define DD4HEP_IMPLEMENT_PLUGIN_REGISTRY(X,Y)
147 #define DD4HEP_OPEN_PLUGIN(ns,name) namespace ns { namespace { struct name {}; } } namespace dd4hep
148 #define DD4HEP_PLUGINSVC_CNAME(name, serial) name##_dict_##serial
149 #define DD4HEP_PLUGINSVC_FACTORY(type,name,signature,serial) \
151 struct DD4HEP_PLUGINSVC_CNAME(__typeFactory__,serial) { \
152 DD4HEP_PLUGINSVC_CNAME(__typeFactory__,serial)() { \
153 DD4HEP_FACTORY_CALL(type,#name,signature); }}; \
154 static const DD4HEP_PLUGINSVC_CNAME(__typeFactory__,serial) \
155 DD4HEP_PLUGINSVC_CNAME(s____typeFactory__,serial); \
159 #define DD4HEP_PLUGIN_FACTORY_ARGS_0(R) \
160 template <typename P> class Factory<P, R()> \
161 : public dd4hep::PluginFactoryBase { \
165 template <typename P> inline R Factory<P,R()>::call()
167 #define DD4HEP_PLUGIN_FACTORY_ARGS_1(R,A0) \
168 template <typename P> class Factory<P, R(A0)> \
169 : public dd4hep::PluginFactoryBase { \
171 static R call(A0 a0); \
173 template <typename P> inline R Factory<P,R(A0)>::call(A0 a0)
175 #define DD4HEP_PLUGIN_FACTORY_ARGS_2(R,A0,A1) \
176 template <typename P> class Factory<P, R(A0,A1)> \
177 : public dd4hep::PluginFactoryBase { \
179 static R call(A0 a0,A1 a1); \
181 template <typename P> inline R Factory<P,R(A0,A1)>::call(A0 a0, A1 a1)
183 #define DD4HEP_PLUGIN_FACTORY_ARGS_3(R,A0,A1,A2) \
184 template <typename P> class Factory<P, R(A0,A1,A2)> \
185 : public dd4hep::PluginFactoryBase { \
187 static R call(A0 a0,A1 a1,A2 a2); \
189 template <typename P> inline R Factory<P,R(A0,A1,A2)>::call(A0 a0, A1 a1, A2 a2)
191 #define DD4HEP_PLUGIN_FACTORY_ARGS_4(R,A0,A1,A2,A3) \
192 template <typename P> class Factory<P, R(A0,A1,A2,A3)> \
193 : public dd4hep::PluginFactoryBase { \
195 static R call(A0 a0,A1 a1,A2 a2, A3 a3); \
197 template <typename P> inline R Factory<P,R(A0,A1,A2,A3)>::call(A0 a0, A1 a1, A2 a2, A3 a3)
199 #define DD4HEP_PLUGIN_FACTORY_ARGS_5(R,A0,A1,A2,A3,A4) \
200 template <typename P> class Factory<P, R(A0,A1,A2,A3,A4)> \
201 : public dd4hep::PluginFactoryBase { \
203 static R call(A0 a0,A1 a1,A2 a2, A3 a3, A4 a4); \
205 template <typename P> inline R Factory<P,R(A0,A1,A2,A3,A4)>::call(A0 a0, A1 a1, A2 a2, A3 a3, A4 a4)
208 #endif // DD4HEP_PLUGINS_H
bool any_has_value(std::any a)
DD4HEP_SIGNATURE signature_t
Factory template for the plugin mechanism.
std::size_t info(const std::string &src, const std::string &msg)
PluginDebug(int dbg=2) noexcept(false)
Default constructor.
std::size_t debug(const std::string &src, const std::string &msg)
Factory template for the plugin mechanism.
static T * ptr(const T *_p)
Factory base class implementing some utilities.
Helper to debug plugin manager calls.
GAUDIPS_API void * getCreator(const std::string &id, const std::string &type)
static void add(const std::string &id, R(*func)(Args...))
static const char * value(const void *_p)
std::string missingFactory(const std::string &name) const
Helper to check factory existence.
static R Create(const std::string &id, Args... args)
Namespace for the AIDA detector description toolkit.
static T val(const T *_p)
static T value(const void *_p)
static T & ref(const T *_p)