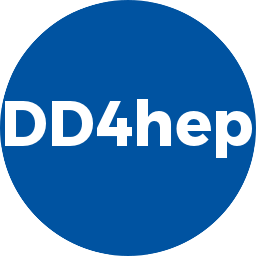 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
25 static const size_t INVALID_NODE = ~0U;
29 std::pair<int, double>
_toInteger(
const std::string& value);
31 void _toDictionary(
const std::string& name,
const std::string& value,
const std::string& typ);
36 std::string _checkEnviron(
const std::string& env) {
38 return r.empty() ? env : r;
46 const ptree::data_type& value_data(
const ptree& entry) {
54 ptree::iterator i = e->second.begin();
55 return i != e->second.end() ? &(*i) : 0;
57 ptree::assoc_iterator i = e->second.find(t);
58 return i != e->second.not_found() ? &(*i) : 0;
63 size_t node_count(
JsonElement* e,
const std::string& t) {
64 return e ? (t==
"*" ? e->second.size() : e->second.count(t)) : 0;
69 auto i = n->second.find(t);
70 return i != n->second.not_found() ? &(*i) : 0;
75 const char* attribute_value(
Attribute a) {
76 return value_data(a->second).c_str();
86 template <
typename T>
static inline std::string __to_string(T value,
const char* fmt) {
88 ::snprintf(text,
sizeof(text), fmt, value);
94 if ( !s || *s == 0 )
return "";
95 else if ( !(*s ==
'$' && *(s+1) ==
'{') )
return s;
96 return _checkEnviron(s);
101 if ( s.length() < 3 || s[0] !=
'$' )
return s;
102 else if ( !(s[0] ==
'$' && s[1] ==
'{') )
return s;
103 return _checkEnviron(s);
108 return __to_string(
v, fmt);
113 return __to_string(
v, fmt);
118 return __to_string(
v, fmt);
123 return __to_string(
v, fmt);
128 return __to_string(
v, fmt);
133 return __to_string(
v, fmt);
138 return __to_string(
v, fmt);
190 : m_tag(
copy.m_tag), m_node(
copy.m_node)
197 : m_tag(tag_value), m_node(node)
209 m_ptr = std::make_pair(
m_node->second.ordered_begin(),
m_node->second.not_found());
213 return &(*
m_ptr.first);
247 return m_node->first.c_str();
252 return value_data(
m_node->second).c_str();
257 return value_data(
m_node->second).c_str();
262 return attribute_node(
m_node, tag_value);
272 std::vector < Attribute > attrs;
274 for(ptree::iterator i=
m_node->second.begin(); i!=
m_node->second.end(); ++i) {
276 attrs.emplace_back(a);
283 size_t n = node_count(
m_node, t);
284 if (n == INVALID_NODE && !throw_exception)
286 else if (n != INVALID_NODE)
288 std::string msg =
"Handle_t::numChildren: ";
290 msg +=
"Element [" +
tag() +
"] has no children of type '" +
_toString(t) +
"'";
292 msg +=
"Element [INVALID] has no children of type '" +
_toString(t) +
"'";
293 throw std::runtime_error(msg);
299 if (e || !throw_exception)
301 std::string msg =
"Handle_t::child: ";
303 msg +=
"Element [" +
tag() +
"] has no child of type '" +
_toString(t) +
"'";
305 msg +=
"Element [INVALID]. Cannot remove child of type: '" +
_toString(t) +
"'";
306 throw std::runtime_error(msg);
314 return node_first(
m_node, tag_value) != 0;
322 std::string msg =
"Handle_t::attr_ptr: ";
324 msg +=
"Element [" +
tag() +
"] has no attribute of type '" +
_toString(t) +
"'";
326 msg +=
"Element [INVALID] has no attribute of type '" +
_toString(t) +
"'";
327 throw std::runtime_error(msg);
333 return a->first.c_str();
335 throw std::runtime_error(
"Attempt to access invalid XML attribute object!");
340 return attribute_value(
attr_ptr(attr_tag));
345 return attribute_value(attr_val);
351 return a ? attribute_value(a) : 0;
360 throw std::runtime_error(
"Document::root: Invalid handle!");
366 printout(DEBUG,
"DocumentHolder",
"+++ Release JSON document....");
383 : m_children(element, tag_value) {
389 : m_children(node_list) {
407 throw std::runtime_error(std::string(e.what()) +
"\n" +
"dd4hep: Error interpreting XML nodes of type <" +
tag() +
"/>");
409 throw std::runtime_error(std::string(e.what()) +
"\n" +
"dd4hep: Error interpreting collections XML nodes.");
446 void operator()(
const JsonElement* e,
const std::string& tag)
const {
447 std::string t = tag+
" ";
448 printout(INFO,
"DumpTree",
"+++ %s %s: %s",tag.c_str(), e->first.c_str(), e->second.data().c_str());
449 for(
auto i=e->second.begin(); i!=e->second.end(); ++i)
const char * attr_name(const Attribute attr) const
Access attribute name (throws exception if not present)
Handle_t m_element
The underlying object holding the JsonElement pointer.
size_t size() const
Access the collection size. Avoid this call – sloooow!
Handle_t(Elt_t e=0)
Initializing constructor.
void operator++() const
Operator to advance the collection (pre increment)
int _toInt(const char *value)
Conversion function from raw unicode string to int.
bool _toBool(const char *value)
Conversion function from raw unicode string to bool.
Collection_t(Handle_t node, const char *tag)
Constructor over JsonElements with a given tag name.
DocumentHolder & assign(DOC d)
Assign new document. Old document is dropped.
std::string _ptrToString(const void *p, const char *fmt="%p")
Format void pointer (64 bits) to string with arbitrary format.
~NodeList()
Default destructor.
void throw_loop_exception(const std::exception &e) const
Helper function to throw an exception.
std::string tag() const
Text access to the element's tag.
void exception(const std::string &src, const std::string &msg)
Elt_t m_node
The pointer to the JsonElement.
void _toDictionary(const std::string &name, const std::string &value)
Enter name value pair to the dictionary. "value" must be a numerical expression, which is evaluated.
const char * attr_value(const Attribute attr) const
Access attribute value by the attribute (throws exception if not present)
std::string _toString(const Attribute attr)
Convert json attribute to STL string.
const JsonAttr * Attribute
NodeList & operator=(const NodeList &l)
Assignment operator.
Collection_t & reset()
Reset the collection object to restart the iteration.
float _toFloat(const char *value)
Conversion function from raw unicode string to float.
long _toLong(const char *value)
Conversion function from raw unicode string to long.
JsonElement * next() const
Advance to next element.
NodeList children(const char *tag) const
Access a group of children identified by the same tag name.
Attribute attr_nothrow(const char *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)
JsonElement * reset()
Reset the nodelist - e.g. restart iteration from beginning.
boost::property_tree::ptree::value_type JsonElement
bool hasAttr(const char *t) const
Check for the existence of a named attribute.
const char * rawText() const
Unicode text access to the element's text.
std::pair< int, double > _toInteger(const std::string &value)
Attribute getAttr(const char *name) const
Access single attribute by its name.
const char * rawValue() const
Unicode text access to the element's value.
Elt_t ptr() const
Direct access to the JsonElement by function.
NodeList m_children
Reference to the list of child nodes.
double _toDouble(const char *value)
Conversion function from raw unicode string to double.
Namespace for the AIDA detector description toolkit supporting JSON utilities.
void _toDictionary(const char *name, T value)
Helper function to populate the evaluator dictionary.
std::pair< int, double > _toFloatingPoint(const std::string &value)
Class describing a list of JSON nodes.
std::string _getEnviron(const std::string &env)
Evaluate string constant using environment stored in the evaluator.
NodeList(const NodeList &l)
Copy constructor.
size_t numChildren(const char *tag, bool throw_exception) const
Access the number of children of this DOM element with a given tag name.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this DOM element.
virtual ~DocumentHolder()
Standard destructor - releases the document.
const char * rawTag() const
Unicode text access to the element's tag.
bool hasChild(const char *tag) const
Check the existence of a child with a given tag name.
void dumpTree(Handle_t elt)
boost::property_tree::ptree ptree
Handle_t root() const
Access the ROOT eleemnt of the DOM document.
void operator--() const
Operator to advance the collection (pre decrement)
Class supporting the basic functionality of an JSON document including ownership.
Namespace for the AIDA detector description toolkit.
Class to easily access the properties of single JsonElements.
Class to support the access to collections of JsonNodes (or JsonElements)
Handle_t child(const char *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
Elt_t ptr() const
Access to JsonElement pointer.
User abstraction class to manipulate JSON elements within a document.
const char * attr_value_nothrow(const char *attr) const
Access attribute value by the attribute's unicode name (no exception thrown if not present)
std::string getEnviron(const std::string &env)
Helper function to lookup environment from the expression evaluator.
JsonElement * previous() const
Go back to previous element.
Attribute attr_ptr(const char *attr) const
Access attribute pointer by the attribute's unicode name (throws exception if not present)