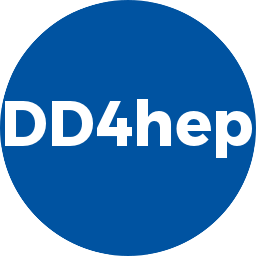 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef JSON_ELEMENTS_H
14 #define JSON_ELEMENTS_H
26 #define RAD_2_DEGREE 57.295779513082320876798154814105
29 #define M_PI 3.14159265358979323846
43 std::string
_toString(
const char *toTranscode);
47 std::string
_toString(
const std::string& s);
49 std::string
_toString(
unsigned long i,
const char* fmt =
"%lu");
51 std::string
_toString(
unsigned int i,
const char* fmt =
"%u");
53 std::string
_toString(
int i,
const char* fmt =
"%d");
55 std::string
_toString(
long i,
const char* fmt =
"%ld");
57 std::string
_toString(
float d,
const char* fmt =
"%.17e");
59 std::string
_toString(
double d,
const char* fmt =
"%.17e");
61 std::string
_ptrToString(
const void* p,
const char* fmt =
"%p");
63 template <
typename T> std::string
_toString(
const T* p,
const char* fmt =
"%p")
67 template <
typename T>
void _toDictionary(
const char* name, T value);
78 std::string
getEnviron(
const std::string& env);
81 bool _toBool(
const char* value);
83 int _toInt(
const char* value);
85 long _toLong(
const char* value);
105 typedef std::pair<JsonElement::second_type::assoc_iterator,JsonElement::second_type::assoc_iterator>
iter_t;
152 const char*
rawTag()
const;
179 bool hasAttr(
const char* t)
const;
184 return this->attr<T>(this->
attr_name(a));
187 template <
class T> T
attr(
const char* name)
const;
200 #define INLINE inline
205 template <>
INLINE const char* Handle_t::attr<const char*>(
const char* tag_value)
const {
209 template <>
INLINE bool Handle_t::attr<bool>(
const char* tag_value)
const {
213 template <>
INLINE int Handle_t::attr<int>(
const char* tag_value)
const {
217 template <>
INLINE long Handle_t::attr<long>(
const char* tag_value)
const {
221 template <>
INLINE float Handle_t::attr<float>(
const char* tag_value)
const {
225 template <>
INLINE double Handle_t::attr<double>(
const char* tag_value)
const {
229 template <>
INLINE std::string Handle_t::attr<std::string>(
const char* tag_value)
const {
276 template <
class T>
void for_each(
const char* tag_name, T oper)
const {
400 template <
class T> T
attr(
const char* tag_value)
const
425 class DocumentHandler;
434 #endif // JSON_ELEMENTS_H
const char * attr_name(const Attribute attr) const
Access attribute name (throws exception if not present)
Handle_t m_element
The underlying object holding the JsonElement pointer.
size_t size() const
Access the collection size. Avoid this call – sloooow!
bool hasChild(const char *tag_value) const
Check the existence of a child with a given tag name.
Handle_t(Elt_t e=0)
Initializing constructor.
void for_each(const char *tag_name, T oper) const
Loop processor using function object.
bool operator!() const
operator NOT: check handle validity
void operator++() const
Operator to advance the collection (pre increment)
int _toInt(const char *value)
Conversion function from raw unicode string to int.
bool _toBool(const char *value)
Conversion function from raw unicode string to bool.
Collection_t(Handle_t node, const char *tag)
Constructor over JsonElements with a given tag name.
DocumentHolder & assign(DOC d)
Assign new document. Old document is dropped.
std::string _ptrToString(const void *p, const char *fmt="%p")
Format void pointer (64 bits) to string with arbitrary format.
~NodeList()
Default destructor.
const char * attr_name(const Attribute a) const
Access attribute name (throws exception if not present)
Document(DOC d=0)
Constructor.
void throw_loop_exception(const std::exception &e) const
Helper function to throw an exception.
std::string tag() const
Text access to the element's tag.
void exception(const std::string &src, const std::string &msg)
Elt_t m_node
The pointer to the JsonElement.
T attr(const Attribute a) const
Access typed attribute value by the JsonAttr.
const char * attr_value(const Attribute attr) const
Access attribute value by the attribute (throws exception if not present)
std::string _toString(const Attribute attr)
Convert json attribute to STL string.
const JsonAttr * Attribute
NodeList & operator=(const NodeList &l)
Assignment operator.
Collection_t & reset()
Reset the collection object to restart the iteration.
std::string tag() const
Access the tag name of this element.
float _toFloat(const char *value)
Conversion function from raw unicode string to float.
T attr(const char *tag_value) const
Access attribute with implicit return type conversion.
long _toLong(const char *value)
Conversion function from raw unicode string to long.
Handle_t::Elt_t Elt_t
Simplification type declarations.
bool hasAttr(const char *name) const
Check for the existence of a named attribute.
JsonElement * next() const
Advance to next element.
NodeList children(const char *tag) const
Access a group of children identified by the same tag name.
Attribute attr_nothrow(const char *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)
JsonElement * reset()
Reset the nodelist - e.g. restart iteration from beginning.
boost::property_tree::ptree::value_type JsonElement
Element & operator=(const Element &c)
Assignment operator.
Element & operator=(Handle_t handle)
Assignment operator.
bool hasAttr(const char *t) const
Check for the existence of a named attribute.
const char * rawText() const
Unicode text access to the element's text.
Class supporting the basic functionality of an JSON document.
Attribute getAttr(const char *name) const
Access single attribute by its name.
const char * rawValue() const
Unicode text access to the element's value.
DocumentHolder()
Default Constructor.
Elt_t ptr() const
Direct access to the JsonElement by function.
NodeList m_children
Reference to the list of child nodes.
double _toDouble(const char *value)
Conversion function from raw unicode string to double.
DOC ptr() const
Accessot to DOM document behaviour.
void _toDictionary(const char *name, T value)
Helper function to populate the evaluator dictionary.
void for_each(T oper) const
Loop processor using function object.
Class describing a list of JSON nodes.
DOC operator->() const
Accessot to DOM document behaviour using arrow operator.
NodeList(const NodeList &l)
Copy constructor.
const char * tagName() const
Access the tag name of this element.
size_t numChildren(const char *tag, bool throw_exception) const
Access the number of children of this DOM element with a given tag name.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this DOM element.
std::string text() const
Access the tag name of this element.
Handle_t child(const char *tag_value, bool except=true) const
Access child by tag name. Thow an exception if required in case the child is not present.
Elt_t operator->() const
Direct access to the JsonElement using the operator->
virtual ~DocumentHolder()
Standard destructor - releases the document.
std::pair< JsonElement::second_type::assoc_iterator, JsonElement::second_type::assoc_iterator > iter_t
const char * rawTag() const
Unicode text access to the element's tag.
bool hasChild(const char *tag) const
Check the existence of a child with a given tag name.
std::string value() const
Text access to the element's value.
void dumpTree(Handle_t elt)
Handle_t root() const
Access the ROOT eleemnt of the DOM document.
void operator--() const
Operator to advance the collection (pre decrement)
Class supporting the basic functionality of an JSON document including ownership.
const char * attr_value(const Attribute a) const
Access attribute value by the attribute (throws exception if not present)
Namespace for the AIDA detector description toolkit.
Element(const Element &e)
Constructor from JsonElement handle.
Class to easily access the properties of single JsonElements.
std::string text() const
Text access to the element's text.
Class to support the access to collections of JsonNodes (or JsonElements)
size_t numChildren(const char *tag_value, bool exc=true) const
Access the number of children of this element with a given tag name.
Element(const Handle_t &e)
Constructor from JsonElement handle.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this element.
Handle_t child(const char *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
Elt_t ptr() const
Access to JsonElement pointer.
User abstraction class to manipulate JSON elements within a document.
const char * attr_value_nothrow(const char *attr) const
Access attribute value by the attribute's unicode name (no exception thrown if not present)
Elt_t current() const
Access to current element.
std::string getEnviron(const std::string &env)
Helper function to lookup environment from the expression evaluator.
DocumentHolder(DOC d)
Constructor.
boost::property_tree::ptree::value_type JsonAttr
void handle(const O *o, const C &c, F pmf)
T attr(const char *name) const
Access typed attribute value by its unicode name.
JsonElement * previous() const
Go back to previous element.
Attribute attr_ptr(const char *attr) const
Access attribute pointer by the attribute's unicode name (throws exception if not present)